In today’s fast-paced business environment, efficiency and responsiveness are crucial for maintaining a competitive edge. Background batch processing plays a pivotal role in achieving these goals by handling time-consuming tasks asynchronously, thereby freeing up system resources and ensuring a seamless user experience. This technique is particularly essential in scenarios where large volumes of data need to be processed, or where tasks must be executed at regular intervals without direct user interaction.
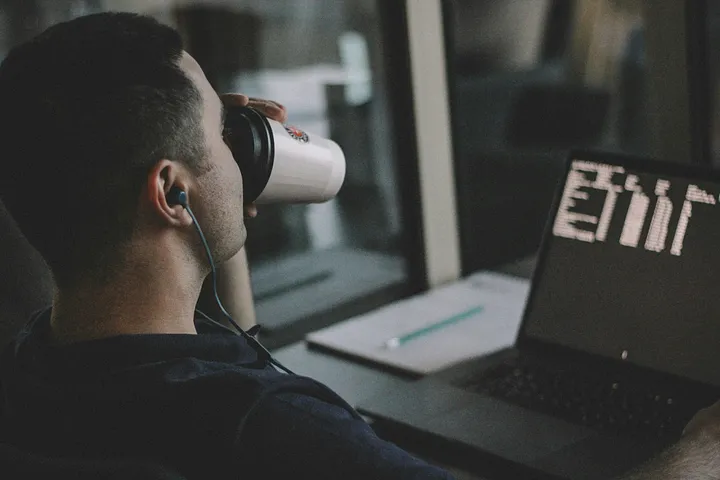
Common business scenarios for background batch processing
- E-commerce platforms: Updating inventory levels, processing orders, and managing user notifications.
- Financial services: Running nightly reports, processing transactions, and monitoring fraud detection algorithms.
- Healthcare systems: Updating patient records, processing insurance claims, and managing appointment schedules.
- Social media platforms: Processing user activity logs, updating recommendation algorithms, and handling content moderation tasks.
Implementing background batch processing in .NET
Implementing background batch processing in .NET can be approached in various ways, depending on the specific needs of the business and the existing technological stack. Here are some effective methods:
1. Using the async keyword in C#
The async
keyword in C# allows for asynchronous execution of tasks, meaning that certain operations can be performed without blocking the main execution thread. This is particularly useful for background processing where tasks can be performed concurrently with other operations.
Example in C#:
public async Task ProcessBatchAsync()
{
var data = await FetchDataAsync();
await ProcessDataAsync(data);
Console.WriteLine("Batch processing completed");
}
public void ScheduleBatchProcessing()
{
var timer = new System.Threading.Timer(
async e => await ProcessBatchAsync(),
null,
TimeSpan.Zero,
TimeSpan.FromMinutes(5)
);
}
Trade-offs:
- Advantages: Simple to implement within an existing codebase, efficient use of system resources, non-blocking execution enhances user experience.
- Disadvantages: Limited to environments that support async/await, not suitable for extremely large-scale operations.
When to choose:
Using the async keyword in C# improves application responsiveness and efficiency, especially for I/O-bound operations or background processing. For instance, when sending emails after an order is placed, it prevents users from waiting unnecessarily and allows the application to proceed to the next step promptly. However, effective implementation should consider application-specific needs and constraints.
2. Background job scheduler (Hangfire)
Hangfire is a popular library for background job scheduling in .NET. It allows you to create and manage background jobs with ease, and it integrates seamlessly with various storage backends like SQL Server.
Example with Hangfire:
public void ConfigureServices(IServiceCollection services)
{
services.AddHangfire(x => x.UseSqlServerStorage("YourConnectionString"));
services.AddHangfireServer();
}
public void Configure(IApplicationBuilder app, IBackgroundJobClient backgroundJobs)
{
app.UseHangfireDashboard();
backgroundJobs.Enqueue(() => Console.WriteLine("Background job started"));
RecurringJob.AddOrUpdate(() => ProcessPendingRecords(), Cron.MinuteInterval(5));
}
public void ProcessPendingRecords()
{
var records = GetPendingRecords();
if (records.Any())
{
ProcessRecords(records);
Console.WriteLine("Records processed");
}
}
Trade-offs:
- Advantages: Automated and reliable execution, ideal for periodic tasks, scalable for moderate data loads.
- Disadvantages: Requires additional libraries or tools, may need manual intervention for error handling and recovery.
When to choose:
Hangfire offers automation and scalability for periodic tasks. However, it introduces additional dependencies and may require manual effort for advanced error handling and recovery scenarios.
3. Windows Task Scheduler
The Windows Task Scheduler allows you to automate the execution of programs or scripts at specified times or intervals. This method is straightforward and useful for running .exe files or batch scripts.
Example using Windows Task Scheduler:
- Open Task Scheduler.
- Create a new basic task.
- Set the trigger to “Daily” and specify the interval.
- Set the action to “Start a Program” and browse to your .exe file or script.
- Finish and save the task.
Trade-offs:
- Advantages: Simple to set up, no additional coding required, reliable execution.
- Disadvantages: Limited to Windows environments, less flexible for complex job definitions.
When to choose:
Windows Task Scheduler is an effective choice for automating routine tasks on Windows systems, offering simplicity and reliability without requiring programming expertise. However, it may not meet the needs of environments requiring cross-platform compatibility or sophisticated job scheduling capabilities.
4. Cron jobs
Utilizing cron jobs for scheduling tasks on Unix-like systems is a straightforward method for periodic batch processing. In a .NET context, this can be achieved using Quartz.NET, a powerful and flexible job scheduling library.
Example with Quartz.NET:
public class BatchJob : IJob
{
public Task Execute(IJobExecutionContext context)
{
return ProcessPendingRecords();
}
}
public void ConfigureServices(IServiceCollection services)
{
services.AddQuartz(q =>
{
q.UseMicrosoftDependencyInjectionScopedJobFactory();
var jobKey = new JobKey("BatchJob");
q.AddJob<BatchJob>(opts => opts.WithIdentity(jobKey));
q.AddTrigger(opts => opts
.ForJob(jobKey)
.WithIdentity("BatchJob-trigger")
.WithCronSchedule("0 */5 * * * ?")); // Every 5 minutes
});
services.AddQuartzHostedService(q => q.WaitForJobsToComplete = true);
}
Trade-offs:
- Advantages: Simple and reliable for periodic tasks, well-suited for Unix-like environments.
- Disadvantages: Limited flexibility in job definitions, potential challenges in error handling, and job recovery.
When to choose:
Quartz.NET with cron jobs is a robust choice for straightforward periodic batch processing tasks in a .NET environment, particularly suitable for Unix-like systems. However, it may require additional effort to manage error scenarios and lacks the flexibility of more advanced job scheduling frameworks in certain complex use cases.
5. Asynchronous queues (Azure Service Bus)
Using asynchronous messaging queues like Azure Service Bus is an excellent approach for handling high-throughput background processing tasks. These systems decouple the task producer and consumer, enabling efficient and reliable message handling.
Example with Azure Service Bus:
Producer:
var client = new QueueClient(connectionString, queueName);
var message = new Message(Encoding.UTF8.GetBytes("Task data"));
await client.SendAsync(message);
Console.WriteLine("Sent message");
Consumer:
var client = new QueueClient(connectionString, queueName);
client.RegisterMessageHandler(
async (message, token) =>
{
var data = Encoding.UTF8.GetString(message.Body);
await ProcessDataAsync(data);
await client.CompleteAsync(message.SystemProperties.LockToken);
Console.WriteLine("Processed message");
},
new MessageHandlerOptions(ExceptionHandler) { MaxConcurrentCalls = 1, AutoComplete = false }
);
Task ExceptionHandler(ExceptionReceivedEventArgs exceptionReceivedEventArgs)
{
Console.WriteLine($"Message handler encountered an exception {exceptionReceivedEventArgs.Exception}.");
return Task.CompletedTask;
}
Trade-offs:
- Advantages: High scalability and reliability, suitable for distributed systems, provides fault tolerance and load balancing.
- Disadvantages: Requires setup and maintenance of additional infrastructure, increased complexity in system design. This also requires an Azure paid subscription.
When to choose:
Azure Service Bus, it excels in scenarios requiring high scalability, reliability, fault tolerance, and load balancing for distributed systems. This makes it particularly effective for handling high-throughput background processing tasks in an Azure-native environment. However, adopting Azure Service Bus does necessitate an Azure paid subscription and involves setting up and maintaining additional infrastructure, which can add complexity to system design.
6. Using Kafka
Apache Kafka is a highly scalable and distributed streaming platform. It is ideal for building real-time data pipelines and streaming applications, enabling you to handle high-throughput background processing tasks efficiently.
Example with Kafka:
Producer:
var config = new ProducerConfig { BootstrapServers = "localhost:9092" };
using (var producer = new ProducerBuilder<Null, string>(config).Build())
{
var result = await producer.ProduceAsync("task-topic", new Message<Null, string> { Value = "Task data" });
Console.WriteLine($"Sent message to {result.TopicPartitionOffset}");
}
Consumer:
var config = new ConsumerConfig
{
GroupId = "task-group",
BootstrapServers = "localhost:9092",
AutoOffsetReset = AutoOffsetReset.Earliest
};
using (var consumer = new ConsumerBuilder<Ignore, string>(config).Build())
{
consumer.Subscribe("task-topic");
while (true)
{
var consumeResult = consumer.Consume();
await ProcessDataAsync(consumeResult.Message.Value);
Console.WriteLine($"Processed message: {consumeResult.Message.Value}");
}
}
Trade-offs:
- Advantages: High scalability, fault tolerance, and reliability, suitable for real-time data processing.
- Disadvantages: Requires setup and maintenance of Kafka infrastructure, increased complexity in system design.
When to choose:
Choose Kafka when you need robust scalability, fault tolerance, and reliable real-time data processing. It excels in handling high-throughput data pipelines and distributed streaming applications, making it ideal for scenarios demanding strong data integrity and continuous data flow. However, implementing Kafka requires careful setup and ongoing maintenance of its infrastructure, which can introduce complexity to your system architecture and operations.
7. Azure Functions
Azure Functions is a serverless computing service that enables you to run code on-demand without provisioning or managing infrastructure. It is ideal for background processing tasks triggered by various events or schedules.
Example with Azure Functions:
public static class TimerFunction
{
[FunctionName("TimerFunction")]
public static async Task Run([TimerTrigger("*/5 * * * *")]TimerInfo myTimer, ILogger log)
{
await ProcessPendingRecordsAsync();
log.LogInformation($"C# Timer trigger function executed at: {DateTime.Now}");
}
}
Trade-offs:
- Advantages: Serverless and scalable, easy to set up and maintain, integrates with various Azure services.
- Disadvantages: Potential cold start latency, dependent on Azure infrastructure. This requires a paid Azure subscription.
When to choose:
Azure Functions are particularly effective for executing precise background jobs at specific times, especially within an Azure-native environment. However, if you do not already have an Azure setup, adopting them may introduce additional complexity.
8. Database triggers
Database triggers can be used to initiate batch-processing tasks based on specific changes or events in the database. This method ensures that tasks are processed immediately after the triggering event.
Example with SQL Server Trigger:
CREATE TRIGGER ProcessRecordsTrigger
ON YourTable
AFTER INSERT, UPDATE
AS
BEGIN
-- Call your batch processing logic here
EXEC dbo.ProcessRecords;
END;
Trade-offs:
- Advantages: Immediate response to database changes, tightly integrated with data operations.
- Disadvantages: Increased complexity in database logic, potential performance impact on database operations.
When to choose:
Database triggers are most suitable for scenarios where you need to change records based on updates or deletions, or when managing dependent records. For example, you might use triggers to log every deleted inventory item into a dedicated history table.
Conclusion
Background batch processing is critical for optimizing business operations and enhancing system performance. By selecting the appropriate method — whether using async keywords, background job schedulers, Windows Task Scheduler, cron jobs, asynchronous queues, Kafka, Azure Functions, or database triggers—businesses can effectively manage their processing needs and ensure efficient and reliable task execution. Each method has its trade-offs, so it's essential to consider the specific requirements and constraints of your business scenario when making a decision.