What Is Caching?
Caching is a performance optimization strategy and design consideration. Caching can significantly improve app performance by making infrequently changing (or expensive to retrieve) data more readily available.
Why Caching?
To eliminate the need to send requests towards the API in many cases and also to send full responses in other cases.
Some Caching Benefits?
- Reduction in network round trips by making infrequently changing (or expensive to retrieve) data more readily available.
- Reduction in network bandwidth for making database calls or queries.
- Boosts application performance and enhance user experience.
Some Cache Types?
There are three types of caches:
— Client Cache
Client cache lives on the client (browser); thus, it is a private cache. It is private because it is related to a single client.
— Gateway Cache
The gateway cache lives on the server and is a shared cache. This cache is shared because the resources it caches are shared over different clients.
— Proxy Cache
The proxy cache is also a shared cache, but it doesn’t live on the server nor the client side. It lives on the network.
Now that We have seen reasons why we should start implementing Cache in our app, Let’s take a look at some Caching Implementation in .NET.
Note: We need a sample API.
(In Our case, We’ll be using an already created API because this tutorial is about caching)
You can download source code here https://github.com/JiboGithub/StudentTeacherAPI.git
This tutorial puts in assumption that you are familiar and comfortable developing Web APIs with .Net Core.
Let’s continue if you didn’t enter the if statement.
Response Caching In .NET
Response caching reduces the number of requests a client or proxy makes to a web server. Response caching also reduces the amount of work the web server performs to generate a response. Response caching is set in headers.
Note: Disable caching for content that contains information for authenticated clients. Caching should only be enabled for content that doesn’t change based on a user’s identity or whether a user is signed in.
See why → https://docs.microsoft.com/en-us/aspnet/core/performance/caching/middleware?view=aspnetcore-6.0
Let’s Get Started……………………………..
Add on GetTeachersController API method like this:
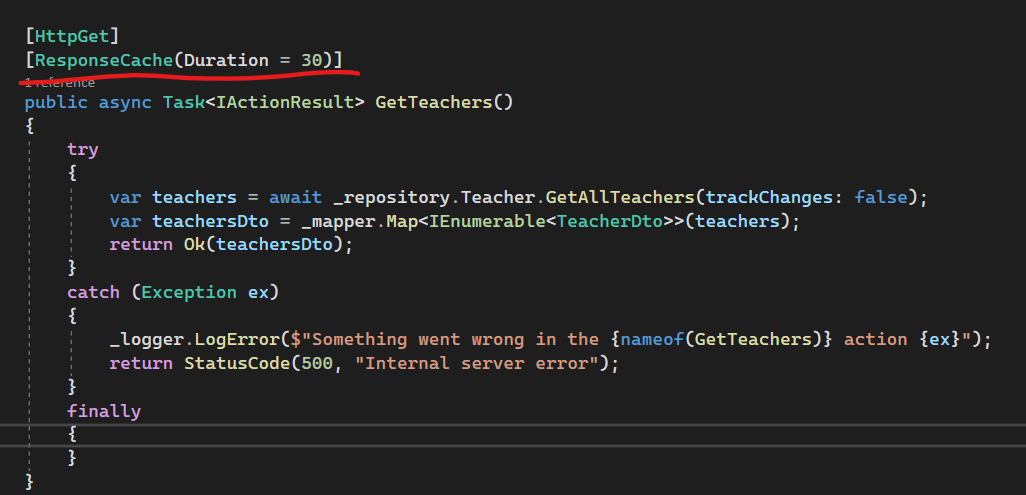
Adding Response Cache to API
Run App to and make the API request. Navigate to headers and inspect the cache-control header.
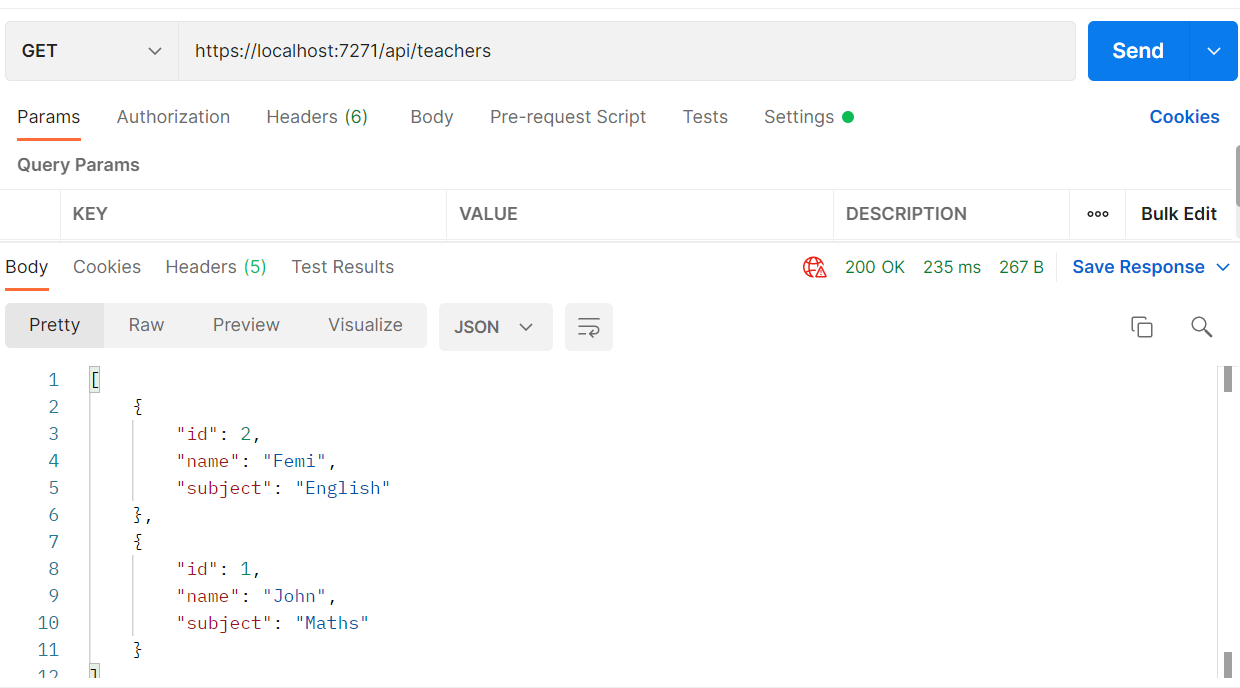
Running GetTeachers API
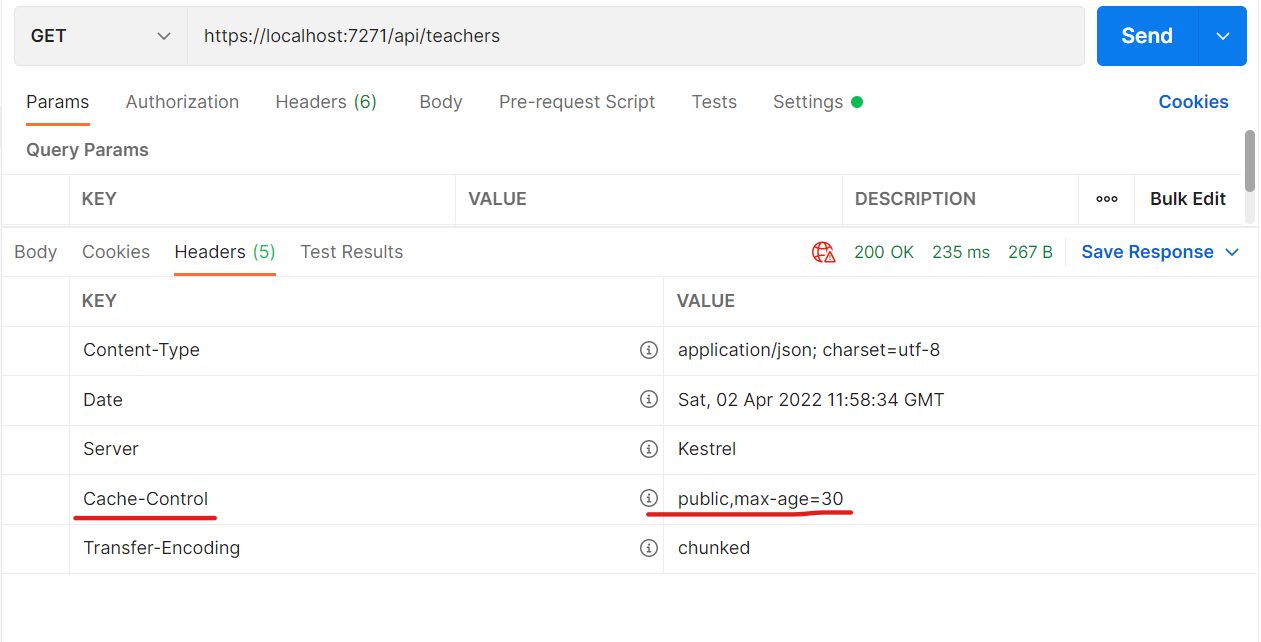
Here the Cache-Control header was created with a public cache and set to a duration of 30 seconds.
Note: This is just a header; The response is not stored yet…
So we need a cache-store to cache the response.
Now we are going to add that next…
Navigate to ServiceCollectionExtension class in Extensions folder located in StudentTeacher main API project.
Add the method ConfigureResponseCaching:

Add in ServiceCollectionExtension.cs

Add in Program.cs

Add below app.UseHttpsDirection in Program.cs
Let’s run it on Postman……….
Now let’s run the app and call the GetTeachers API which we have placed a cache attribute on.
This time we are going to send the API request twice. The first response will be the actual query that fetches the records from our database. The record gets cached for 30 seconds, then the second time we send that same request, we get the response from the cache store as far as the cached duration has not elapsed.
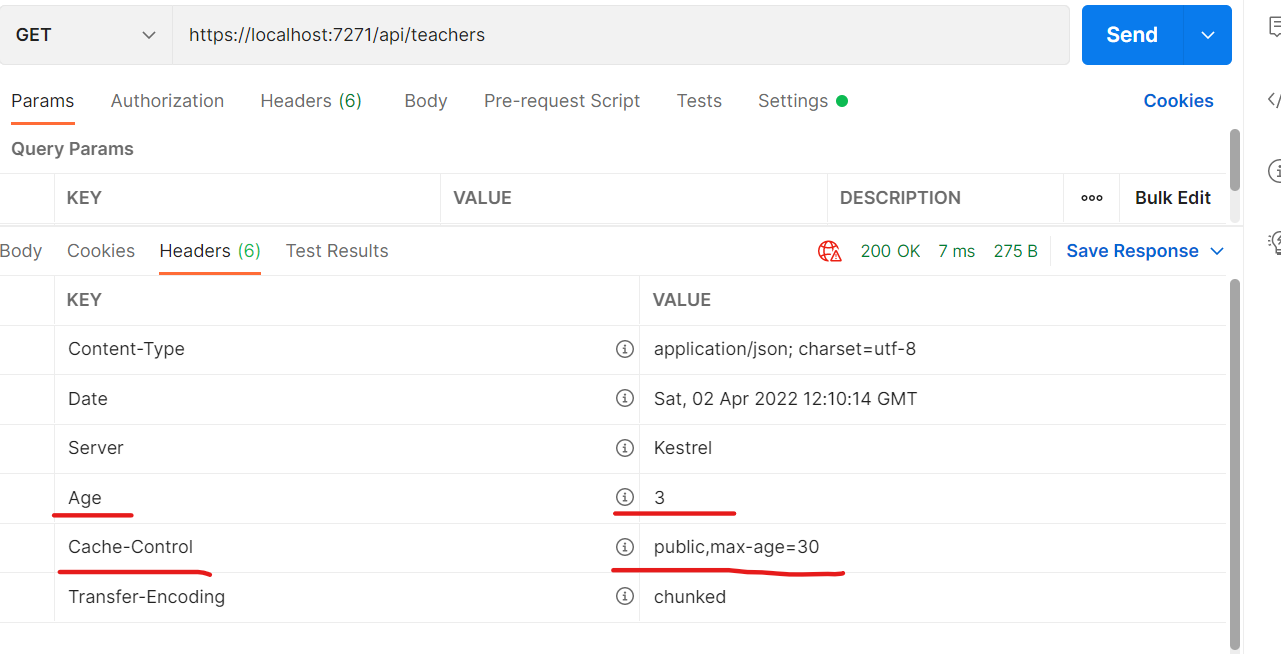
Getting Cached Response
We can now see there is an additional Age header with value of 3 which indicates that the number of seconds the object was stored in the cache.
Now that the second response was retrieved from the cache-store.
After the expiration period passes, the response will be sent from the API, and cached again.
Wait for the 30 seconds to elapse, send the API request again and notice that the Age header value will disappear, so when you send the request again before the expiration time, then the Age header appears again bearing the value of seconds the response has been cached.
Using Cache Profiles
Cache profiles can be used for same implementation. This can improve code readability and assist in having well-defined caches in your .NET application.
Add the below method in ServiceCollectionExtension.cs
public static void ConfigureControllers(this IServiceCollection services)
{
services.AddControllers(config => { config.CacheProfiles.Add(“30SecondsCaching”, new CacheProfile{Duration = 30 }); });
}
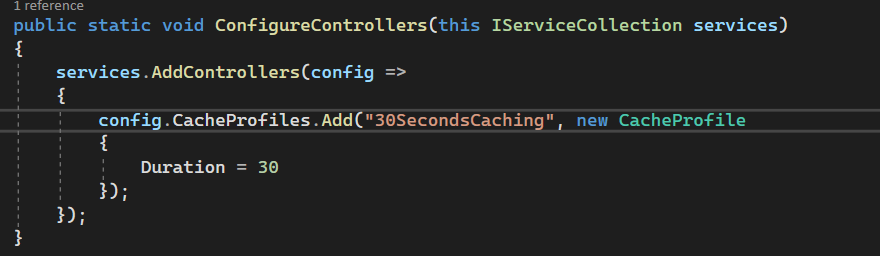
Extension service update
Go to Program.cs file and change builder.Services.AddControllers(); to the new extension method builder.Services.ConfigureControllers();

Go to TeachersController and update the Attribute for GetTeachers method
OLD ←
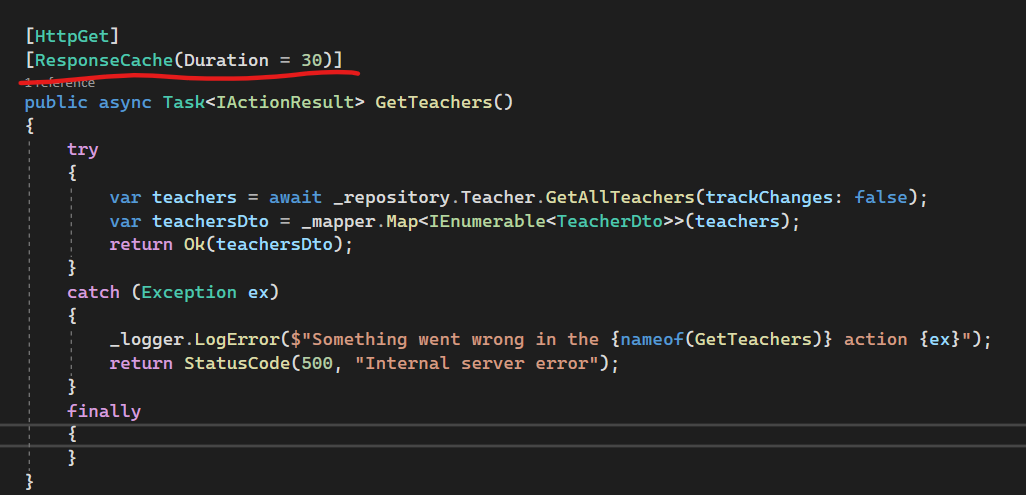
NEW →
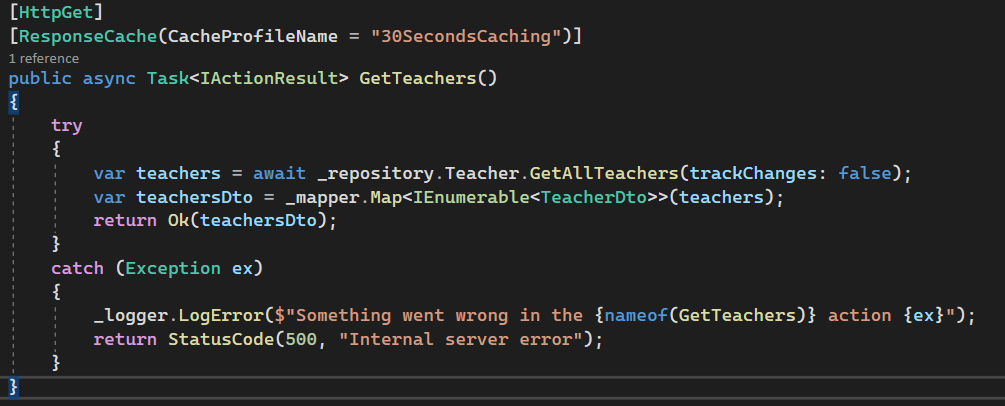
Run the App again and it should work the same.
Caching Consideration
Before Implementing Caching in your app, You need to be sure what type of response you want to cache
Since most times, we deal with records fetched from a database, we want to make sure such records are not the type that change constantly. if so, we need to implement the appropriate expiration time against such to avoid sending outdated data to the client.
For example
Imagine a scenario where records that are gotten from a database changes every 10 seconds or its dynamic but changes fast, you have implemented a 1 minute caching on such record. You risk sending old data to the client and that becomes a problem.
Also there are cases where the data may not change at all or persists over a long period of time, then there is little or no risk to such caching implementation. i.e an API that fetches all countries in the world or all states in country.
mostly static data enjoy a great benefit from caching.
While the Response Caching is easy and straightforward to implement, Due to some of its limitation of not working for Authenticated requests.
You can instead explore other Caching options that best suits your needs here → Caching in .NET | Microsoft Docs and also find out new discoveries and usage.
Link to updated source code → JiboGithub/StudentTeacherAPI
I hope you found this useful.