Containerizing and deploying ASP.NET Core applications
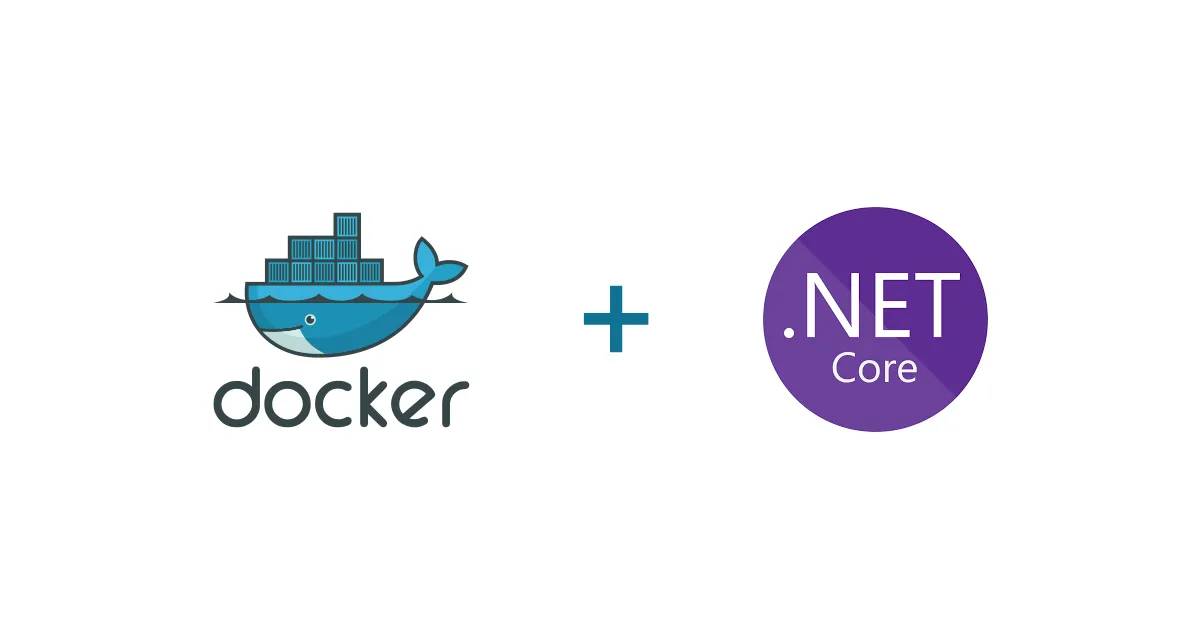
Containerization has transformed the way applications are developed, deployed, and managed in the modern software industry. Docker, in particular, has become a pivotal tool, simplifying the packaging and deployment of applications, including ASP.NET Core applications. In this guide, we’ll dive into the world of containerization and explore how Docker can significantly enhance the development and deployment of ASP.NET Core applications.
The Importance of containerization
Containers have revolutionized application deployment by providing a consistent and portable environment. They encapsulate an application and its dependencies, ensuring consistent performance across various environments, from a developer’s laptop to a production server.
Containers are an efficient and lightweight solution for packaging applications and their components. They encapsulate everything needed to run an application, from libraries and frameworks to system tools, in a single, self-contained unit. This approach simplifies development, deployment, and scaling, making it a crucial tool for modern developers.
The benefits of using Docker for ASP.NET Core applications
Docker has emerged as the standard for creating, managing, and orchestrating containers, particularly for ASP.NET Core developers. Its popularity is due to the significant advantages it offers:
- Consistency: Docker ensures that your ASP.NET Core application runs consistently across different environments, eliminating issues related to varying setups.
- Isolation: Containers provide isolation from the underlying infrastructure, allowing multiple ASP.NET Core applications to coexist without conflicts.
- Scalability: Docker simplifies horizontal scaling, enabling you to run multiple container instances of your ASP.NET Core applications effortlessly.
- Efficiency: Containers are lightweight and resource-efficient, reducing overhead compared to traditional virtualization.
- DevOps Integration: Docker seamlessly integrates into DevOps workflows, enabling smooth CI/CD processes.
In this guide, we’ll walk you through the process of Dockerizing your ASP.NET Core applications, enabling you to harness these benefits and streamline your development and deployment workflows. Whether you’re a seasoned developer or new to ASP.NET Core and Docker, you’ll discover how containerization can empower your projects.
Prerequisites
Before you embark on the journey of Dockerizing your ASP.NET Core application, you need to ensure that your development environment is set up correctly. Here are the prerequisites:
1. Docker: Docker is the foundation for containerization. It allows you to create, manage, and run containers effortlessly.
- Installation: You can download Docker for your specific operating system from the official Docker website:
— [Docker for Windows]
— [Docker for macOS]
— [Docker for Linux]
- Verification: After installation, open a terminal (Command Prompt on Windows, Terminal on macOS/Linux) and run `docker — version` to confirm a successful installation. You should see the Docker version displayed.
2. .NET SDK: Since you’re working with ASP.NET Core, you’ll need the .NET SDK to build and run your ASP.NET Core application.
- Installation: Download and install the .NET SDK from the official .NET website:
— [.NET SDK Downloads]
- Verification: After installation, run `dotnet — version` in your terminal to ensure that the .NET SDK is installed correctly. You should see the installed SDK version.
3. An ASP.NET Core application: You should have an existing ASP.NET Core application that you want to containerize. If you don’t have one yet, you can create a new ASP.NET Core project using the .NET CLI or Visual Studio.
- Creating an ASP.NET Core project: Use the following .NET CLI command to create a new ASP.NET Core web application:
dotnet new web -n MyAspNetCoreApp
Replace `MyAspNetCoreApp` with your preferred project name.
With these prerequisites in place, you’re ready to dive into the world of Docker and ASP.NET Core. In the upcoming sections, we’ll guide you through the process of containerizing your ASP.NET Core application for efficient deployment and scaling.
Getting started with Docker
Docker is a powerful tool that simplifies the process of packaging, distributing, and running applications within isolated environments called containers. Containers provide a consistent and lightweight way to package applications and their dependencies. Let’s dive into the basics of Docker:
What is Docker?
Docker is a containerization platform that allows you to create, deploy, and run applications as self-sufficient containers. These containers package an application’s code, runtime, libraries, and system tools, ensuring that it runs consistently across various environments.
Key Docker concepts:
- Docker Container: A Docker container is a lightweight, standalone executable package that includes everything needed to run an application, including code, runtime, libraries, and system tools. Containers are isolated from each other and from the host system, providing consistency and security.
- Docker Image: A Docker image is a read-only template that contains the instructions for creating a Docker container. Images serve as the blueprint for containers and can be shared and reused.
- Dockerfile: A Dockerfile is a plain-text configuration file that defines the steps for creating a Docker image. It specifies the base image, sets up the environment, copies application code, and configures runtime settings.
The containerization process:
Containerization simplifies the deployment of applications, including ASP.NET Core applications. Here’s a high-level overview of the containerization process:
• Step 1: Create a Dockerfile to containerize your ASP.NET Core application, you start by creating a Dockerfile in your project’s root directory. The Dockerfile contains instructions for building a Docker image.
# Use an official ASP.NET Core runtime as a base image
FROM mcr.microsoft.com/dotnet/aspnet:5.0 AS base
WORKDIR /app
EXPOSE 80
# Use the SDK image to build the application
FROM mcr.microsoft.com/dotnet/sdk:5.0 AS build
WORKDIR /src
COPY ["MyAspNetCoreApp/MyAspNetCoreApp.csproj", "MyAspNetCoreApp/"]
RUN dotnet restore "MyAspNetCoreApp/MyAspNetCoreApp.csproj"
COPY . .
WORKDIR "/src/MyAspNetCoreApp"
RUN dotnet build "MyAspNetCoreApp.csproj" -c Release -o /app/build
# Publish the application
FROM build AS publish
RUN dotnet publish "MyAspNetCoreApp.csproj" -c Release -o /app/publish
# Create the final runtime image
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "MyAspNetCoreApp.dll"]
This Dockerfile uses official ASP.NET Core images to build and run your application.
• Step 2: Build the Docker Image Run the following command in the same directory as your Dockerfile to build the Docker image:
docker build -t my-aspnet-core-app .
Replace my-aspnet-core-app
with a meaningful name for your image.
• Step 3: Run a Docker Container Once the image is built, you can run a Docker container based on that image:
docker run -d -p 80:80 --name my-aspnet-container my-aspnet-core-app
This command starts a container named my-aspnet-container
from the my-aspnet-core-app
image, mapping port 80 from the container to port 80 on your host machine.
Now, your ASP.NET Core application is running in a Docker container, isolated and ready for deployment.
Setting up an ASP.NET Core project
Before you containerize your ASP.NET Core application with Docker, you’ll need to have a working ASP.NET Core project. In this section, we’ll cover how to create a simple ASP.NET Core application and provide an overview of its project structure and dependencies.
Creating a sample ASP.NET Core application:
If you don’t have an existing ASP.NET Core application, you can quickly create a new one using the .NET CLI. Open your terminal or command prompt and follow these steps:
• Step 1: Create a new ASP.NET Core web application
dotnet new web -n MyAspNetCoreApp
This command generates a new ASP.NET Core web application named MyAspNetCoreApp
.
• Step 2: Navigate to the project directory
cd MyAspNetCoreApp
• Step 3: Run the Application
dotnet run
Start the ASP.NET Core application. You should see output indicating that the application is running locally on port 5000 (HTTP) or 5001 (HTTPS).
Project structure and dependencies:
An ASP.NET Core project typically follows a well-defined structure. Here’s a brief overview of the key components you’ll find in your project directory:
- Program.cs: This file contains the entry point of your ASP.NET Core application. It configures the host and sets up the application’s startup.
- Startup.cs: The
Startup
class is where you configure the application's services and middleware. It defines the request processing pipeline.
- Controllers: This folder contains your application’s controllers, which handle incoming HTTP requests and define the application’s behavior.
- Views: In the Views folder, you’ll find Razor views that define the HTML templates for your application’s pages. These views use the Razor syntax for dynamic content rendering.
- wwwroot: The
wwwroot
folder stores static assets such as CSS, JavaScript files, and images.
- appsettings.json: This configuration file holds application settings, such as connection strings and custom configuration values.
- Startup.cs: The
Startup
class is where you configure the application's services and middleware. It defines the request processing pipeline.
- Dependencies: Your ASP.NET Core application may have dependencies on packages and libraries. These dependencies are managed using NuGet, and you can find them listed in the
.csproj
file in your project directory.
Here’s a simple example of a basic ASP.NET Core controller and view:
HomeController.cs:
using Microsoft.AspNetCore.Mvc;
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}
Index.cshtml (in Views/Home):
@{
ViewData["Title"] = "Home Page";
}
<h1>Welcome to ASP.NET Core!</h1>
<p>This is the home page of your ASP.NET Core application.</p>
With your ASP.NET Core application set up, you’re ready to proceed with Dockerizing it in the following sections.
Dockerizing your ASP.NET Core application
Now that you have your ASP.NET Core application up and running, it’s time to containerize it using Docker. This section will guide you through creating a Dockerfile for your application, discussing Dockerfile best practices, and building a Docker image.
Creating a Dockerfile:
A Dockerfile is a text file that contains a set of instructions for building a Docker image. In this case, we’ll create a Dockerfile to package your ASP.NET Core application.
Sample Dockerfile:
# Use an official ASP.NET Core runtime as a base image
FROM mcr.microsoft.com/dotnet/aspnet:5.0 AS base
WORKDIR /app
EXPOSE 80
# Use the SDK image to build the application
FROM mcr.microsoft.com/dotnet/sdk:5.0 AS build
WORKDIR /src
COPY ["MyAspNetCoreApp/MyAspNetCoreApp.csproj", "MyAspNetCoreApp/"]
RUN dotnet restore "MyAspNetCoreApp/MyAspNetCoreApp.csproj"
COPY . .
WORKDIR "/src/MyAspNetCoreApp"
RUN dotnet build "MyAspNetCoreApp.csproj" -c Release -o /app/build
# Publish the application
FROM build AS publish
RUN dotnet publish "MyAspNetCoreApp.csproj" -c Release -o /app/publish
# Create the final runtime image
FROM base AS final
WORKDIR /app
COPY --from=publish /app/publish .
ENTRYPOINT ["dotnet", "MyAspNetCoreApp.dll"]
In this Dockerfile:
- We use official ASP.NET Core images as base images for both building and running the application.
- We copy the ASP.NET Core project files, restore dependencies, build the application, and publish it.
- We create a final runtime image from the base image, copying the published application files and specifying the entry point.
Dockerfile best practices and optimizations:
- Use official images: Whenever possible, use official base images provided by trusted sources like Microsoft. These images are regularly updated and maintained.
- Minimize image size: Keep your Docker images as small as possible. Avoid unnecessary packages and dependencies. Use multi-stage builds, as demonstrated in the Dockerfile, to reduce image size.
- Clean up: Remove unnecessary files and temporary build artifacts in the final image to reduce its size further.
Building a Docker image:
To build a Docker image from your Dockerfile, navigate to the directory containing the Dockerfile and run the following command:
docker build -t my-aspnet-core-app .
Replace my-aspnet-core-app
with a meaningful name for your Docker image. The -t
flag specifies the image name or tag.
This command will execute the instructions in your Dockerfile, creating a Docker image containing your ASP.NET Core application.
Running Docker containers
With your ASP.NET Core application Docker image created, you can now run it as a Docker container. This section will demonstrate how to run a Docker container from the image, cover common Docker commands for managing containers, and show how to access the running ASP.NET Core application within the container.
Running a Docker container:
To run a Docker container based on your ASP.NET Core image, use the docker run
command. Replace my-aspnet-container
with your chosen container name and my-aspnet-core-app
with the image name you provided during the image build:
docker run -d -p 80:80 --name my-aspnet-container my-aspnet-core-app
-d
: Runs the container in detached mode, allowing you to continue using your terminal.
-p 80:80
: Maps port 80 on your host machine to port 80 within the container, enabling you to access the ASP.NET Core application.
--name my-aspnet-container
: Assigns the name my-aspnet-container
to the running container.
Common Docker container commands:
Here are some common Docker commands for managing containers:
• Start a stopped container:
docker start my-aspnet-container
• Stop a running container:
docker stop my-aspnet-container
• Restart a container:
docker restart my-aspnet-container
• View running containers:
docker ps
• View all containers (including stopped):
docker ps -a
• Access the container shell (interactive mode):
docker exec -it my-aspnet-container sh
Accessing the running ASP.NET Core application:
Once your container is running, you can access the ASP.NET Core application within it. Open your web browser and navigate to:
http://localhost:80
You should see your ASP.NET Core application running, just as it would on a traditional web server.
Environment variables and configuration
Configuring your ASP.NET Core application is a crucial aspect of containerization and deployment. In this section, we’ll explore how to use environment variables for configuration, discuss the concept of 12-factor app configuration, and demonstrate how to pass environment variables to Docker containers.
Using environment variables for configuration:
Environment variables are an excellent way to manage configuration settings in your ASP.NET Core application. They offer flexibility and security by allowing you to store sensitive information separate from your code.
In your ASP.NET Core application, you can access environment variables using the IConfiguration
interface. The appsettings.json
file can be extended to read values from environment variables, providing a seamless way to switch between local development and containerized production environments.
Example of reading environment variables in Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
// Add configuration to access appsettings.json and environment variables
IConfigurationBuilder configurationBuilder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json")
.AddEnvironmentVariables(); // Reads environment variables
IConfiguration configuration = configurationBuilder.Build();
// Access configuration values
string connectionString = configuration.GetConnectionString("DefaultConnection");
services.AddDbContext<ApplicationDbContext>(options =>
options.UseSqlServer(connectionString));
// ...
}
The 12-Factor app configuration:
The 12-factor app methodology is a set of best practices for building modern, scalable, and maintainable applications. One of its key principles is the use of environment variables for configuration. This approach decouples configuration from code, making it easier to manage and secure.
Passing environment variables to Docker containers:
To pass environment variables to Docker containers, you can use the -e
flag when running a container with docker run
. For example, to pass a connection string as an environment variable:
docker run -d -p 80:80 --name my-aspnet-container -e ConnectionString="your_connection_string_here" my-aspnet-core-app
Inside your ASP.NET Core application, you can then access this environment variable as shown in the previous example.
Benefits of using environment variables:
- Security: Sensitive information, such as database connection strings and API keys, can be stored securely outside your codebase.
- Portability: Environment variables make it easy to switch between different environments (development, staging, production) without code changes.
- 12-Factor compatibility: Following the 12-factor app configuration guidelines promotes best practices in application development and deployment.
Docker Compose for multi-container applications
As your application grows, you might need to manage multiple containers and services, such as databases, cache stores, and more. Docker Compose is a powerful tool that simplifies the management of multi-container applications. In this section, we’ll introduce Docker Compose, create a Docker Compose file for your ASP.NET Core application and a related database service, and demonstrate how to start and manage multiple containers using Docker Compose.
Introducing Docker compose:
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a declarative YAML file, known as a docker-compose.yml file, to define the services, networks, and volumes required for your application.
Creating a Docker Compose file:
To manage your ASP.NET Core application and a related database service, create a docker-compose.yml
file in your project directory. Below is an example of a simple docker-compose.yml
file that includes an ASP.NET Core service and a SQL Server database service:
Sample docker-compose.yml
file:
version: '3.8'
services:
webapp:
image: my-aspnet-core-app # Use the image you built earlier
ports:
- "80:80"
environment:
- ConnectionString=your_connection_string_here # Use your actual connection string
sqlserver:
image: mcr.microsoft.com/mssql/server:2019-latest
environment:
- SA_PASSWORD=your_password_here # Set your SQL Server password
- ACCEPT_EULA=Y
ports:
- "1433:1433"
In this example:
- The
webapp
service uses the Docker image you built for your ASP.NET Core application.
- The
sqlserver
service uses the official SQL Server image from Microsoft.
- Environment variables are defined to configure your application and database.
Starting and managing containers with Docker Compose:
To start containers defined in your docker-compose.yml
file, navigate to the directory containing the file and run the following command:
docker-compose up -d
The -d
flag runs the containers in detached mode, allowing you to continue using your terminal.
To stop and remove the containers, use the following command:
docker-compose down
Docker Compose simplifies the management of complex multi-container applications. It allows you to define, start, and stop all the services in your application with a single command.
With Docker Compose, you can easily scale your application by adding more services or containers, making it a powerful tool for orchestrating your Dockerized ASP.NET Core applications.
Deploying dockerized ASP.NET Core applications
Deploying Dockerized ASP.NET Core applications allows you to leverage the benefits of containerization for production environments. In this section, we’ll explore deployment options for Dockerized ASP.NET Core applications, including local, cloud, and Kubernetes deployments. We’ll also highlight key considerations for production deployments.
Deployment options:
1. Local Deployment:
For local development and testing, you can deploy Dockerized ASP.NET Core applications on your development machine. Use Docker Compose or Docker commands to manage containers locally.
2. Cloud Deployment:
Cloud platforms like Amazon Web Services (AWS), Microsoft Azure, Google Cloud Platform (GCP), and others offer container orchestration services like Amazon ECS, Azure Kubernetes Service (AKS), and Google Kubernetes Engine (GKE). These services make it easy to deploy and manage Docker containers in the cloud.
3. Kubernetes Deployment:
Kubernetes is a powerful container orchestration platform that can be used for deploying and scaling Dockerized ASP.NET Core applications. You can set up Kubernetes clusters on various cloud providers or on-premises environments.
Considerations for production deployments:
When deploying Dockerized ASP.NET Core applications to production, several considerations are crucial for ensuring a reliable and secure deployment:
- Security: Secure your container images by regularly updating base images and applying security patches. Use secrets management tools for sensitive data like database connection strings and API keys.
- Scaling: Consider how your application will scale in a production environment. Kubernetes, for example, provides auto-scaling capabilities that automatically adjust the number of container replicas based on traffic.
- Monitoring and logging: Implement monitoring and logging solutions to gain insights into the health and performance of your application. Tools like Prometheus and Grafana are popular for containerized applications.
- High availability: Ensure high availability by running multiple replicas of your application behind a load balancer. This minimizes downtime in case of failures.
- Data management: Plan for data storage and management. Use container-friendly databases like PostgreSQL, MySQL, or cloud-based database services.
- Continuous Integration and Continuous Deployment (CI/CD): Set up CI/CD pipelines to automate the building and deployment of Docker containers. Tools like Jenkins, GitLab CI/CD, and Azure DevOps are commonly used for CI/CD with containers.
Example of Kubernetes deployment:
Below is a simplified example of deploying an ASP.NET Core application to Kubernetes using a Kubernetes Deployment resource:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-aspnet-deployment
spec:
replicas: 3 # Adjust the number of replicas as needed
selector:
matchLabels:
app: my-aspnet-app
template:
metadata:
labels:
app: my-aspnet-app
spec:
containers:
- name: my-aspnet-container
image: my-aspnet-core-app:latest # Use your Docker image
ports:
- containerPort: 80
This YAML defines a Kubernetes Deployment that runs three replicas of your ASP.NET Core application behind a service with a load balancer.
Deploying Dockerized ASP.NET Core applications to production environments requires careful planning, but it offers the flexibility and scalability needed to meet the demands of modern applications.
Conclusion
In this comprehensive guide, we’ve explored the world of Dockerizing ASP.NET Core applications and the myriad benefits it brings to the modern development and deployment landscape. Let’s recap the key takeaways:
Key takeaways:
- Containerization with Docker: Docker provides a lightweight, consistent, and isolated environment for your ASP.NET Core applications. Containers package your application, its dependencies, and configuration, ensuring consistent behavior across different environments.
- Dockerfile: A Dockerfile is the blueprint for creating a Docker image. It defines the steps to build an image, set up the environment, and configure runtime settings. Dockerfiles are a crucial part of containerization.
- Environment variables: Leveraging environment variables for configuration allows you to separate sensitive information from your code. This practice enhances security and portability.
- Docker Compose: Docker Compose simplifies the management of multi-container applications. It uses a declarative docker-compose.yml file to define services, networks, and volumes. Docker Compose is invaluable for orchestrating complex setups.
- Deployment options: You have various deployment options, from local development and cloud platforms to Kubernetes clusters. Each option offers its advantages, allowing you to scale and manage your application effectively.
- Production considerations: In production deployments, security, scaling, monitoring, high availability, data management, and CI/CD pipelines are crucial factors to consider. Addressing these aspects ensures a reliable and efficient production environment.
Dockerizing your ASP.NET Core applications is a smart choice for modern development workflows. It streamlines development, simplifies deployments, and enhances scalability, all while maintaining the reliability and security demanded by production environments.
Thank you for joining us on this Dockerization adventure. We hope this guide empowers you to build, ship, and scale ASP.NET Core applications with confidence and efficiency.