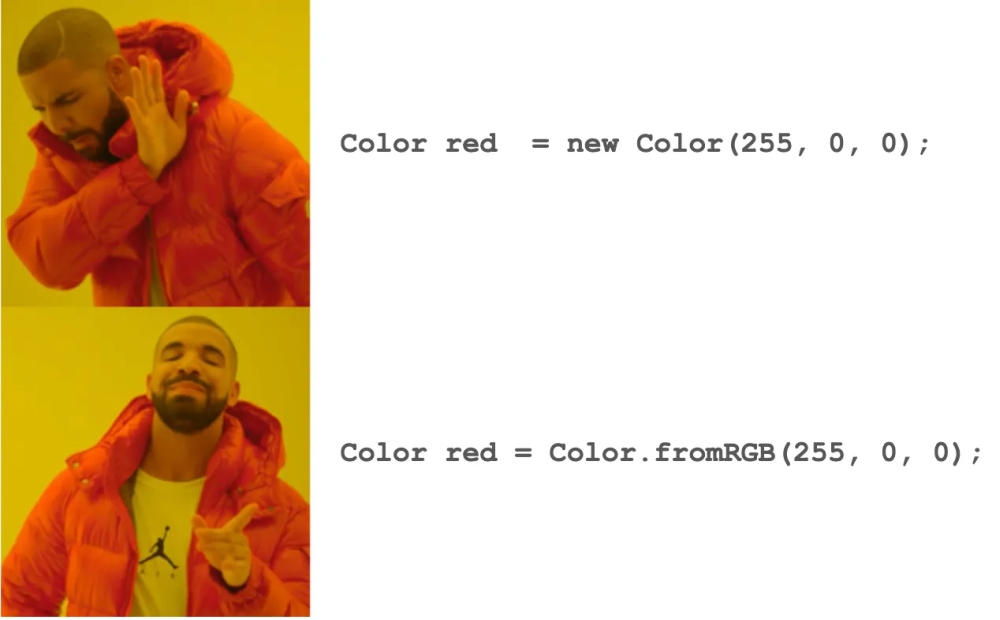
Constructor
We have been using constructors in object-oriented programming languages such as Java, C++, etc. A constructor is a special type of static method which can be invoked using thenew keyword and used to initialize the fields of a newly created object.
Problem with constructors
- The name of the constructor must match the name of the class, hence you cannot give a meaningful name to the constructor.
- The return type is not applicable to the constructor, hence you can always expect the object of the same class. It means you cannot return the object of its subtype of the class.
- You cannot mock a constructor for unit testing.
Static factory methods
The static factory method is another way of creating and initializing an object. Using the static factory method instead of a constructor allows you to return objects of different subtypes based on the arguments, making the code more flexible and easier to understand.
Additionally, static factory methods can have more descriptive names, making the code more self-explanatory, and they can also return objects that are already created, which can help to reduce unnecessary object creation and improve performance.
Note: Static factory methods are not the same as the factory design pattern.
Example
public class ComplexNumber
{
private final double realPart;
private final double imaginaryPart;
private ComplexNumber(double realPart, double imaginaryPart)
{
this.realPart = realPart;
this.imaginaryPart = imaginaryPart;
}
public static ComplexNumber fromRealNumber(double realPart)
{
return new ComplexNumber(realPart, 0);
}
public static ComplexNumber fromImaginaryNumber(double imaginaryPart)
{
return new ComplexNumber(0, imaginaryPart);
}
// ... other methods ...
}
In this approach, the developer can create instances of ComplexNumber
by calling the appropriate static factory method, such as ComplexNumber.fromRealNumber(3.14)
. This can be more readable and expressive than using a constructor, especially when the construction process is more complex or involves multiple steps.
Few use cases in Java platform classes
Here are a few examples of Java built-in classes that use static factory methods:
Optional
Instead of using a constructor to create an instance of Optional
, you can use static factory methods such as of
, ofNullable
, and empty
. For example:
Optional<String> programmer = Optional.of("Mia");
LocalDate
LocalDate dateOfBirth = LocalDate.of(2020, 1, 1);
Collections
List<String> emptyList = List.of();
Set<String> numbers = Set.of(1, 5, 10);
Map<String, Integer> emptyMap = Collections.emptyMap();
Also, In Effective Java, Joshua Bloch clearly states that “Consider static factory methods instead of constructors” in this book.
Conclusion
This article explained the features of constructors in object-oriented programing languages, their cons, why a static factory method is useful, and what it can do that a constructor cannot do.
After all, there is no hard and fast rule for following this approach. It's completely up to the developer to choose the preferable way.