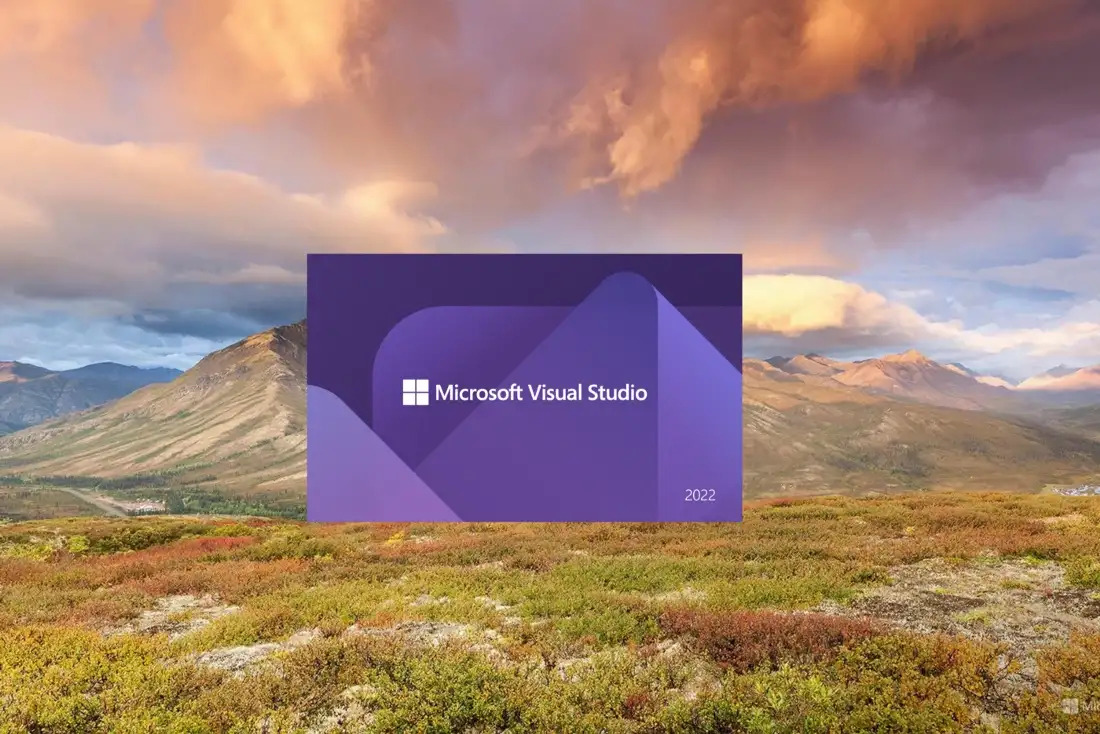
Aha! There is always something intimidating about Dependency Injection. I distinctly remember a couple of years ago, telling a .Net recruiter for a contract project, that,
“I had no idea what DI is”
He immediately stopped talking to me. It’s just one of those moments.
It was true, to an extent. It’s one of those things that you have been doing for years, but, you never knew you were doing it.
Like how you unconsciously woo women in college and accidentally end up with a girlfriend.
Or, when you watch so many English movies since childhood, you are accidentally making yourself an excellent speaker of English, without being aware of it.
Anyway, that’s neither here nor there. Let’s get back to, DI.
As always, the code is available here.
Core Idea
DI gets its name from the final, 5th, enter in SOLID principles. The 5th entry of SOLID says the following.
- High-level modules should not import anything from low-level modules. Both should depend on abstractions (e.g., interfaces).
- Abstractions should not depend on details. Details (concrete implementations) should depend on abstractions.
Step One — Interface and Implementations
Right then. First up, you want to create an interface and and it’s implementation.
public interface ISayMyName
{
public string IAmName();
}
public class SayMyNameOne : ISayMyName
{
public string IAmName()
{
var name = "I am Batman!";
return name;
}
}
public class SayMyNameTwo : ISayMyName
{
public string IAmName()
{
var name = "I am Vengence!";
return name;
}
}
You will notice that I have two implementations. That is on purpose. The whole point of using interfaces is to gain the flexibility of being able to change implementations to prove the decoupling facility provided by DI.
Don’t forget to do some Unit Testing in an xUnit project, whilst you are at it.
Step Two — Inject It
Now, the next step is to inject your interface, along with the implementation. This should happen when your app builds.
This is .Net 6. So, we do this in Program.cs. I am using Scoped way of injecting. There are other ways to inject, which are beyond my knowledge level.
//Ideally, you want to use one of the two implementation.
//Ultimatley, the goal here, of Dependency Injection,
//is the flexibility to change the implementation class
//without affecting the code within.
//use the first implementation
//builder.Services.AddScoped<ISayMyName,SayMyNameOne>();
//use the second implementation
builder.Services.AddScoped<ISayMyName, SayMyNameTwo>();
Hopefully, you will read the comments.
Essentially, I, the developer, the coder, and also you, can ‘switch’ between the two implementations.
This way, you use the implementation you want, and the code, is completely unaware of it, hence, decoupling itself from the implementations, current, past and future.
Step Three — The Constructor! Don’t Forget This
The key step.
Note the assignment that happens in the constructor. Without that, DI would fail.
[Route("api/[controller]")]
[ApiController]
public class NameWithDIController : ControllerBase
{
ISayMyName _sayMyName;
//this is the key part
//this is where, the interface is married to the implementation class
//as provided by the startup settings in Program.cs
public NameWithDIController(ISayMyName sayMyName)
{
_sayMyName = sayMyName;
}
[HttpGet]
[Route("SayMyName")]
public ActionResult<string> GetName()
{
var response = _sayMyName.IAmName();
return response;
}
}
Final Note
Of course, this is the most simplest case of doing DI. It may not even be the most efficient way of doing it.
But, it works. Hope it helps you.