Scheduling one or more background tasks is almost inevitable when building robust, self-sustaining APIs with .NET. A few packages have been around for years, such as Hangfire and Quartz.NET.
ASP.NET Core allows background tasks to be implemented as hosted services. However, you might need something more customizable and lightweight with a simplistic syntax. I present Coravel.
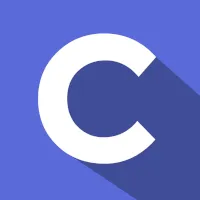
Coravel
Coravel is an open-source, lightweight library for .NET that allows you to easily perform background processing and scheduling in your ASP.NET Core application. Coravel helps developers get their .NET applications up and running fast by making advanced application features like task/job scheduling, queuing, caching, mailing (and more!) accessible and easy to use.
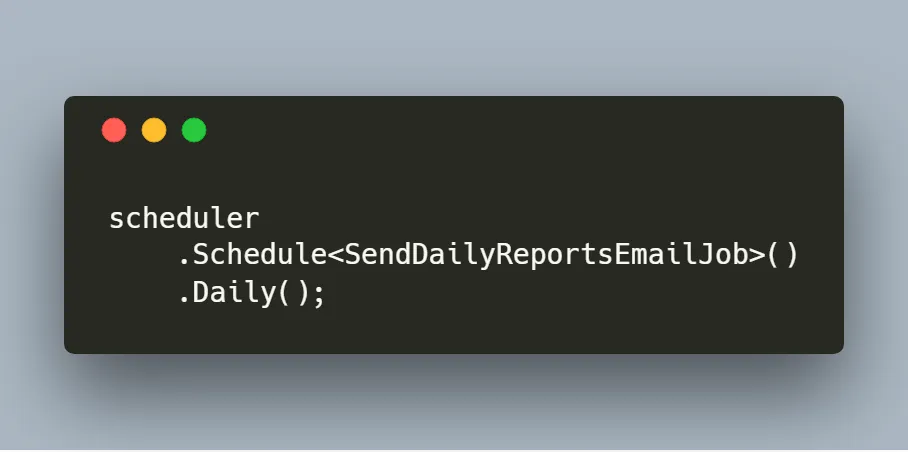
Scheduling syntax
Coravel is inspired by Laravel’s task scheduler, and it’s built on top of the .NET Core built-in dependency injection. It uses a fluent API to schedule tasks, allowing you to easily specify the frequency, start time, and end time of the task. It also provides a simple way to queue and process background jobs, allowing you to easily process large amounts of data or perform long-running tasks.
Why do I use Coravel for scheduling?
- It is lightweight compared to alternatives such as Hangfire and Quartz.net.
- It is Near-zero config and gets the job done with minimal lines of code.
- It is is easy to use with a simplistic syntax
- It supports async/await and is super efficient.
Coravel Pro
Coravel Pro is a professional admin panel with visual tools that seamlessly integrate into your .NET Core apps.
Features include:
- Database persisted scheduling
- A beautiful UI to manage your jobs/invocables
- Health metrics dashboard
- Easily configured tabular reports of your data (supports aggregation/complex projections)
If you love Coravel, are building a .NET Core app with EF Core, and want even more awesome tools — check it out!
Requirements
Coravel is a .NET Standard library designed for .NET Core apps. Include Coravel in either an existing .NET Core application (version 2.1.0+) or another .NET Standard project(s).
Installation
Coravel requires the nuget package Coravel
to get started.
dotnet add package coravel
Task/Job scheduling
Usually, you have to configure a cron job or a task via Windows Task Scheduler to get a single or multiple re-occurring tasks to run.
With Coravel, you can setup all your scheduled tasks in one place using a simple, elegant, fluent syntax — in code!
Invocables represent a self-contained job within your application. Creating an invocable uses the shared interface Coravel.Invocable.IInvocable
. Using .NET Core’s dependency injection services, your invocables will have all their dependencies injected whenever they are executed.
Below is a sample code for creating an invocable (Job):
public class ExpiryJobInvocable : IInvocable
{
private readonly ICatalogueService _catalogueService;
private readonly ILogger<ExpiryJobInvocable> _logger;
public ExpiryJobInvocable(ICatalogueService catalogueService, ILogger<ExpiryJobInvocable> logger)
{
_logger = logger;
_catalogueService = catalogueService;
}
public async Task Invoke()
{
await _catalogueService.ExpireCatalogueListing();
}
}
Registering invocables with dependency injection looks like this:
public static IServiceCollection RegisterCoravelScheduler(this IServiceCollection services)
{
services.AddScheduler();
services.AddTransient<ExpiryJobInvocable>();
return services;
}
Also, do not forget to register in the application builder by specifying the frequency. It exposes preconfigured methods, which include cron expression syntax.
var app = builder.Build();
app.Services.UseScheduler(scheduler =>
{
scheduler.Schedule<ExpiryJobInvocable>().EveryMinute().PreventOverlapping(nameof(ExpiryJobInvocable));
}).LogScheduledTaskProgress(app.Services.GetRequiredService<ILogger<IScheduler>>());
Here are the methods available for specifying frequency:
//
// Summary:
// Defines methods available to you for specifying the frequency of how often your
// scheduled tasks will run.
public interface IScheduleInterval
{
IScheduledEventConfiguration EveryMinute();
IScheduledEventConfiguration EveryFiveMinutes();
IScheduledEventConfiguration EveryTenMinutes();
IScheduledEventConfiguration EveryFifteenMinutes();
IScheduledEventConfiguration EveryThirtyMinutes();
IScheduledEventConfiguration Hourly();
IScheduledEventConfiguration HourlyAt(int minute);
IScheduledEventConfiguration Daily();
IScheduledEventConfiguration DailyAtHour(int hour);
IScheduledEventConfiguration DailyAt(int hour, int minute);
IScheduledEventConfiguration Weekly();
IScheduledEventConfiguration Monthly();
IScheduledEventConfiguration Cron(string cronExpression);
IScheduledEventConfiguration EverySecond();
IScheduledEventConfiguration EveryFiveSeconds();
IScheduledEventConfiguration EveryTenSeconds();
IScheduledEventConfiguration EveryFifteenSeconds();
IScheduledEventConfiguration EveryThirtySeconds();
IScheduledEventConfiguration EverySeconds(int seconds);
}
Coravel supports other functions aside from Task Scheduling. I summarized a few of them, and feel free to utilize them.
Queuing
Coravel gives you a zero-configuration queue that runs in-memory. This is useful to offload long-winded tasks to the background instead of making your users wait for their HTTP request to finish.
For more information about utilizing Coravel’s Queuing functionality, check out the documentation page.
Caching
Coravel provides you with an easy-to-use API for caching in your .NET Core applications. By default, it uses an in-memory cache. Coravel also provides some database drivers for more robust scenarios.
For more information about utilizing Coravel’s Caching functionality, check out the documentation page.
Event Broadcasting
Coravel’s event broadcasting allows listeners to subscribe to events that occur in your application. This is a great way to build maintainable applications whose parts are loosely coupled!
For more information about utilizing Coravel’s Events functionality, check out the documentation page.
Mailing
E-mails are not as easy as they should be. Luckily for you, Coravel solves this by offering:
- Built-in e-mail friendly razor templates
- Simple and flexible mailing API
- Render your e-mails for visual testing via ASP Controllers, etc.
- Drivers supporting SMTP, e-mailing to a local log file or BYOM (“bring your own mailer”) driver
- Quick and simple configuration via appsettings.json
Coravel uses Mailables to send mail. Each Mailable is a C# class that represents a specific type of e-mail that you can send, such as “New User Sign-up”, “Completed Order”, etc.
For more information about utilizing Coravel’s mailing functionality, check out the documentation page.