Unleashing the Power of Meta-Programming: A Comprehensive Guide to C# Reflection
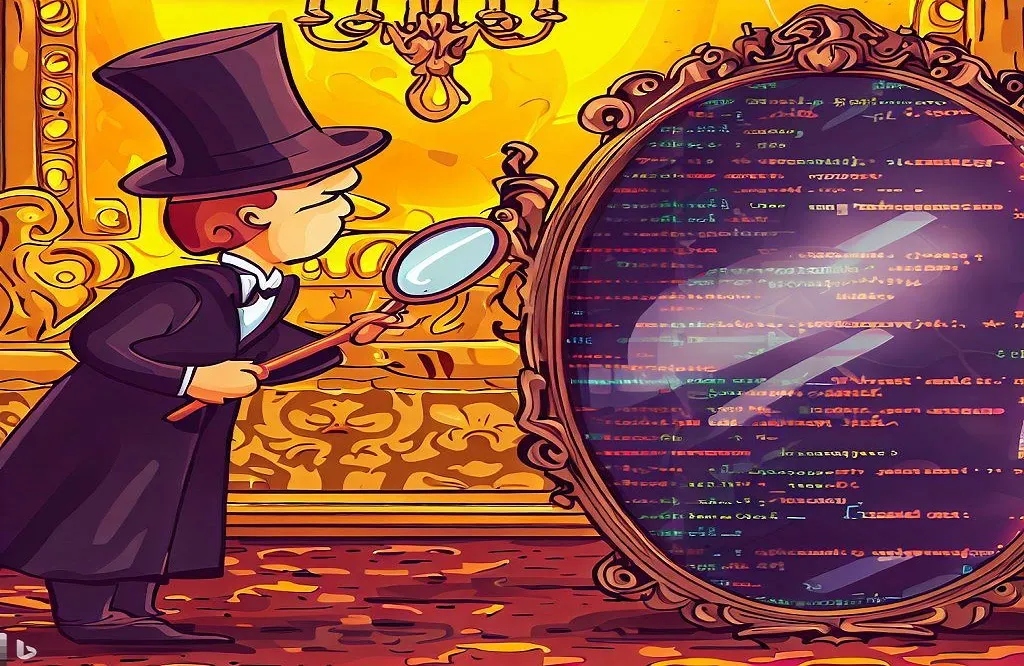
Reflection, put simply, is a mechanism provided by the .NET framework that allows a running program to examine and manipulate itself. It’s like a coding mirror that gives your application the ability to look at its own structure, inspect its assemblies, types, and members, and even modify them-all at runtime.
The concept might seem abstract and complicated at first, but once you understand its power, it opens the doors to some exciting possibilities. It brings an added dimension of flexibility and dynamism to your code, allowing you to interact with it in new and fascinating ways.
Picture this scenario: you have a class, and you know nothing about it-what methods it has, what properties it possesses, or what constructors it offers. With Reflection, you can investigate this class, discover its properties and methods, and call them, even if they are private! The class can be a sealed box, yet Reflection grants you an X-ray vision to see inside it.
But, it’s not just about investigating. Reflection in C# also lets you modify your code at runtime. Imagine being able to create new instances of a class without knowing its type at compile time, or changing the values of private fields, or even generating entirely new methods on the fly!
Meta-Programming, a term that sounds like something straight out of a sci-fi novel, is as powerful as it sounds. It is a practice that elevates your programming skills to a meta level, allowing your code to read, generate, analyze, or transform other code. In other words, you’re programming your programs to program!
But why does meta-programming matter, and why should you care about it?
The beauty of meta-programming and, by extension, Reflection, is the ability to write more dynamic, flexible, and adaptable code. It allows you to do things like reading metadata about types, creating and manipulating objects, or invoking methods, all at runtime.
This dynamism has broad implications. It can lead to cleaner, more modular, and scalable codebases, which can adapt based on configuration files or user inputs. It can open up possibilities for plugin architectures, where third-party code can be integrated and interacted with safely and efficiently. And it can enable powerful serialization mechanisms, which is useful for saving and loading data in games, web applications, and more.
Also note that the GetTypes
method will throw an exception if any of the classes in the module cannot be loaded. If you want to avoid this, you can use the GetExportedTypes
method instead, which only returns public types
Retrieving a Type’s Assembly
You can use Reflection to retrieve the assembly a type belongs to.
Type type = typeof(MyClass); // Replace with your type
Assembly assembly = type.Assembly;
Console.WriteLine($"Type {type.Name} is defined in assembly {assembly.FullName}");
Retrieving All Loaded Assemblies
Reflection allows you to retrieve all assemblies currently loaded in the application domain.
// Get all currently loaded assemblies in the current application domain
Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies();
foreach (Assembly assembly in assemblies)
{
Console.WriteLine(assembly.FullName);
}
Inspecting Assembly Information
Reflection allows you to inspect various information about an assembly, like its version, culture, public key, and more.
// Load an assembly.
Assembly assembly = Assembly.LoadFrom(@"C:\path\to\your\assembly.dll");
// Print the assembly information.
Console.WriteLine("Full Name: " + assembly.FullName);
Console.WriteLine("Location: " + assembly.Location);
Console.WriteLine("CodeBase: " + assembly.CodeBase);
Console.WriteLine("EntryPoint: " + assembly.EntryPoint);
// Print the version information.
Version version = assembly.GetName().Version;
Console.WriteLine("Version: " + version);
// Print all the types in the assembly.
foreach (Type type in assembly.GetTypes())
{
Console.WriteLine("Type: " + type.FullName);
}
Getting All Types in an Assembly
You can get all types in an assembly, which can be useful for various purposes like building your own type browser, creating IoC containers, or plugin systems.
Assembly assembly = Assembly.Load("YourAssemblyName"); // Replace with your assembly name
// Get all types
Type[] types = assembly.GetTypes();
foreach (Type type in types)
{
Console.WriteLine(type.FullName);
}
Examining a Type’s Base Classes
You can trace a type’s inheritance hierarchy up to the very root.
You can retrieve a type’s base class in C# using the BaseType
property of the System.Type
class. If you want to get the full inheritance chain, you can continue retrieving the BaseType
of the base type until you reach null
, which means you've reached the top of the inheritance hierarchy (the object
class).
Type type = typeof(MyDerivedClass); // Replace with your type
while (type != null)
{
Console.WriteLine(type.FullName);
type = type.BaseType;
}
Checking If a Type is Abstract or Sealed
Sometimes, you might want to determine if a type is abstract or sealed. you can use the IsAbstract
and IsSealed
properties of the System.Type
class to determine whether a type is abstract or sealed, respectively.
Type type = typeof(MyClass); // Replace with your type
bool isAbstract = type.IsAbstract;
bool isSealed = type.IsSealed;
Console.WriteLine($"Type {type.Name} is {(isAbstract ? "abstract" : "not abstract")}");
Console.WriteLine($"Type {type.Name} is {(isSealed ? "sealed" : "not sealed")}");
Examining a Type’s Implemented Interfaces
You can retrieve the interfaces implemented by a type using the GetInterfaces
method of the System.Type
class. This method returns an array of Type
objects that represent all the interfaces implemented by the current Type
.
Type type = typeof(MyClass); // Replace with your type
// Get all implemented interfaces
Type[] interfaces = type.GetInterfaces();
foreach (Type interfaceType in interfaces)
{
Console.WriteLine(interfaceType.FullName);
}
Determining If an Object Is an Instance of a Specific Type
Reflection allows you to check if an object is an instance of a specific type.
You can determine if an object is an instance of a specific type by using the is
keyword or the GetType()
method. The is
keyword checks if the run-time type of an object is compatible with a given type, and the GetType()
method gets the exact run-time type of an instance.
MyClass myObject = new MyClass();
if (myObject is MyClass)
{
Console.WriteLine("myObject is an instance of MyClass");
}
else
{
Console.WriteLine("myObject is not an instance of MyClass");
}
Creating a Type Instance at Runtime
You can create an instance of a type at runtime using the Activator.CreateInstance
method. This method uses reflection to create an instance of the specified type.
// Get the type
Type type = typeof(MyClass); // Replace with your type
// Create an instance of the type
object instance = Activator.CreateInstance(type);
// Cast the instance to the specific type
MyClass myInstance = (MyClass)instance;
The instance is created using the parameterless constructor. If you want to use a constructor that takes parameters, you need to pass the parameters as an array after the type:
// Create an instance using a constructor that takes a string parameter
object instance = Activator.CreateInstance(type, new object[] { "parameter" });
Creating a Type at Runtime
Using Reflection.Emit, you can define a new type, its members (fields, properties, methods, events, etc.), and even its IL at runtime.
AssemblyName assemblyName = new AssemblyName("DynamicAssembly");
AssemblyBuilder assemblyBuilder = AssemblyBuilder.DefineDynamicAssembly(assemblyName, AssemblyBuilderAccess.Run);
ModuleBuilder moduleBuilder = assemblyBuilder.DefineDynamicModule("DynamicModule");
// Define a public class named 'DynamicType' in the assembly.
TypeBuilder typeBuilder = moduleBuilder.DefineType("DynamicType", TypeAttributes.Public);
// Define a public string field named 'DynamicField' in the type.
FieldBuilder fieldBuilder = typeBuilder.DefineField("DynamicField", typeof(string), FieldAttributes.Public);
// Create the type.
Type dynamicType = typeBuilder.CreateType();
Creating an Instance of a Type Without Invoking Its Constructor
This can be useful for deserialization or cloning scenarios, where you want to populate an object’s fields or properties manually without invoking its constructor.
System.Runtime.Serialization.FormatterServices.GetUninitializedObject(Type type)
method can be used to create an instance of a type without invoking its constructor.
This method creates a new instance of the specified type, but unlike Activator.CreateInstance
, it does not call the object's constructor, hence the object is uninitialized.
Type type = typeof(MyClass); // Replace with your type
// Create an instance of the type without calling its constructor
object instance = FormatterServices.GetUninitializedObject(type);
// Now you can use the instance...
Creating Arrays of a Certain Type at Runtime
This can be useful when dealing with collections of objects that are determined at runtime.
You can create an array of a certain type at runtime using the Array.CreateInstance
method. This method returns a new array of a specified type with the specified length.
Type elementType = typeof(string);
int arrayLength = 5;
Array array = Array.CreateInstance(elementType, arrayLength);
// Now you can use this array as you would any other
for (int i = 0; i < array.Length; i++)
{
array.SetValue($"Element {i}", i);
}
// Print the elements to verify
for (int i = 0; i < array.Length; i++)
{
Console.WriteLine(array.GetValue(i));
}
Determining If a Type Is Marked with a Specific Attribute
This can be useful when your code needs to behave differently depending on the attributes applied to a type.
You can use the IsDefined
method of the System.Type
class to determine if a type is marked with a specific attribute. The IsDefined
method checks if the attribute class, specified by the type parameter, is applied to the type.
Type type = typeof(MyClass); // Replace with your type
// Check if the type is marked with the MyAttribute
bool hasAttribute = Attribute.IsDefined(type, typeof(MyAttribute));
Console.WriteLine(hasAttribute ? "Attribute is present." : "Attribute is not present.");
Invoking a Constructor Dynamically
Just as you can invoke methods dynamically, you can also invoke constructors. This is typically done by retrieving the ConstructorInfo
object representing the constructor you want to call, and then using its Invoke
method.
public class MyClass
{
public MyClass(string message)
{
Console.WriteLine(message);
}
}
//...
Type type = typeof(MyClass);
ConstructorInfo constructor = type.GetConstructor(new[] { typeof(string) });
object instance = constructor.Invoke(new object[] { "Hello, World!" });
Determining If a Type Is a ValueType or a ReferenceType
This can be handy in certain scenarios where you need to treat value types and reference types differently. You can determine if a type is a value type or a reference type by using the Type.IsValueType
property, which gets a value indicating whether the Type
is a value type.
Type type = typeof(int);
if (type.IsValueType)
{
Console.WriteLine($"{type.Name} is a value type.");
}
else
{
Console.WriteLine($"{type.Name} is a reference type.");
}
Getting the Underlying Type of Nullable Types
This can be helpful for generic code, where the exact type might be a nullable.
You can get the underlying type of a nullable type using the Nullable.GetUnderlyingType
method. This method returns the underlying type argument of the specified nullable type.
Type nullableType = typeof(int?);
Type underlyingType = Nullable.GetUnderlyingType(nullableType);
Console.WriteLine("The underlying type of {0} is {1}.", nullableType.Name, underlyingType.Name);
Finding All Types Derived from a Base Class or Interface
This can be particularly useful when creating plugin-based architectures or auto-registering classes in an IoC container:
public interface IMyInterface
{
void MyMethod();
}
public class MyClass : IMyInterface
{
public void MyMethod() { }
}
//...
Type targetType = typeof(IMyInterface);
List<Type> derivedTypes = new List<Type>();
foreach (var assembly in AppDomain.CurrentDomain.GetAssemblies())
{
foreach (var type in assembly.GetTypes())
{
if (targetType.IsAssignableFrom(type) && type.IsClass)
{
derivedTypes.Add(type);
}
}
}
//Print derived types
foreach (var type in derivedTypes)
{
Console.WriteLine(type.FullName);
}
Obtaining Full Method Signature
You can retrieve the full signature of a method, including its return type, name, type parameters, and parameter types. Here’s how you can do this:
public class TestClass
{
public void TestMethod<T>(int value, T item) { }
}
MethodInfo method = typeof(TestClass).GetMethod("TestMethod");
// Get return type
string returnType = method.ReturnType.Name;
// Get method name
string methodName = method.Name;
// Get generic arguments if any
string genericArguments = "";
if (method.IsGenericMethod)
{
Type[] arguments = method.GetGenericArguments();
genericArguments = string.Join(", ", arguments.Select(arg => arg.Name));
genericArguments = "<" + genericArguments + ">";
}
// Get parameters
string parameters = string.Join(", ", method.GetParameters().Select(param => $"{param.ParameterType.Name} {param.Name}"));
// Construct full method signature
string fullSignature = $"{returnType} {methodName}{genericArguments}({parameters})";
Console.WriteLine(fullSignature);
Calling a Method by Name
You can call a method by its name. This is particularly useful when the method to call is determined at runtime.
public class MyClass
{
public void MyMethod()
{
Console.WriteLine("MyMethod is called!");
}
}
//...
// Create an instance of MyClass
MyClass myObject = new MyClass();
// Get the Type information.
Type myType = myObject.GetType();
// Get the MethodInfo for the method
MethodInfo myMethod = myType.GetMethod("MyMethod");
// Invoke the method on the instance
myMethod.Invoke(myObject, null);
Dynamically Invoking Extension Methods
Normally, you can’t directly call an extension method using MethodInfo.Invoke
. However, by treating it as a static method of the static class that defines it, you can:
Invoking extension methods dynamically using Reflection can be a bit tricky compared to invoking normal methods. This is because extension methods are static methods that appear as instance methods due to C# syntactic sugar. You don’t invoke an extension method on an instance of a type, instead, the instance is passed as the first parameter to the static extension method.
Let’s illustrate this with an example. Suppose we have the following extension method for the string
type:
public static class StringExtensions
{
public static string AppendHello(this string str)
{
return str + " Hello";
}
}
To dynamically invoke this extension method using Reflection, you would do:
// Get the MethodInfo for the extension method
MethodInfo extensionMethod = typeof(StringExtensions).GetMethod("AppendHello");
// Prepare the parameters
object[] parameters = new object[] { "World" };
// Invoke the extension method and get the result
string result = (string)extensionMethod.Invoke(null, parameters);
Console.WriteLine(result); // Outputs: "World Hello"
Working with Indexers
You can use Reflection to get, set, and invoke indexers, which are special kinds of properties.
In C#, an indexer is a special kind of property that allows a class or struct to be accessed the same way as an array for its internal collection. This is particularly useful when you want to create your own custom collections.
When it comes to Reflection, indexers can be a bit tricky because they are represented as properties named Item
in the underlying IL code. You can use Reflection to get or set the values of an indexer just like you would with a property.
Here’s an example of using Reflection to work with an indexer in C#:
public class MyCollection
{
private List<string> items = new List<string> { "Hello", "World" };
public string this[int index]
{
get { return items[index]; }
set { items[index] = value; }
}
}
//...
// Create an instance of MyCollection
MyCollection collection = new MyCollection();
// Get the Type information
Type type = collection.GetType();
// Get the PropertyInfo for the indexer
PropertyInfo indexerProperty = type.GetProperty("Item");
// Get the value of the indexer for the first item
object value = indexerProperty.GetValue(collection, new object[] { 0 });
Console.WriteLine(value); // Outputs: Hello
// Set the value of the indexer for the first item
indexerProperty.SetValue(collection, "Hi", new object[] { 0 });
// Check the changed value
value = indexerProperty.GetValue(collection, new object[] { 0 });
Console.WriteLine(value); // Outputs: Hi
Detecting a Property’s Getter and Setter
Reflection allows you to determine whether a property has a getter or a setter.
Detecting whether a property has a getter or setter (or both) can be accomplished using Reflection. In C#, properties are members of classes, struct, or interfaces and are typically used as public data members. However, unlike fields, properties provide a layer of abstraction by exposing get and set accessors.
public class MyClass
{
public int MyProperty { get; set; } // Property with both getter and setter
public int MyPropertyGet { get; } // Property with getter only
}
// ...
// Create an instance of MyClass
MyClass myObject = new MyClass();
// Get the Type information.
Type myType = myObject.GetType();
// Get the PropertyInfo for each property
PropertyInfo myProperty = myType.GetProperty("MyProperty");
PropertyInfo myPropertyGet = myType.GetProperty("MyPropertyGet");
// Check if the properties have a getter or setter
Console.WriteLine("MyProperty has getter: " + (myProperty.GetMethod != null)); // Outputs: True
Console.WriteLine("MyProperty has setter: " + (myProperty.SetMethod != null)); // Outputs: True
Console.WriteLine("MyPropertyGet has getter: " + (myPropertyGet.GetMethod != null)); // Outputs: True
Console.WriteLine("MyPropertyGet has setter: " + (myPropertyGet.SetMethod != null)); // Outputs: False
Changing Field or Property Values of Immutable Objects
Even if an object is designed to be immutable, Reflection allows changing the values of its fields or properties.
Immutable objects are designed not to allow their state to be changed once they are created. However, with the power of Reflection, it is technically possible to alter the state of an immutable object by directly modifying its fields, although this goes against the principle of immutability and should be done very cautiously.
Let’s consider the System.String
class, which is immutable in C#. That is, once a String
object is created, its value cannot be changed. But using Reflection, we can forcefully change its value:
string str = "Hello";
Console.WriteLine(str); // Outputs: Hello
// Get the 'm_stringLength' field information
var stringLengthField = typeof(string).GetField("m_stringLength", BindingFlags.NonPublic | BindingFlags.Instance);
// Change the length of the string
stringLengthField.SetValue(str, 3);
// Get the 'm_firstChar' field information
var firstCharField = typeof(string).GetField("m_firstChar", BindingFlags.NonPublic | BindingFlags.Instance);
// Create a char array of the new value
char[] newValue = "Hey".ToCharArray();
// Change the value of the string
firstCharField.SetValue(str, newValue[0]);
// Verify the change
Console.WriteLine(str); // Outputs: Hey
Listing Custom Attributes on a Member
You can extract custom attributes from a class, method, property, or other members. This is handy for creating your own custom attributes for marking or annotating code:
You can list custom attributes on a member using the MemberInfo.GetCustomAttributes
method. This method returns an array that contains all the custom attributes applied to a member.
public class MyClass
{
[Obsolete("This method is obsolete.")]
[Description("This is a sample method.")]
public void MyMethod() { }
}
// ...
MethodInfo method = typeof(MyClass).GetMethod("MyMethod");
Attribute[] attributes = Attribute.GetCustomAttributes(method);
foreach (Attribute attribute in attributes)
{
Console.WriteLine("Attribute on MyMethod: " + attribute.GetType().Name);
}
Dynamically Creating Delegates for Methods
This can improve the performance of invoking methods via Reflection.
Delegates are a way to encapsulate a method into a callable object in C#. They’re particularly useful when you want to pass a method as an argument, use a method as an event handler, or pair methods together for easy invocation.
Using Reflection, you can dynamically create delegates for methods. This can be useful when the method you want to encapsulate into a delegate isn’t known until runtime.
public class MyClass
{
public void MyMethod()
{
Console.WriteLine("MyMethod is called!");
}
}
// Create an instance of MyClass
MyClass myObject = new MyClass();
// Get the Type information.
Type myType = myObject.GetType();
// Get the MethodInfo for the method
MethodInfo myMethod = myType.GetMethod("MyMethod");
// Create a delegate for the method
Action myDelegate = (Action)Delegate.CreateDelegate(typeof(Action), myObject, myMethod);
// Invoke the delegate
myDelegate();
Checking If a Delegate References a Specific Method
You can check if a delegate references a specific method, which can be useful for ensuring a delegate isn’t invoked twice for the same method.
A delegate in C# is a reference type data type that holds the reference to a method. Sometimes, you might want to check if a delegate references a particular method.
Consider the following delegate and methods:
public delegate void MyDelegate(string message);
public class MyClass
{
public void Method1(string message)
{
Console.WriteLine($"Method1 says: {message}");
}
public void Method2(string message)
{
Console.WriteLine($"Method2 says: {message}");
}
}
Now, we can create a delegate instance referencing Method1
:
MyClass myClass = new MyClass();
MyDelegate myDelegate = myClass.Method1;
To check if myDelegate
references Method1
, we can do the following:
MethodInfo methodInfo = myDelegate.Method;
bool referencesMethod1 = methodInfo.Name == nameof(MyClass.Method1);
Console.WriteLine(referencesMethod1); // Outputs: True
Creating a Delegate for a Property Getter or Setter
This can provide a significant performance improvement when getting or setting property values dynamically.
Creating delegates for a property’s getter or setter can allow you to access those methods dynamically at runtime. Here’s how you can create delegates for property getters and setters:
Consider we have a class Person
:
public class Person
{
public string Name { get; set; }
}
You can create a delegate for the Name
property's getter like this:
Person person = new Person { Name = "Alice" };
// Get the PropertyInfo for the Name property
PropertyInfo propertyInfo = typeof(Person).GetProperty("Name");
// Get the getter method for the property
MethodInfo getter = propertyInfo.GetGetMethod();
// Create a delegate for the getter
Func<Person, string> getterDelegate = (Func<Person, string>)Delegate.CreateDelegate(typeof(Func<Person, string>), getter);
// Now you can use the delegate to get the property value
string name = getterDelegate(person);
Console.WriteLine(name); // Outputs: Alice
To create a delegate for the setter, you can do something similar:
// Get the setter method for the property
MethodInfo setter = propertyInfo.GetSetMethod();
// Create a delegate for the setter
Action<Person, string> setterDelegate = (Action<Person, string>)Delegate.CreateDelegate(typeof(Action<Person, string>), setter);
// Now you can use the delegate to set the property value
setterDelegate(person, "Bob");
Console.WriteLine(person.Name); // Outputs: Bob
Dynamically Adding Events
Reflection allows you to dynamically add events to an object.
Dynamically adding events in C# is a powerful technique which can be done using Reflection. Events in C# are a way for a class to provide notifications to clients of that class when some interesting thing happens to an object. The most familiar use for events is in graphical user interfaces; typically, the classes that describe controls in the interface have events that are notified when the user does something to the control (like click a button).
public class MyClass
{
public event Action MyEvent;
public void TriggerEvent()
{
MyEvent?.Invoke();
}
}
public class MyListener
{
public void OnEvent()
{
Console.WriteLine("Event received!");
}
}
//...
// Create the objects
MyClass myObject = new MyClass();
MyListener listener = new MyListener();
// Get the EventInfo for the event
EventInfo myEvent = typeof(MyClass).GetEvent("MyEvent");
// Get the MethodInfo for the listener method
MethodInfo listenerMethod = typeof(MyListener).GetMethod("OnEvent");
// Create a delegate for the listener method
Delegate myDelegate = Delegate.CreateDelegate(myEvent.EventHandlerType, listener, listenerMethod);
// Add the event handler
myEvent.AddEventHandler(myObject, myDelegate);
// Trigger the event
myObject.TriggerEvent(); // Outputs: "Event received!"
Inspecting Enumerations
Reflection allows you to inspect enumeration types. You can obtain the names and values of an enum type.
Enumerations in C# (also known as Enums) are a type that consists of a set of named constants. Reflection can be used to inspect and work with enums in ways that might not be possible with the normal enum syntax.
Let’s consider the following enum:
public enum Color
{
Red,
Blue,
Green
}
We can inspect the names and values of this enumeration like so:
Type colorType = typeof(Color);
foreach (var name in Enum.GetNames(colorType))
{
Console.WriteLine($"Name: {name}, Value: {(int)Enum.Parse(colorType, name)}");
}
This code will output:
Name: Red, Value: 0
Name: Blue, Value: 1
Name: Green, Value: 2
Enum.GetNames
retrieves all the names of the enum as a string array, and Enum.Parse
is used to get the corresponding value for each name.
You can also use Enum.GetValues
to retrieve an array of all the values in the enum:
foreach (var value in Enum.GetValues(colorType))
{
Console.WriteLine($"Name: {Enum.GetName(colorType, value)}, Value: {(int)value}");
}
This will output the same results as the previous example.
Another useful operation is to check if a specific string or value corresponds to a valid enum member. You can do this with Enum.IsDefined
:
bool isValidName = Enum.IsDefined(colorType, "Red"); // Outputs: True
bool isValidValue = Enum.IsDefined(colorType, 3); // Outputs: False
Accessing Private Members
Perhaps one of the most intriguing uses of Reflection is its ability to access private members of a class. While this power should be used judiciously and responsibly (given that private members are private for a reason), it can come in handy in certain situations such as debugging or testing.
Consider the following class:
public class SecretHolder
{
private string secret = "The cake is a lie";
}
Normally, you wouldn’t be able to access the secret
field from outside the class. But with Reflection, you can! Here's how:
SecretHolder holder = new SecretHolder();
FieldInfo secretField = typeof(SecretHolder).GetField("secret", BindingFlags.NonPublic | BindingFlags.Instance);
string secretValue = (string)secretField.GetValue(holder);
Console.WriteLine(secretValue); // Outputs: "The cake is a lie"
As you can see, Reflection allows us to break down the usual boundaries of encapsulation in C#.
Generating Code on the Fly
One of the most impressive tricks you can do with Reflection is generate code on the fly. The .NET framework includes a namespace called System.Reflection.Emit
which provides classes for creating and saving assemblies, types, and methods dynamically.
For instance, imagine you want to create a new class at runtime that has a single method which prints a message. Here’s how you could accomplish that:
AssemblyName assemblyName = new AssemblyName("DynamicAssembly");
AssemblyBuilder assemblyBuilder = AppDomain.CurrentDomain.DefineDynamicAssembly(assemblyName, AssemblyBuilderAccess.Run);
ModuleBuilder moduleBuilder = assemblyBuilder.DefineDynamicModule("MainModule");
TypeBuilder typeBuilder = moduleBuilder.DefineType("DynamicType", TypeAttributes.Public | TypeAttributes.Class);
MethodBuilder methodBuilder = typeBuilder.DefineMethod("PrintMessage", MethodAttributes.Public, null, null);
ILGenerator ilGenerator = methodBuilder.GetILGenerator();
ilGenerator.Emit(OpCodes.Ldstr, "Hello from a dynamic method!");
ilGenerator.Emit(OpCodes.Call, typeof(Console).GetMethod("WriteLine", new Type[] { typeof(string) }));
ilGenerator.Emit(OpCodes.Ret);
Type dynamicType = typeBuilder.CreateType();
dynamicType.GetMethod("PrintMessage").Invoke(Activator.CreateInstance(dynamicType), null);
This will print “Hello from a dynamic method!” to the console. Even though this is a simple example, it illustrates the potential of dynamically generating code with Reflection.
Manipulating Generic Types
Reflection is a powerful tool when working with generic types. It allows you to create instances of generic types, call generic methods, and more, all at runtime.
For instance, suppose you have a generic class and you want to create an instance of it with a specific type argument, but this type is only known at runtime. Here’s how you could do this:
public class GenericClass<T>
{
public T Value { get; set; }
}
string typeName = "System.String";
Type genericType = typeof(GenericClass<>).MakeGenericType(Type.GetType(typeName));
object instance = Activator.CreateInstance(genericType);
Here, we create an instance of GenericClass<string>
, even though we only knew we wanted to use string
as the type argument at runtime.
Finding Out if a Class Implements a Specific Interface
Reflection can be used to find out if a class implements a specific interface. This can be useful when dealing with plugin systems or handling objects dynamically. You can use the IsAssignableFrom
method or the GetInterface
method of the System.Type
class to determine if a class implements a specific interface.
Using IsAssignableFrom:
// Assuming MyClass implements IMyInterface
bool implementsInterface = typeof(IMyInterface).IsAssignableFrom(typeof(MyClass));
if (implementsInterface)
{
Console.WriteLine("MyClass implements IMyInterface.");
}
else
{
Console.WriteLine("MyClass does not implement IMyInterface.");
}
Using GetInterface:
// GetInterface returns null if the interface isn't found
Type interfaceType = typeof(MyClass).GetInterface("IMyInterface");
if (interfaceType != null)
{
Console.WriteLine("MyClass implements IMyInterface.");
}
else
{
Console.WriteLine("MyClass does not implement IMyInterface.");
}
Building LINQ Expressions Dynamically
In some scenarios, you might want to build LINQ expressions dynamically at runtime. For instance, you might want to create a filtering mechanism for a database query that depends on user input or other runtime variables. This can be accomplished with the System.Linq.Expressions
namespace, which allows you to build expression trees.
Let’s consider a simple example, where we create an expression to filter a list of people based on their age:
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
// ... elsewhere in your code
// Assume we have a List<Person> named people
int targetAge = 30; // Assume this could change at runtime
// Build the expression
ParameterExpression pe = Expression.Parameter(typeof(Person), "person");
Expression left = Expression.Property(pe, typeof(Person).GetProperty("Age"));
Expression right = Expression.Constant(targetAge);
Expression comparison = Expression.GreaterThan(left, right);
// Compile and run the expression
Func<Person, bool> ageFilter = Expression.Lambda<Func<Person, bool>>(comparison, pe).Compile();
List<Person> filteredPeople = people.Where(ageFilter).ToList();
Inspecting and Modifying a Method’s IL
Inspecting and modifying a method’s Intermediate Language (IL) code directly is a more advanced and complex operation that should be used with care. That said, there are libraries available that allow you to do this, such as the open-source library Mono.Cecil.
Here’s a basic example of how to read a method’s IL code with Mono.Cecil:
using Mono.Cecil;
using Mono.Cecil.Cil;
using System;
public class Program
{
public static void Main()
{
// Load the assembly containing the method you want to inspect
AssemblyDefinition assembly = AssemblyDefinition.ReadAssembly(typeof(Program).Assembly.Location);
// Get the method you want to inspect
MethodDefinition method = assembly.MainModule.GetType("Program").Methods.First(m => m.Name == "MethodToInspect");
// Print the IL instructions of the method
foreach (Instruction instruction in method.Body.Instructions)
{
Console.WriteLine(instruction);
}
}
public static void MethodToInspect()
{
Console.WriteLine("This is the method to inspect");
}
}
Please note that modifying IL code can be very complex and risky if you are not thoroughly familiar with the IL language, as it’s easy to create invalid or unsafe code. Additionally, modifying the IL code of an existing assembly typically involves rewriting the assembly, which can have side effects and potential legal and security issues.
Dynamically Building and Compiling a Complete C# Class
Creating a dynamic C# class involves generating a C# source code string at runtime, compiling this source code into an assembly, and then using reflection to access the compiled code. This can be done using the CSharpCodeProvider
class in the System.CodeDom.Compiler
namespace.
Let’s consider an example:
using System;
using System.CodeDom.Compiler;
using Microsoft.CSharp;
public class Program
{
static void Main()
{
// Define the source code string
string sourceCode = @"
public class DynamicClass
{
public static void DynamicMethod()
{
System.Console.WriteLine(""Hello from DynamicMethod!"");
}
}";
// Set compiler parameters
CompilerParameters parameters = new CompilerParameters();
parameters.GenerateInMemory = true;
parameters.ReferencedAssemblies.Add("System.dll");
// Compile the source code
CSharpCodeProvider codeProvider = new CSharpCodeProvider();
CompilerResults results = codeProvider.CompileAssemblyFromSource(parameters, sourceCode);
if (results.Errors.Count > 0)
{
foreach (CompilerError error in results.Errors)
{
Console.WriteLine(error.ErrorText);
}
}
else
{
// Access the compiled code
Type dynamicType = results.CompiledAssembly.GetType("DynamicClass");
dynamicType.GetMethod("DynamicMethod").Invoke(null, null);
}
}
}
Avoiding Performance Pitfalls
One common concern about using Reflection is its impact on performance. Indeed, Reflection can be slower than direct calls, as it involves a level of indirection and requires inspecting metadata. However, it’s essential to keep this in context. Most of the time, the difference in performance won’t be noticeable unless you’re using Reflection in a performance-critical loop or in high-frequency operations.
That said, there are ways to minimize the performance impact:
- Caching Results: If you’re repeatedly retrieving the same type, method, or field information, consider caching the results. This can significantly reduce the overhead of metadata lookup.
- Using
Typeof
Instead of GetType
: When possible, use typeof(ClassName)
instead of instance.GetType()
. The former is evaluated at compile-time and is faster.
- Avoiding Unnecessary Use: Don’t use Reflection when there are simpler, direct ways to accomplish the same thing.
As a rule of thumb, use Reflection only when necessary. If there’s a direct way to accomplish what you need, it’s generally better to go that route. Reflection should be your tool of choice when you need to do things that are impossible or awkward to do without it, such as accessing private members for testing, implementing plugin architectures, or working with types and methods that are only known at runtime.
Reflection is a powerful tool, but like any tool, it’s most effective when used correctly. We hope these pro tips help you in your journey to master Reflection in C#. As with any topic in programming, the best way to learn is by doing, so don’t be afraid to experiment, make mistakes, and learn from them.