Clean code is a set of programming practices that emphasize the readability, maintainability, and simplicity of code. Writing clean code is essential because it helps developers to understand and modify code more efficiently, which can save time and reduce the risk of introducing errors.
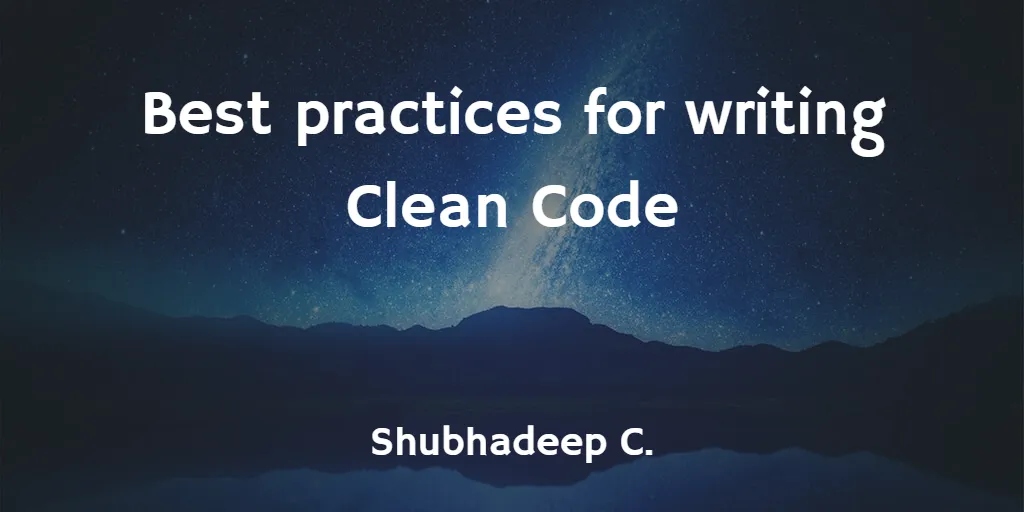
Benefits of Clean Code:
- Readability: Clean code is easy to read and understand, making it easier to maintain, debug, and update. When code is well-organized and well-structured, it is more accessible to other developers who may need to work on the same codebase.
- Maintainability: Clean code is easier to maintain over time. When code is well-organized and well-structured, it is easier to make changes or fix bugs without introducing new problems. This can save time and effort in the long run and can help prevent code from becoming obsolete.
- Scalability: Clean code is more scalable. When code is well-organized and well-structured, it can be more easily extended and modified to support new features or requirements. This can help ensure that the codebase is flexible and adaptable to changing needs.
- Collaboration: Clean code is easier to collaborate on. When code is well-organized and well-structured, it is easier for multiple developers to work on the same codebase without getting in each other’s way. This can help promote teamwork and collaboration among developers.
- Efficiency: Clean code is more efficient. When code is well-organized and well-structured, it can run faster and use less memory than poorly-organized code. This can help improve the overall performance of an application or system.
- Debugging: Clean code is easier to debug. When code is well-organized and well-structured, it is easier to identify the source of bugs or errors, making it easier to fix them. This can help reduce the time and effort required to troubleshoot and fix issues in the code.
- Reusability: Clean code is more reusable. When code is well-organized and well-structured, it can be more easily reused in other projects or parts of the same project. This can help save time and effort by avoiding the need to rewrite code from scratch.
In this article, we will discuss some of the critical principles of clean code and provide examples in C#.
1. Use meaningful variable names:
Variable names should be meaningful and descriptive of the data they contain. This makes the code easier to read and understand. For example:
// Bad Example
int x = 5;
// Good Example
int numberOfStudents = 5;
2. Use comments sparingly:
Comments should be used only when necessary and should be clear and concise. Overuse of comments can clutter the code and make it harder to read. For example:
// Bad Example
// This function adds two numbers together and returns the result
public int add(int num1, int num2)
{
// add the numbers together
int result = num1 + num2;
// return the result
return result;
}
// Good Example
public int Add(int firstNumber, int secondNumber)
{
int result = firstNumber + secondNumber;
return result;
}
3. Use consistent formatting:
Consistent formatting makes the code easier to read and understand. This includes consistent indentation, spacing, and braces. For example:
// Bad Example
public void PrintName(string name)
{
Console.WriteLine("Name: " + name);
}
// Good Example
public void PrintName(string name)
{
Console.WriteLine($"Name: {name}");
}
4. Write small, focused methods:
Methods should be small and focused, with a single responsibility. This makes the code easier to understand and modify. For example:
// Bad Example
public void DoEverything(int x, int y, int z)
{
// do something
// do something else
// and more
}
// Good Example
public int Add(int firstNumber, int secondNumber)
{
return firstNumber + secondNumber;
}
public int Multiply(int firstNumber, int secondNumber)
{
return firstNumber * secondNumber;
}
5. Use meaningful method names:
Method names should be meaningful and descriptive of what the method does. This makes the code easier to read and understand. For example:
// Bad Example
public void DoSomething(int x, int y, int z)
{
// do something
}
// Good Example
public void CalculateTotalCost(decimal price, int quantity)
{
// calculate total cost
}
6. Use meaningful class names:
Class names should be meaningful and describe what the class does. This makes the code easier to read and understand. For example:
// Bad Example
public class MyClass1
{
// code here
}
// Good Example
public class Student
{
// code here
}
7. Use inheritance and polymorphism:
Inheritance and polymorphism can make the code more flexible and easier to modify. For example:
// Bad Example
public class Animal
{
public void Walk()
{
// walk
}
}
public class Dog
{
public void Walk()
{
// walk
}
public void Bark()
{
// bark
}
}
// Good Example
public abstract class Animal
{
public abstract void Walk();
}
public class Dog : Animal
{
public override void Walk()
{
// walk
}
public void Bark()
{
// bark
}
}
8. Use exception handling:
Exception handling is important to handle errors and prevent unexpected behavior of the code. It is important to handle exceptions in a consistent and meaningful way. For example:
// Bad Example
public void Divide(int num1, int num2)
{
int result = num1 / num2;
}
// Good Example
public void Divide(int num1, int num2)
{
try
{
int result = num1 / num2;
}
catch (DivideByZeroException ex)
{
Console.WriteLine("Cannot divide by zero");
// log the error
}
}
9. Use descriptive error messages:
Error messages should be clear and descriptive, to help developers understand the problem and how to fix it. For example:
// Bad Example
public void CalculateTotal(decimal price, int quantity)
{
if (price < 0 || quantity < 0)
{
throw new Exception("Invalid input");
}
// calculate total
}
// Good Example
public void CalculateTotal(decimal price, int quantity)
{
if (price < 0)
{
throw new ArgumentException("Price cannot be negative", nameof(price));
}
if (quantity < 0)
{
throw new ArgumentException("Quantity cannot be negative", nameof(quantity));
}
// calculate total
}
10. Use unit testing:
Unit testing is an important practice to ensure that the code works as expected and that changes to the code do not introduce errors. Writing unit tests can also help to ensure that the code is clean and well-designed. For example:
// Bad Example
public int Add(int firstNumber, int secondNumber)
{
return firstNumber + secondNumber;
}
// Good Example
public int Add(int firstNumber, int secondNumber)
{
if (firstNumber < 0 || secondNumber < 0)
{
throw new ArgumentException("Both numbers must be positive");
}
return firstNumber + secondNumber;
}
[TestFixture]
public class MathTests
{
[Test]
public void Add_PositiveNumbers_ReturnsCorrectResult()
{
Math math = new Math();
int result = math.Add(2, 3);
Assert.AreEqual(5, result);
}
[Test]
public void Add_NegativeNumber_ThrowsArgumentException()
{
Math math = new Math();
Assert.Throws<ArgumentException>(() => math.Add(-1, 2));
}
}
11. Use comments judiciously:
While it is important to write code that is self-documenting, sometimes comments are necessary to provide additional context or explain complex logic. However, comments should not be used as a substitute for clean code and should be used judiciously. For example:
// Bad Example
public void CalculatePrice(decimal price, int quantity)
{
// calculate total
decimal total = price * quantity;
// add tax
decimal tax = total * 0.05M;
// add shipping
decimal shipping = 10;
// calculate final price
decimal finalPrice = total + tax + shipping;
Console.WriteLine(finalPrice);
}
// Good Example
public void CalculatePrice(decimal price, int quantity)
{
decimal total = price * quantity;
decimal tax = total * 0.05M; // tax rate is 5%
decimal shipping = 10;
decimal finalPrice = total + tax + shipping;
Console.WriteLine(finalPrice);
}
12. Avoid duplication:
Code duplication can make the code harder to understand, maintain, and modify. It is important to identify and remove duplication by using methods, classes, and inheritance. For example:
// Bad Example
public void GenerateInvoice(string customerName, List<Product> products)
{
Console.WriteLine("Invoice for " + customerName);
Console.WriteLine("Product\t\tPrice");
decimal total = 0;
foreach (Product product in products)
{
Console.WriteLine(product.Name + "\t\t" + product.Price);
total += product.Price;
}
Console.WriteLine("Total\t\t" + total);
}
// Good Example
public class Invoice
{
public string CustomerName { get; set; }
public List<Product> Products { get; set; }
public decimal GetTotal()
{
decimal total = 0;
foreach (Product product in Products)
{
total += product.Price;
}
return total;
}
}
public class InvoiceGenerator
{
public void GenerateInvoice(Invoice invoice)
{
Console.WriteLine("Invoice for " + invoice.CustomerName);
Console.WriteLine("Product\t\tPrice");
foreach (Product product in invoice.Products)
{
Console.WriteLine(product.Name + "\t\t" + product.Price);
}
Console.WriteLine("Total\t\t" + invoice.GetTotal());
}
}
13. Keep methods small:
Small methods are easier to understand, test, and modify. It is important to break down larger methods into smaller, focused methods that do one thing and do it well. For example:
// Bad Example
public void SaveData(string data)
{
if (data == null)
{
throw new ArgumentNullException(nameof(data));
}
if (data.Length > 100)
{
throw new ArgumentException("Data length cannot exceed 100 characters", nameof(data));
}
if (File.Exists("data.txt"))
{
File.Delete("data.txt");
}
File.WriteAllText("data.txt", data);
}
// Good Example
public void SaveData(string data)
{
ValidateData(data);
DeleteExistingFile("data.txt");
WriteFile("data.txt", data);
}
private void ValidateData(string data)
{
if (data == null)
{
throw new ArgumentNullException(nameof(data));
}
if (data.Length > 100)
{
throw new ArgumentException("Data length cannot exceed 100 characters", nameof(data));
}
}
private void DeleteExistingFile(string fileName)
{
if (File.Exists(fileName))
{
File.Delete(fileName);
}
}
private void WriteFile(string fileName, string data)
{
File.WriteAllText(fileName, data);
}
Conclusion
In conclusion, writing clean code is an important practice that can save time, reduce the risk of errors, and make code easier to understand and modify. By using meaningful variable and method names, consistent formatting, small and focused methods, inheritance and polymorphism, and exception handling, developers can write code that is more readable, maintainable, and simple. Using descriptive error messages and unit testing can also help to ensure that the code works as expected and is clean and well-designed. These practices can be applied to C# code, as well as other programming languages.