Pagination is in front of us everyday yet we take it for granted kind of like we do with most things. It’s what chunks huge lists of data (blog posts, articles, products) into pages so we can navigate through data.
This is how you did pagination until this point.
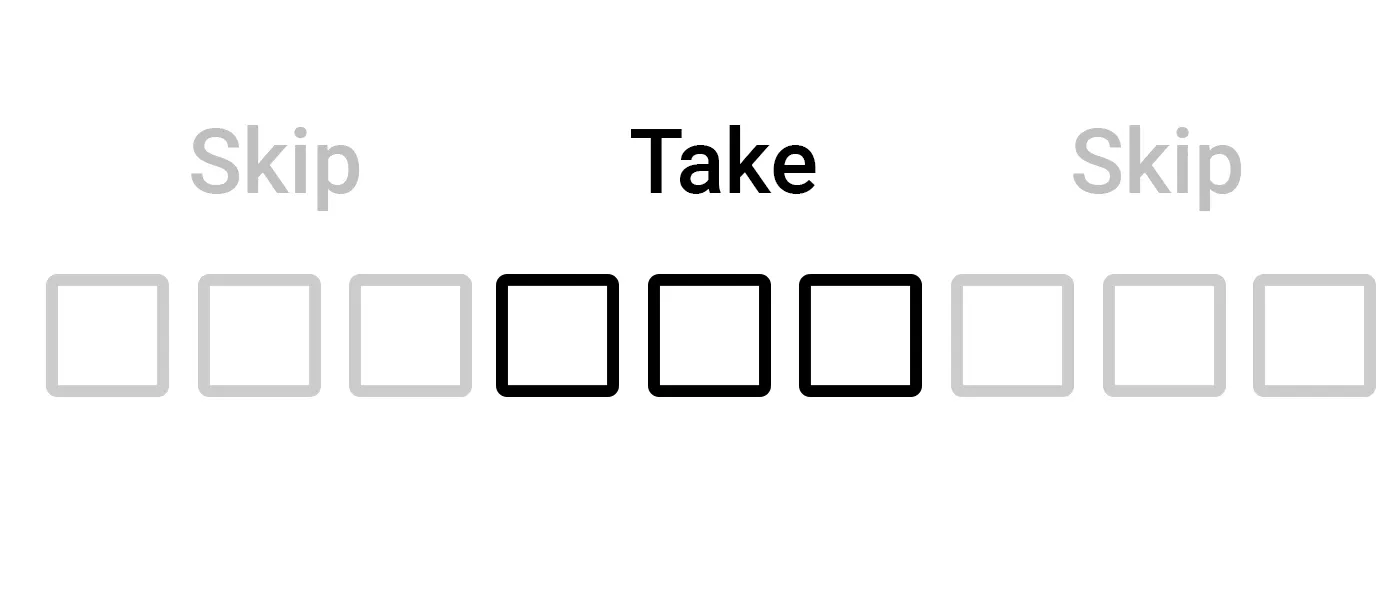
using System;
public class Article
{
public int Id;
public string Title;
public string Content;
public int AuthorId;
public List<Article> GetArticles()
{
return new List<Article> {
new Article {Id = 1, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 2, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 3, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
new Article {Id = 4, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 5, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 6, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
new Article {Id = 7, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 8, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 9, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
new Article {Id = 10, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 11, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 12, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
};
}
}
public class Program
{
public static void Main()
{
List<Article> articles = new Article().GetArticles();
int pageNumber = 1;
int itemsPerPage = 5;
IEnumerable<Article> page = articles.Skip((pageNumber - 1) * itemsPerPage).Take(itemsPerPage);
Console.WriteLine($"Page: {pageNumber}");
foreach(Article article in page)
{
Console.WriteLine($" Id: {article.Id}, Title:{article.Title}, Content:{article.Content}, AuthorId:{article.AuthorId}");
}
}
}
And now this is how you can achieve the same result but in a more readable way using the Chunk method.
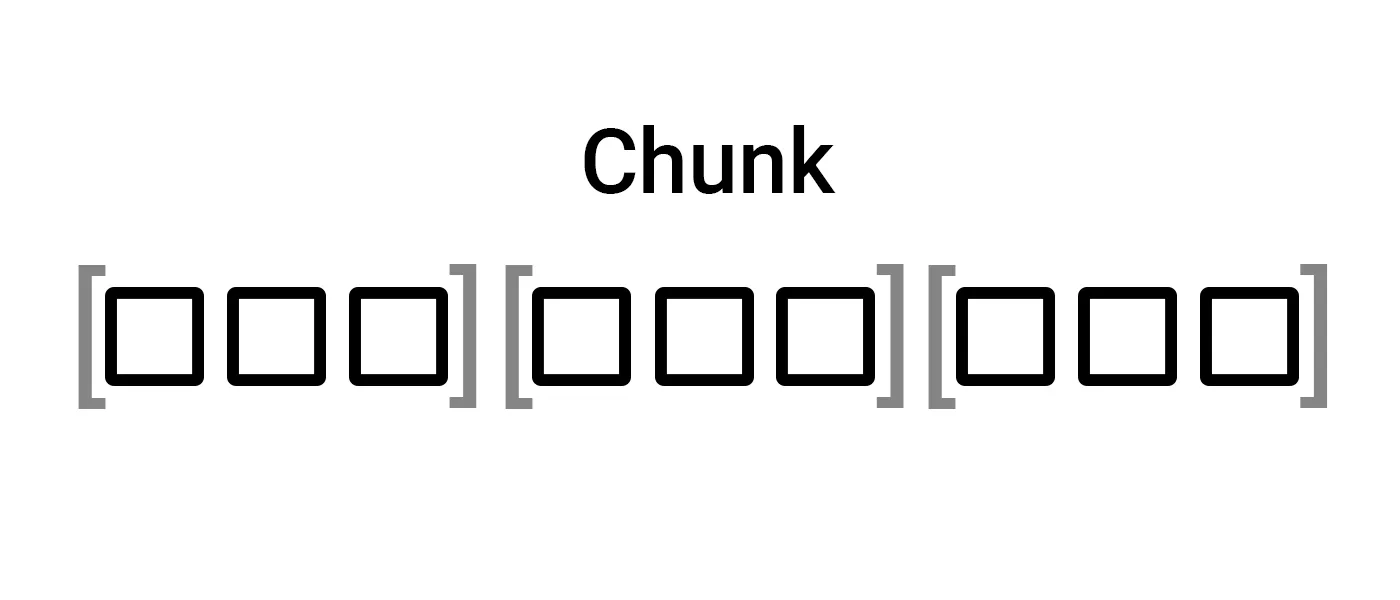
using System;
public class Article
{
public int Id;
public string Title;
public string Content;
public int AuthorId;
public List<Article> GetArticles()
{
return new List<Article> {
new Article {Id = 1, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 2, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 3, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
new Article {Id = 4, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 5, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 6, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
new Article {Id = 7, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 8, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 9, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
new Article {Id = 10, Title = "Best title one", Content = "Amazing content", AuthorId = 1},
new Article {Id = 11, Title = "Another title", Content = "More amazing content", AuthorId = 1},
new Article {Id = 12, Title = "Unicorns", Content = "Even more amazing content", AuthorId = 1},
};
}
}
public class Program
{
public static void Main()
{
List<Article> articles = new Article().GetArticles();
int pageNumber = 1;
int itemsPerPage = 5;
IEnumerable<Article[]> allPages = articles.Chunk(itemsPerPage);
Console.WriteLine($"Page: {pageNumber}");
foreach(Article article in allPages.ElementAt(pageNumber - 1))
{
Console.WriteLine($" Id: {article.Id}, Title:{article.Title}, Content:{article.Content}, AuthorId:{article.AuthorId}");
}
}
}
There’s nothing wrong with using the first method to do pagination. The Chunk method is a new way of doing achieving the same result but with a cleared code. It takes only one parameter, the maximum size of each chunk (largest numbers of items per page). Is important to note that the Chunk method is only available in .NET 6.
Conclusion
This is another way to do things, another tool in your arsenal so you can use both.