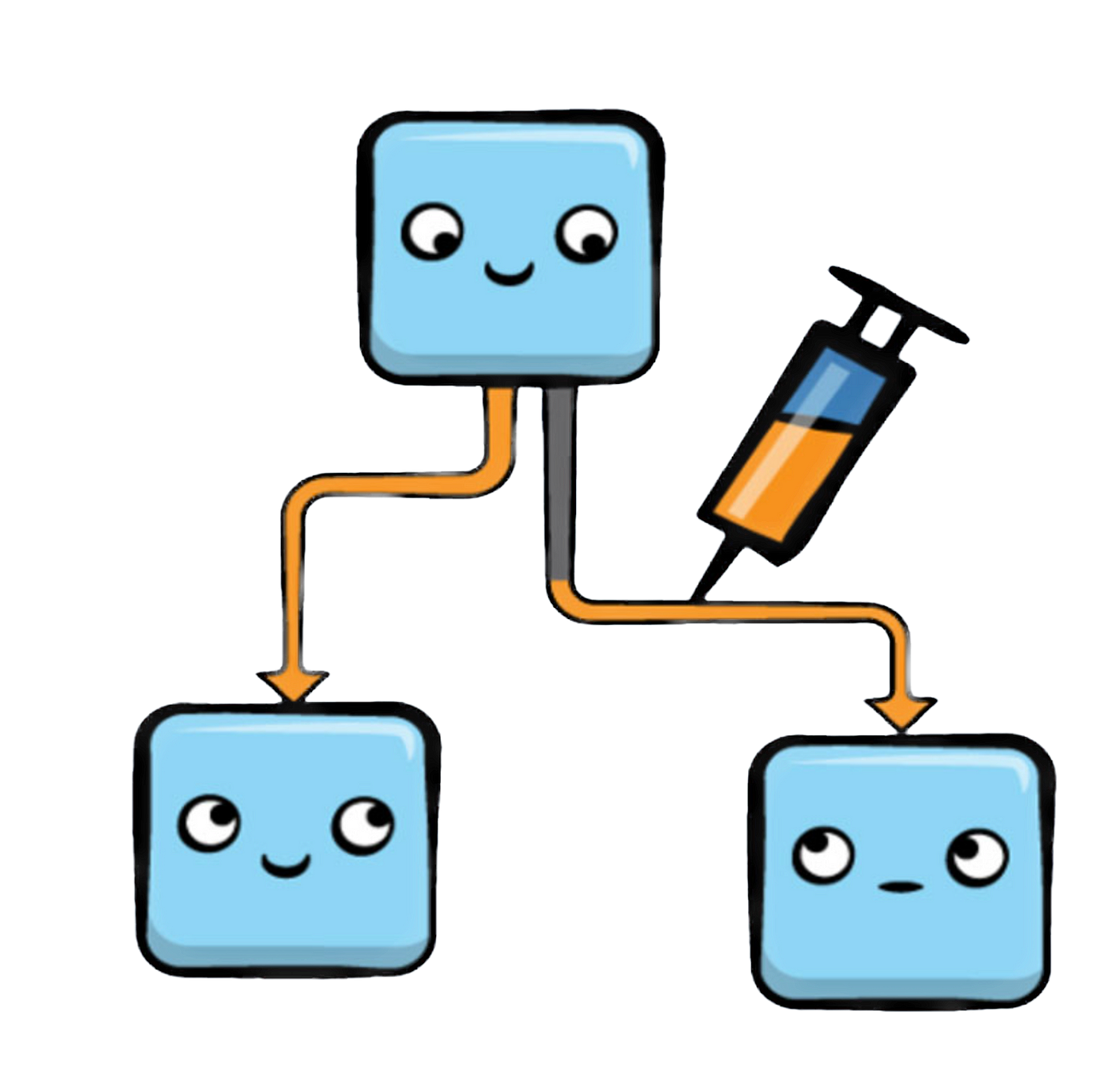
When crafting elegant and scalable software in C#, a keen understanding of Dependency Injection (DI) is more than a luxury — it’s a necessity. It’s a design pattern that underpins many modern .NET applications, providing a solid foundation for managing dependencies between classes. In its most basic form, Dependency Injection promotes loose coupling, simplifying the maintenance and testing of applications.
This article will explore the best practices for Dependency Injection in C#, shedding light on common pitfalls and demonstrating how to harness the full power of this vital technique. As we journey through this in-depth examination, we’ll unpack the history and importance of Dependency Injection, delve into its implementation, and scrutinize real-world applications.
By the end of this article, you’ll be equipped to integrate Dependency Injection into your C# applications effectively, optimizing code quality and enhancing scalability. Whether you’re a seasoned C# veteran seeking a fresh perspective or a novice programmer looking to deepen your understanding, this comprehensive guide will serve as an invaluable resource in your coding toolkit.
Background
To fully appreciate Dependency Injection in C# and understand its potential, we need to delve into its historical context and explore the essence of its necessity in modern software development.
A Brief History of Dependency Injection
The roots of Dependency Injection stretch back to the advent of object-oriented programming, a paradigm that favors the creation of cohesive and loosely coupled modules. However, the official term “Dependency Injection” wasn’t coined until the early 2000s when Martin Fowler, a British software engineer, gave this powerful technique a name. Fowler highlighted Dependency Injection as a form of Inversion of Control (IoC), a broader principle that shifts the control of objects or portions of a program to a container or a framework.
Since Fowler’s introduction, Dependency Injection has gained significant traction across programming languages, but it has shown particular effectiveness within the .NET framework, more specifically in C#. Its wide adoption can be attributed to the development of various DI frameworks and libraries that simplify the implementation of this pattern.
Why Dependency Injection is Necessary in C#
While C# is a flexible and powerful language, like any tool, its power can be both an asset and a liability. Without proper management, dependencies between classes can result in tightly-coupled code, making it difficult to modify, scale, and test applications.
Enter Dependency Injection. This pattern seeks to mitigate these issues, contributing to more maintainable and scalable C# code. It provides a systematic way to assemble classes from loosely-coupled components, ultimately improving the reusability and testability of the code.
Moreover, with the advent of .NET Core and the built-in support for Dependency Injection, the technique has become even more critical in creating robust and efficient applications.
Having touched on the historical and practical reasons for Dependency Injection’s relevance, let’s move on to understanding this pattern’s core concepts and how they’re implemented in C#.
Understanding Dependency Injection
Before we delve into the intricacies of employing Dependency Injection in C#, we need to understand its core concepts and its relationship with the broader principle of Inversion of Control.
Core Concepts of Dependency Injection
The concept of Dependency Injection revolves around the idea of “dependencies.” In the context of programming, a dependency is when one object relies on another to perform its function. Traditionally, an object would create or find its dependencies internally, but this leads to a tightly-coupled design that’s hard to manage and test.
Dependency Injection addresses this by having dependencies provided to the object (or “injected”), typically through the object’s constructor, a method, or a property. This way, the object isn’t responsible for finding or creating its dependencies, leading to a more modular and flexible design.
The three primary types of Dependency Injection are:
1. Constructor Injection: The dependencies are provided through a class constructor. This is the most commonly used and the most recommended form of dependency injection.
public interface ILogger
{
void Log(string message);
}
public class ConsoleLogger : ILogger
{
public void Log(string message)
{
Console.WriteLine(message);
}
}
public class MyClass
{
private ILogger _logger;
// Constructor
public MyClass(ILogger logger)
{
_logger = logger;
}
public void DoSomething()
{
_logger.Log(“We did something!”);
}
}
In this example, MyClass
is dependent on ILogger. The ILogger
dependency is injected via the constructor and can be easily replaced with any class that implements the ILogger
interface.
2. Setter Injection: The client exposes a setter method that the injector uses to inject the dependency.
public class MyClass
{
private ILogger _logger;
// Property
public ILogger Logger
{
set { _logger = value; }
}
public void DoSomething()
{
_logger.Log(“We did something!”);
}
}
In this example, the ILogger
dependency is injected via the Logger
property.
3. Interface Injection: The dependency provides an injector method that will inject the dependency.
Interface injection requires the dependent class to implement an interface that will be used to provide the dependency.
Here’s an example:
public interface ILogger
{
void Log(string message);
}
public interface ILoggerSetter
{
void SetLogger(ILogger logger);
}
public class MyClass : ILoggerSetter
{
private ILogger _logger;
public void SetLogger(ILogger logger)
{
_logger = logger;
}
public void DoSomething()
{
_logger.Log(“We did something!”);
}
}
In this example, MyClass
implements the ILoggerSetter
interface to allow ILogger dependency injection.
Each of these types has its uses, which we will explore further in the implementation section.
Dependency Injection and Inversion of Control
Inversion of Control (IoC) is a broader design principle that Dependency Injection falls under. It involves inverting the flow of control in a system, meaning that the framework or container calls the custom, user-written code, rather than the other way around.
In the context of Dependency Injection, IoC means inverting the control of managing dependencies. Instead of each object controlling its dependencies, this responsibility is given to an external entity (an IoC container). This external entity creates and wires up dependencies where they are needed.
By adhering to the principle of IoC, Dependency Injection allows for a much cleaner and modular codebase, making it easier to manage complexity, particularly in large-scale applications.
With a basic understanding of Dependency Injection and its relationship with Inversion of Control in place, we can proceed to explore the actual implementation of Dependency Injection in C# programming.
Implementing Dependency Injection in C#
Now that we’ve grasped the core concepts of Dependency Injection and seen them in action through illustrative C# code snippets, it’s time to dive into the implementation process. This includes understanding standard practices and exploring Dependency Injection Containers in C#, which aid in managing dependencies.
Standard Practices
The implementation of Dependency Injection in C# aligns with several standard practices. The examples previously given demonstrated how dependencies are injected through constructors, setters, or interfaces. However, there are further aspects to consider:
- High-level modules should not depend on low-level modules: Both should depend on abstractions. This principle, one of the SOLID principles for object-oriented programming, encourages us to design systems in a way that reduces the dependencies between modules.
- Abstraction should not depend on details: Details should depend on abstractions. This principle suggests that the overall strategy of a system should dictate the low-level tactics, not the other way around.
Suppose we have a NotificationService
class which is a high-level module in our application. This class depends on a EmailService
class which is a low-level module for sending notifications.
// Low-Level Module
public class EmailService
{
public void SendEmail(string email, string message)
{
// Code to send email
}
}
// High-Level Module
public class NotificationService
{
private EmailService _emailService;
public NotificationService()
{
_emailService = new EmailService();
}
public void Notify(string email, string message)
{
_emailService.SendEmail(email, message);
}
}
In the above code, NotificationService
is tightly coupled with EmailService
. This isn't an ideal situation, because if we decide to change our notification method from email to something else like SMS or push notifications, NotificationService
would have to change too.
We can solve this by depending on an abstraction rather than depending directly on EmailService
. Let's define an interface INotificationService
, and let the EmailService
implement this interface.
// Abstraction
public interface INotificationService
{
void Notify(string contact, string message);
}
// Low-Level Module
public class EmailService : INotificationService
{
public void Notify(string email, string message)
{
// Code to send email
}
}
// High-Level Module
public class NotificationService
{
private INotificationService _notificationService;
public NotificationService(INotificationService notificationService)
{
_notificationService = notificationService;
}
public void Notify(string contact, string message)
{
_notificationService.Notify(contact, message);
}
}
Now, NotificationService
depends on the abstraction INotificationService
, not on the low-level module EmailService
. If we need to change our notification method, we just need to create a new class implementing INotificationService
, for example, SMSService
, and inject it into NotificationService
. The NotificationService
class itself doesn't need to change, which adheres to the Open/Closed Principle—another SOLID principle. This decouples the high-level module from the low-level module and makes the system more modular and flexible.
Adhering to these principles results in an application structure that is modular, scalable, and easy to understand and maintain.
Dependency Injection Containers in C#
A Dependency Injection Container, also known as an IoC (Inversion of Control) Container, is a framework for implementing automatic dependency injection. It manages object creation and injects dependencies when required, making it easier to implement Dependency Injection in a consistent manner throughout an application.
.NET Core has built-in support for Dependency Injection and comes with its own lightweight IoC container. However, if you need more features, there are other more powerful containers available like Autofac, Ninject, and Unity.
Here’s an example of how to use the built-in IoC container in .NET Core:
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<ILogger, ConsoleLogger>();
}
}
In this example, the Startup
class has a ConfigureServices
method. This is where you configure the application's services. In the method, a ConsoleLogger
is registered as a service that can fulfil the ILogger
dependency whenever it's required. The AddTransient
method specifies that a new ConsoleLogger
instance should be created each time the ILogger
service is requested.
With a fundamental understanding of implementing Dependency Injection in C# under our belt, we’re ready to explore the best practices to follow when incorporating this design pattern into your applications.
Best Practices
Understanding the mechanism of Dependency Injection and its implementation in C# is half the battle. Equally crucial is understanding the best practices that ensure its benefits are fully harnessed. These practices not only improve code maintainability and readability, but they also enhance the flexibility and scalability of your applications.
1. Favor Constructor Injection
While there are various types of Dependency Injection, Constructor Injection is generally preferred. By using the constructor to inject dependencies, you guarantee the class has all it needs to be fully operational. This promotes immutability and enhances thread-safety, as the dependencies can’t be changed once they’re set.
2. Avoid Using Service Locator
Service Locator is often considered an anti-pattern as it hides class dependencies, making code harder to understand and maintain. It can also make code harder to test because it couples your code to specific dependencies, making it less flexible. While there can be use cases where a Service Locator may seem beneficial, using Dependency Injection will generally result in more maintainable and testable code.
3. Use Abstractions, Not Concretions
A key principle of Dependency Injection is to “depend upon abstractions, not concretions.” This means that your classes should depend on interfaces or abstract classes, not on concrete classes. This makes the system more modular and flexible, as you can easily swap one implementation for another.
4. Be Mindful of Lifetimes
In C#, different Dependency Injection lifetimes such as Transient, Scoped, and Singleton can be specified. Understanding their differences is critical. For example, a Singleton service lives for the duration of the application and all consumers share the same instance. A Transient service, on the other hand, is created each time it’s requested. Choosing the wrong lifetime can lead to issues like unintended sharing of state between requests.
5. Keep Constructors Lean
The constructor should be used to assign dependencies to fields or properties, but nothing more. Heavy computation or logic that could throw exceptions should be avoided in constructors. The reason for this is that if a service’s instantiation fails, it might cause the entire Dependency Injection framework to fail, which can be hard to debug.
6. Don’t Try to Resolve Everything with Dependency Injection
Dependency Injection is a tool, not a solution to every problem. There are cases where it’s not necessary and could even over-complicate things. If a class is not a shared dependency or doesn’t need to be substituted for testing or different implementations, it might not need to be injected.
Adhering to these best practices while implementing Dependency Injection in C# can lead to more robust, testable, and maintainable code. It’s an essential tool in a developer’s toolkit, making software development a more manageable and enjoyable task.
Addressing Common Problems
As potent as Dependency Injection is, it’s not a silver bullet. Like any approach, it has its share of problems that developers should be aware of. Here, we will address a few common challenges associated with Dependency Injection in C# and provide recommendations to overcome them.
1. Overcomplication
One of the most common pitfalls developers fall into is overusing Dependency Injection, which can lead to an overcomplicated system. This usually happens when developers try to apply Dependency Injection to classes that don’t have dependencies or don’t need to be interchangeable.
Solution: Remember, Dependency Injection is a tool and should only be used when it solves a problem. If a class doesn’t have dependencies or doesn’t need to have different implementations, it might be best not to inject it.
2. Mismanagement of Object Lifetimes
Misunderstanding or incorrectly managing Dependency Injection lifetimes can lead to severe issues such as state bleeding between requests or memory leaks.
Solution: Take the time to understand the differences between Transient, Scoped, and Singleton lifetimes, and choose the appropriate one based on the needs of your application.
3. Troubles with Deep Class Hierarchies
When working with deep class hierarchies, managing dependencies can become problematic. It’s common to see constructors bloated with dependencies, which can make the code harder to read and maintain.
Solution: If a class starts to have too many dependencies, it might be a sign that the class is doing too much and violating the Single Responsibility Principle. Consider refactoring the class and splitting its responsibilities among several smaller, more focused classes.
4. Difficulties with Unit Testing
While Dependency Injection is a boon for unit testing, it can cause difficulties if not implemented correctly. Dependencies that aren’t properly abstracted can make it hard to replace them with mock implementations for testing.
Solution: Always depend on abstractions, not on concrete implementations. Make sure to abstract dependencies using interfaces or abstract classes, which allows for easy substitution with mock implementations during testing.
5. Increased Startup Time
The use of Dependency Injection can increase the startup time of an application as the container needs to resolve all the dependencies at the start.
Solution: To minimize the impact on startup time, keep your constructors lean, and avoid any heavy computation or I/O operations in the constructors. All the heavy operations should be deferred until they are really needed.
By being aware of these common challenges and knowing how to address them, you can ensure that Dependency Injection serves as a net positive for your C# applications.
Innovative Ideas and Trends in Dependency Injection
Dependency Injection (DI) has been a staple in software design for a while, but as with any technology or approach, it continues to evolve. Let’s explore some of the innovative ideas and trends influencing the use of Dependency Injection in C# and .NET:
1. Containerless DI
Containerless Dependency Injection, also known as “Pure DI”, refers to using the principles of Dependency Injection without a DI container. In this approach, dependencies are wired up manually in the “composition root” of the application.
This method tends to be simpler, more explicit, and can lead to better design as it requires the developer to pay more attention to the dependency graph of an application. However, it can also be more tedious and error-prone, particularly for larger applications.
2. Auto-Registration
One of the recent trends is the auto-registration of dependencies, where the DI container automatically scans assemblies and registers services and their implementations. This can greatly reduce the amount of boilerplate code and make the process of adding new services much smoother.
However, it’s important to use this feature judiciously as it can make the code less explicit, potentially hiding errors until runtime.
3. Integrating DI with Functional Programming
As functional programming paradigms are increasingly adopted in C#, some developers are exploring ways to integrate Dependency Injection with functional programming concepts. This can involve using monads, partial application, and other functional constructs to manage dependencies.
4. Using DI for Microservices Architecture
As more and more applications move to microservices architectures, Dependency Injection is playing a crucial role in managing the complexity of these systems. By helping to keep services decoupled and modular, DI can make it easier to develop, test, and maintain microservices.
5. Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming involves separating cross-cutting concerns from the main business logic of the application. With DI, AOP can be implemented more easily, allowing developers to add behavior like logging, caching, and transaction management to their applications in a modular way.
Conclusion
Navigating the world of Dependency Injection in C# is a journey that can significantly elevate your software design, promoting modularity, testability, and maintainability. It’s an approach that aligns with the principles of modern software development, advocating for loosely coupled and highly cohesive systems.
We’ve delved into the concept of Dependency Injection, its types, and how to implement it in C#. We’ve explored the best practices and addressed common problems that arise while working with Dependency Injection. Finally, we’ve touched upon the innovative ideas and trends shaping the landscape of Dependency Injection.
It’s worth noting that while Dependency Injection provides numerous benefits, it isn’t without its challenges. Overuse can lead to overcomplication, and improper management of object lifetimes can lead to bugs. It’s crucial to understand these potential issues and follow the best practices to mitigate them.
As you continue to hone your skills in C#, remember to keep the principles of Dependency Injection in mind. They will not only help you create better software but also guide you to be a better developer. Keep exploring, keep learning, and remember: technology is constantly evolving, and so should we.
Good luck, and happy coding!