Background tasks are crucial in many software applications, and scheduling them to run periodically or at specific times is a common requirement. In the .NET ecosystem, Quartz.NET provides a robust and flexible framework for scheduling such tasks. Let’s delve into what Quartz.NET is, why it is useful, and how we can effectively use it to schedule background jobs.
Introduction to Quartz.NET
Quartz.NET is a full-featured, open-source job scheduling system that can be integrated with, or used alongside, virtually any .NET application. It was ported from the popular Java library, Quartz, which has a long history in the Java community.
Quartz.NET allows developers to schedule jobs using a variety of methods, with jobs set to occur at specific times, intervals, or cron expressions. Additionally, Quartz.NET includes built-in support for job clustering and persistence.
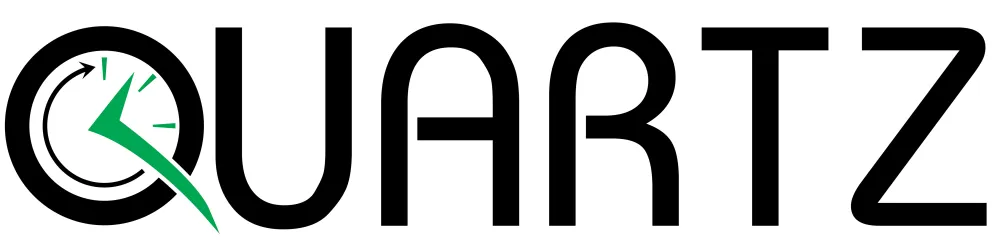
Setting Up Quartz.NET
To use Quartz.NET, first install it via the NuGet package manager. In the package manager console, type:
Install-Package Quartz
Creating a Job
After installing Quartz.NET, the next step is to create a job. Jobs in Quartz.NET are simply classes that implement the IJob interface, which includes a single method, Execute.
Here’s an example of a simple job:
public class HelloWorldJob : IJob
{
public Task Execute(IJobExecutionContext context)
{
Console.WriteLine("Hello, Quartz.NET!");
return Task.CompletedTask;
}
}
This job writes a greeting to the console.
Scheduling a Job
Scheduling a job in Quartz.NET involves a few steps. Here’s an example of how to schedule the HelloWorldJob
to run every 5 seconds:
Scheduling a job in Quartz.NET involves a few steps. Here’s an example of how to schedule the HelloWorldJob to run every 5 seconds:
- Create a
StdSchedulerFactory
and start it:
ISchedulerFactory schedulerFactory = new StdSchedulerFactory();
IScheduler scheduler = await schedulerFactory.GetScheduler();
await scheduler.Start();
- Create a
JobDetail
instance:
IJobDetail job = JobBuilder.Create<HelloWorldJob>()
.WithIdentity("HelloWorldJob", "Group1")
.Build();
- Create a
Trigger
instance:
ITrigger trigger = TriggerBuilder.Create()
.WithIdentity("Trigger1", "Group1")
.StartNow()
.WithSimpleSchedule(x => x
.WithIntervalInSeconds(5)
.RepeatForever())
.Build();
- Schedule the job with the trigger:
await scheduler.ScheduleJob(job, trigger);
Cron Schedules
While simple schedules are sufficient for many tasks, Quartz.NET also supports cron-like schedule expressions for complex schedules.
Here’s an example of a cron schedule that fires every weekday at 9:30 AM:
ITrigger trigger = TriggerBuilder.Create()
.WithIdentity("Trigger2", "Group1")
.WithCronSchedule("0 30 9 ? * MON-FRI")
.Build();
Persisting Jobs
Quartz.NET allows jobs to be persisted, which means they survive application restarts. To configure a persisted job, you must configure Quartz.NET to use a job store. Here’s an example of how to use a JDBC job store:
NameValueCollection props = new NameValueCollection
{
{ "quartz.serializer.type", "binary" },
{ "quartz.jobStore.type", "Quartz.Impl.AdoJobStore.JobStoreTX" },
{ "quartz.jobStore.dataSource", "default" },
{ "quartz.jobStore.tablePrefix", "QRTZ_" },
{ "quartz.dataSource.default.connectionString", "{YourConnectionString}" },
{ "quartz.dataSource.default.provider", "SqlServer" }
};
ISchedulerFactory schedulerFactory = new StdSchedulerFactory(props);
Remember to replace {YourConnectionString}
with your actual database connection string.
Conclusion
Quartz.NET is a powerful, flexible, and feature-rich library for managing and scheduling background jobs in .NET applications. Whether you need to run simple recurring tasks or complex schedules that adhere to cron-like expressions, Quartz.NET has got you covered. It is a robust solution for enterprises with heavy job loads and it also caters to the needs of smaller applications. Moreover, with its ability to persist jobs, it ensures that your scheduled tasks survive application restarts, providing you with reliable and consistent performance. As a .NET developer, mastering Quartz.NET can prove to be a significant enhancement to your toolset. Happy coding!