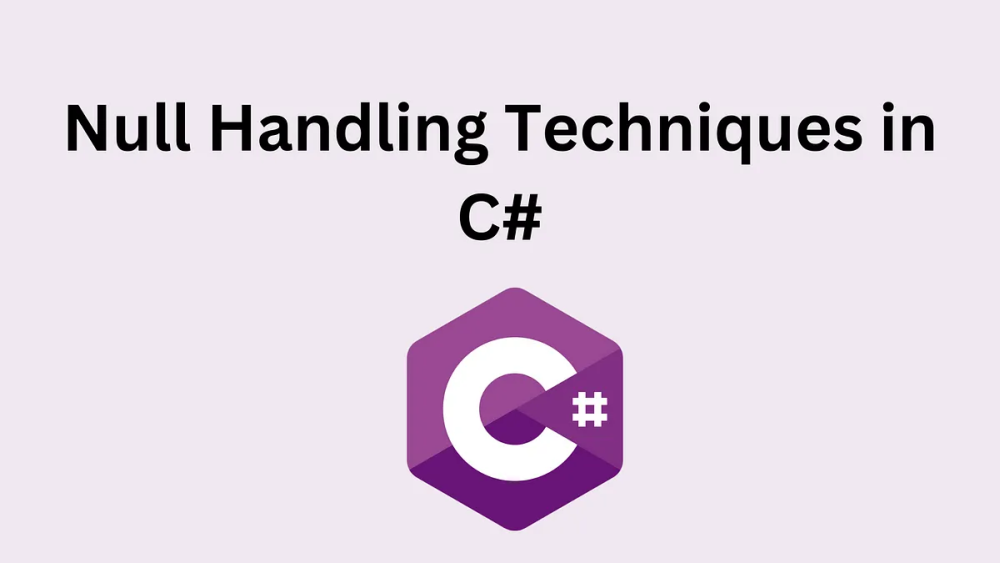
In C# applications, null reference exceptions are frequently the cause of problems and runtime failures. Appropriate null checks are necessary to protect your code from these kinds of problems. This article will examine many approaches to doing null checks in C#, go over the recommended approach, and provide examples to show how to use each one.
1. Traditional null check
The most straightforward way to check for null is through an explicit comparison. For instance:
if (variable != null)
{
// Do something with variable
}
Use the is not null
operator to check for null values. The is not null
operator is a safe way to check for null values, even if the not equality operator (!= null
) has been overridden.
if (variable is not null)
{
// Do something with variable
}
If you are writing code that needs to be compatible with older versions of C#, you should use != null
, because the is not null
operator was not introduced until C# 9.
Here are some examples of when to use is not null
and != null
:
// Use `is not null` if you are working with code that you do not control.
object obj = GetObjectFromSomewhere();
if (obj is not null)
{
// Do something with the object.
}
// Use `!= null` if you are working with code that you control, and you know that the equality operator has not been overloaded.
string name = GetName();
if (name != null)
{
// Do something with the name.
}
// Use `!= null` if you need to be compatible with older versions of C#.
int? number = GetNumber();
if (number != null)
{
// Do something with the number.
}
While this method is simple and widely used, it can be error-prone, especially in large codebases where developers might forget to include null checks, leading to unexpected crashes.
2. Conditional Access Operator (?.)
Introduced in C# 6.0, the conditional access operator is a powerful tool that can help you to write more concise and robust code. By using the conditional access operator, you can avoid having to write explicit null checks for every reference type that you access. For example:
// Access a member of a class.
Person person = GetPerson();
string name = person?.Name;
// Access a member of a struct.
DateTime date = GetDate();
int day = date?.Day;
// Access a member of an interface.
IEnumerable<int> numbers = GetNumbers();
int firstNumber = numbers?.First();
// Access a member of a nullable reference type.
int? number = GetNumber();
int value = number?.Value ?? -1;
Here are some of the benefits of using the conditional access operator:
It makes your code more concise and readable.
It helps to prevent null reference exceptions.
It makes your code more robust and reliable.
3. Null Coalescing Operator (??)
The null coalescing operator (??) in C# is a binary operator that returns its left-hand operand if it is not null, and otherwise returns its right-hand operand.
The syntax for the null coalescing operator is as follows:
int result = leftOperand ?? rightOperand;
where leftOperand
and rightOperand
are expressions of any type.
If the leftOperand
evaluates to null, the null coalescing operator returns the rightOperand
. Otherwise, the null coalescing operator returns the leftOperand
.
The null coalescing operator can be used to provide a default value for an expression, even if the expression evaluates to null. This can be useful for avoiding null reference exceptions and for writing more concise and readable code.
Here are some examples of how to use the null coalescing operator:
// Provide a default value for a variable.
string name = person?.Name ?? "Unknown";
// Get the first element of a collection, or return null if the collection is empty.
int firstNumber = numbers?.First() ?? 0;
// Get the length of a string, or return 0 if the string is null.
int length = string?.Length ?? 0;
4. Guard Clauses
Guard clauses involve checking for null at the beginning of a method and exiting early if the condition is met. This approach can enhance code readability and maintainability. Example:
public void SomeMethod(string variable)
{
if (variable == null)
{
throw new ArgumentNullException(nameof(variable));
}
// Rest of the method logic
}
Guard clauses are especially helpful in preventing downstream issues by catching null references at the entry point of a method.
Preferred Way: Guard Clauses
Among these methods, guard clauses are often considered the preferred way to perform null checks in C#. Here’s why:
1. Early Detection
Guard clauses catch null references at the earliest point in a method, preventing further execution and reducing the likelihood of downstream issues.
2. Code Readability
Explicitly throwing an exception in a guard clause makes the code more readable. It clearly communicates that a null value is not acceptable for the given context.
3. Consistency
Guard clauses enforce a consistent approach to null checks across methods, ensuring that developers adhere to a standardized practice.
Importance of Null Checks
Performing robust null checks is crucial for the following reasons:
1. Preventing Null Reference Exceptions
Null reference exceptions can lead to application crashes and unpredictable behavior. Robust null checks help prevent these issues by catching null references early.
2. Enhancing Code Reliability
Well-executed null checks contribute to the overall reliability of the codebase, reducing the likelihood of bugs and improving the robustness of the application.
3. Improving Maintainability
Code that includes explicit null checks is more maintainable. It helps developers quickly understand the expectations for variable values, making future modifications and debugging easier.
Conclusion
Although there are other approaches, the best one is to employ guard clauses because of their consistency, readability, and early detection capabilities. Developers can make their applications more resistant to null reference exceptions by adopting these best practices, which will result in a software system that is more reliable and predictable.
Keep learning….! 😊