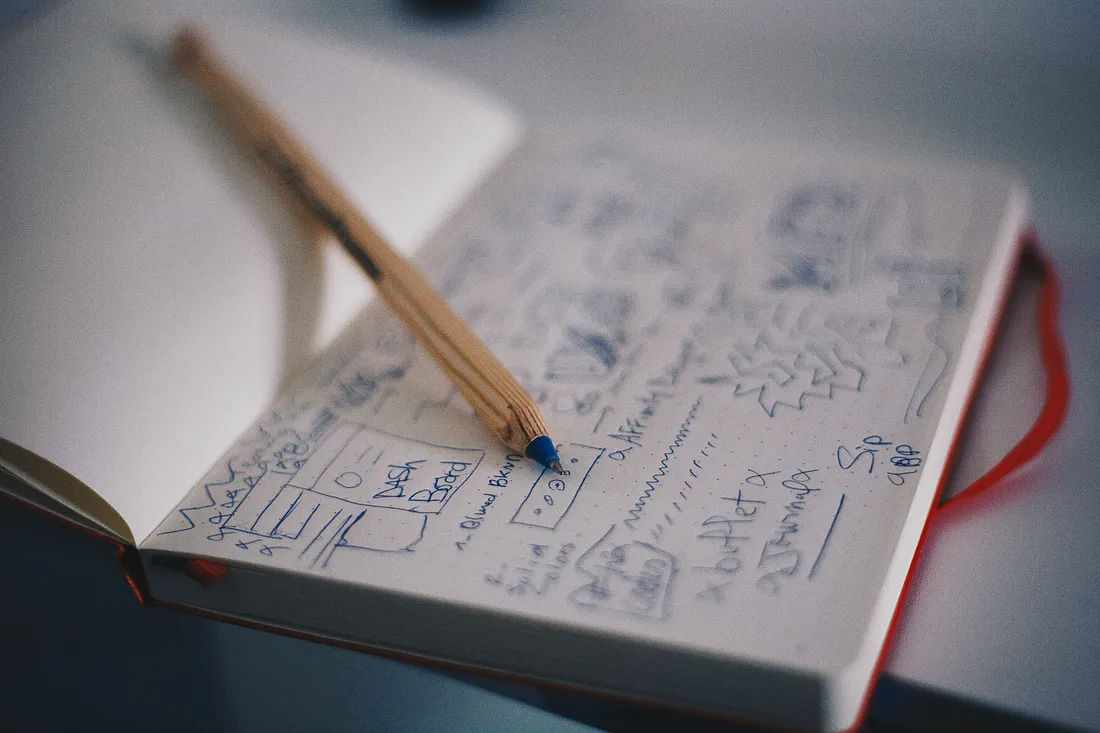
Design patterns are essential for creating maintainable and reusable code in .NET. Whether you’re a seasoned developer or just starting out, understanding and applying these patterns can greatly improve your coding efficiency and overall development process. In this post, we’ll take a look at the most important design patterns in .NET, and provide examples of how they can be implemented.
1. The Singleton Pattern
The Singleton Pattern The singleton pattern is used to ensure that only one instance of a class is created, and that this instance can be accessed globally. A common use case for this pattern is when you need to control access to a shared resource, such as a database connection. Here’s an example of a singleton class in C#:
public class Singleton
{
private static Singleton _instance;
private Singleton() { }
public static Singleton Instance
{
get
{
if (_instance == null)
{
_instance = new Singleton();
}
return _instance;
}
}
}
2. The Factory Pattern
The Factory Pattern The factory pattern is used to create objects without exposing the instantiation logic to the client. This allows for greater flexibility and maintainability, as the client can be decoupled from the specific class being instantiated. Here’s an example of a factory class in C#:
public abstract class Creator
{
public abstract Product FactoryMethod();
}
public class ConcreteCreator : Creator
{
public override Product FactoryMethod()
{
return new ConcreteProduct();
}
}
3. The Observer Pattern
The Observer Pattern The observer pattern is used to create a one-to-many relationship between objects, where one object (the subject) maintains a list of its dependents (the observers) and notifies them of any changes. This pattern is commonly used in event-driven systems, such as GUI applications. Here’s an example of an observer class in C#:
public class Subject
{
private List<Observer> _observers = new List<Observer>();
public void Attach(Observer observer) { _observers.Add(observer); }
public void Detach(Observer observer) { _observers.Remove(observer); }
public void Notify()
{
foreach (Observer observer in _observers)
{
observer.Update();
}
}
}
4. The Decorator Pattern
The decorator pattern is used to add new functionality to an existing object without changing its structure. This pattern is useful when you need to add functionality to a class, but don’t want to create a new class for each combination of functionality. Here’s an example of a decorator class in C#:
public abstract class Component
{
public abstract void Operation();
}
public class ConcreteComponent : Component
{
public override void Operation()
{
// Original functionality
}
}
public abstract class Decorator : Component
{
protected Component _component;
public void SetComponent(Component component)
{
_component = component;
}
public override void Operation()
{
if (_component != null)
{
_component.Operation();
}
}
}
public class ConcreteDecoratorA : Decorator
{
public override void Operation()
{
base.Operation();
// Additional functionality
}
}
5. The Template Method Pattern
The template method pattern is used to define the skeleton of an algorithm, and allow subclasses to fill in the details. This pattern is useful when you have a common algorithm that can be reused, but with different implementation details. Here’s an example of a template method class in C#:
public abstract class AbstractClass
{
public void TemplateMethod()
{
Method1();
Method2();
}
public abstract void Method1();
public virtual void Method2()
{
// Default implementation
}
}
public class ConcreteClass : AbstractClass
{
public override void Method1()
{
// Implementation details
}
}
• • •
These five design patterns are some of the most important and widely used in .NET development. By understanding and implementing these patterns, you can greatly improve the structure and maintainability of your code. Remember to always consider the problem at hand and choose the pattern that best suits it, as using the pattern that doesn’t fit can lead to more complexity rather than solving it.
Additional
The Command Pattern
The Command pattern is used to encapsulate a request as an object, which allows for greater flexibility and separation of concerns. This pattern is useful when you need to handle different requests in a similar way or need to support undo/redo functionality. Here’s an example of a Command class in C#:
public interface ICommand
{
void Execute();
void Undo();
}
public class ConcreteCommand : ICommand
{
private Receiver _receiver;
private string _state;
public ConcreteCommand(Receiver receiver)
{
_receiver = receiver;
}
public void Execute()
{
_state = _receiver.Action();
}
public void Undo()
{
_receiver.Undo(_state);
}
}
The Adapter Pattern
The Adapter pattern is used to adapt an interface of a class to another interface that the client expects. This pattern is useful when you need to integrate with existing systems or frameworks that have a different interface than what you are expecting. Here’s an example of an Adapter class in C#:
public interface ITarget
{
void Request();
}
public class Adaptee
{
public void SpecificRequest()
{
// Original functionality
}
}
public class Adapter : ITarget
{
private Adaptee _adaptee;
public Adapter(Adaptee adaptee)
{
_adaptee = adaptee;
}
public void Request()
{
_adaptee.SpecificRequest();
}
}
Conclusion
In conclusion, understanding and applying design patterns in .NET can greatly improve the structure and maintainability of your code. Whether you’re working on a new project or maintaining an existing one, these patterns can provide a solid foundation for your development process. The Singleton, Factory, Observer, Decorator, Template Method, Command and Adapter patterns are just a few examples of the many design patterns that are available in .NET. By understanding and implementing these patterns, you can write more efficient and organized code, making it easier to add new features, fix bugs and make changes in the future. Always remember that the key to successful implementation of design pattern is to choose the right pattern for the problem at hand.