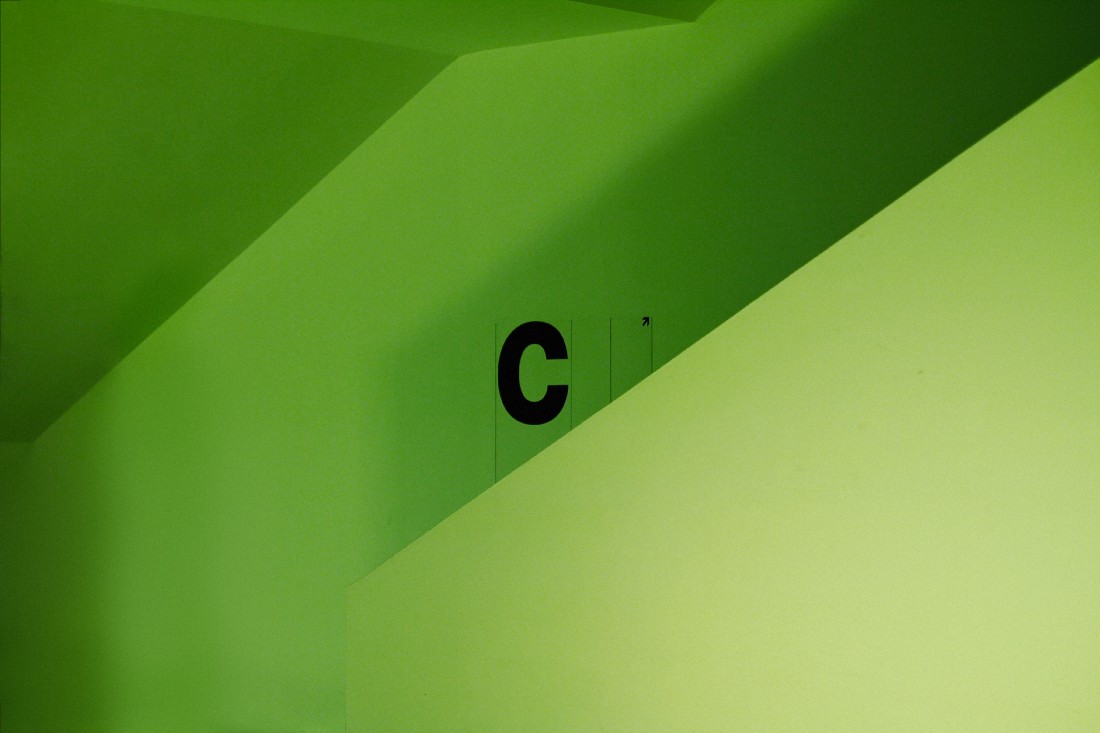
This article will look at the differences between static and readonly C# fields. Basically, a static readonly field can never be replaced by an instance of a reference type. In contrast, a const constant object cannot be replaced by a new one. You will learn what the difference is and how to use inline initialization with readonly fields. Ultimately, this article will help you write better code.
Readonly prevents a field from being replaced by a different instance of the reference type.
The readonly modifier can be used to restrict the use of a variable or object to a single reader and write. The readonly keyword restricts assignment to a value type to the constructor and the class level. When used, readonly prevents the replacement of a reference type field. This keyword will prevent any attempt to modify a field’s value, including changing its name.
The readonly keyword is not supported on array fields. When an array field contains read-only values, it violates the CA2105 rule. To fix the issue, remove the read-only modifier from the field. Immutable types are generally not impacted by breaking changes, so these are fine. If a change is needed to a variable, make sure to make the change in the constructor.
In C#, the const keyword declares a constant variable. It should be initialized at the time of declaration. The readonly keyword can be used on reference types. Its default value is null. The readonly keyword prevents the replacement of a field by a different instance of the reference type. However, the const keyword is not recommended.
You can also use a readonly keyword on computed properties. This will prevent other people from changing the value of the variable. Readonly properties don’t have setters. Instead, they have getters. This means that a value can’t be changed without changing the descriptor. In addition, you can’t change a computed property’s value. The var keyword is used to declare variables rather than constant properties.
When the time of the event has passed, it can be a boolean. This value indicates whether the event is recurring or one-time only. The event is recurring and has an organizer. The organizer object can be moved around. The organizer object is read-only. It doesn’t propagate events. While the organizer object can be changed, the event will still be a single-time occurrence.
The readonly modifier should be avoided when using stored type properties. It ensures that a field won’t be replaced by another instance of the reference type. By default, stored type properties are initialized only on the first access, so they won’t need the lazy modifier. The types for stored type properties include global and computed variables. If you want to write a type property in Swift, you should use the same syntax as for stored-type properties.
Const prevents a constant object from being replaced
When using the const keyword in your code, you are ensuring that the data referenced by a constant object will never change. This ensures that you can make performance optimizations without harming the data. Const references are especially useful when calling functions that take objects by value. This prevents changes to the referenced data, preventing the program from compiling. Read on to learn how to use the const keyword in C#.
In C#, a const reference can never change the value it refers to. However, a const pointer can be replaced. Because of this, it’s crucial to remember that you can’t change a const reference or a const pointer unless you explicitly make it a constant. In many cases, you’ll find yourself changing a const variable and getting rid of the problem!
Another way to avoid changing the value of a constant object is to use a const keyword. It’s a simple way to avoid silly programming mistakes. For example, you might set a field as a test, but then never use it again — this is a problem. In addition, you won’t want to change the name of the constant object! In these situations, a const variable should not be replaced by another object.
When using const references, you’ll want to mark their data members with the keyword &. This will eliminate a temporary clone object and optimize performance. In addition, the const method will invoke the copy constructor, which makes a copy of the data member for later requests. Const is a great way to ensure that data integrity is maintained. It makes it much easier for developers to debug problems and improve the quality of their code.
When using const, you must make sure that the data referenced by the constant object is persistent. In other words, if the data reference is part of the class’s interface, you should make sure that it is a field of the class. You should avoid making reference to local data in functions because they will be invalid references to the data. A const reference should be part of the class’s interface.
Difference between readonly and const
The first thing to understand is the difference between the readonly and const keywords. Constants are absolute fixed values; read-only variables are variable values derived from a user input or a configuration file. Constants are initialized at compile time, while readonly variables are not. Constant fields and read-only variables both allow for additional scenarios during construction, but cannot be changed after.
While the two words sound similar, the main difference between them is how they are used. Const fields are literal values burned into the code, while readonly variables are references. When accessing the values of a read-only field, it must perform a lookup. A static readonly field is a reference, and must be initialized in the constructor. Because of the difference between the two types, they are not always used in the same way.
Const variables should always have values assigned at the time of their declaration. Constants can be any basic data type. Constant values are not mutable and cannot change throughout the program. Constants must be initialized before use. Readonly fields, on the other hand, can be initialized only at the class level. They cannot be modified after initialization. Readonly variables can be initialized inside the constructor or in a class.
The main difference between readonly and const in C# is the way they create read-only variables. Read-only variables are static and cannot be modified once they are initialized. In addition, read-only variables are not initialized at the time of declaration. Instead, they can be initialized inside the constructor. The value of read-only variables cannot be changed once it has been declared. Therefore, read-only variables are often used when you need to assign a value to a class.
Inline initialization for readonly
Inline initialization for readonly in C.NET is an important feature to consider if you use non-constant fields in your classes. This feature prevents you from causing an IL code explosion and is particularly helpful when using structs. The implicit parameter-less constructor of C# provides an easy way to convert inline initializers to parameter-less constructors. This article looks at the advantages and disadvantages of inline initialization for readonly.
Inline initialization for readonly is a better approach than using constants or constant-valued values. Consts are absolute fixed values, whereas read-only variables are variables that come from user input, configuration files, or another variable. Consts are compile-time constants, while read-only variables are runtime constants that can change. ReadOnly constants are easier to use than const, but you’ll need to remember that they’re only available in classes, not within individual methods.
Readonly fields can only be assigned once at the time of declaration. You can’t reassign them again after the constructor exits. Fortunately, C# allows readonly field references to be declared outside of the constructor. If you need to assign them multiple times, consider using the ReadOnlyCollection type. It wraps other collections and prevents writing. But be sure to use it cautiously.
Readonly variables are the best way to declare an array with immutability. You don’t have to worry about modifying the name of the variable. All you need to do is call prepared functions for storing the values of readonly variables. It’s that simple! The benefits of using inline initialization for readonly variables are well worth the effort. You can use the prepared functions to retrieve the length of an array in C#.
Const variables can be declared with the const keyword. Const variables can be accessed using ClassName.StaticMemberName or ClassName.Readonly members can only be accessed through an object. But a readonly variable can be accessed using the object, but its value can’t be changed in the method. That’s one of the benefits of readonly variables. But there are some drawbacks to using them.