Dependency injection is a powerful technique in software development that promotes loose coupling between components and improves testability and maintainability. When working with the HttpClient library in C#, integrating it with dependency injection can lead to cleaner and more manageable code. In this article, we will explore how to use dependency injection with HttpClient and provide a comprehensive guide with code examples.
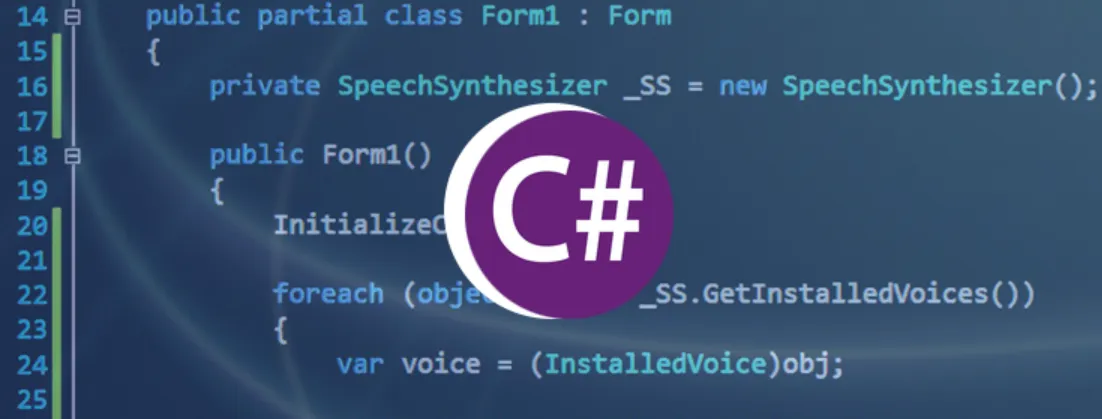
Introduction to Dependency Injection
Dependency injection is a design pattern that allows us to inject dependencies, such as services or objects, into a class rather than creating them within the class. This approach promotes decoupling, making it easier to change or extend the behavior of a component without modifying its code.
In the context of HttpClient, we can inject an instance of HttpClient into our classes or services rather than instantiating it directly. This not only simplifies unit testing but also enables centralized configuration and management of HttpClient instances.
Setting up Dependency Injection
To get started with dependency injection in C#, you need a dependency injection container. In this article, we’ll use the built-in .NET Core Dependency Injection container. Here’s how to set it up:
1. Create a new .NET Core Console Application or use an existing project.
2. Add the necessary NuGet package for dependency injection:
dotnet add package Microsoft.Extensions.DependencyInjection
3. Set up the dependency injection container in the Startup.cs file:
using Microsoft.Extensions.DependencyInjection;
class Program
{
static void Main()
{
var serviceProvider = new ServiceCollection()
.AddHttpClient<MyHttpClient>() // Register the HttpClient as a service
.BuildServiceProvider();
var myHttpClient = serviceProvider.GetRequiredService<MyHttpClient>();
myHttpClient.MakeRequest();
}
}
using Microsoft.Extensions.DependencyInjection;
class Program
{
static void Main()
{
var serviceProvider = new ServiceCollection()
.AddHttpClient<MyHttpClient>() // Register the HttpClient as a service
.BuildServiceProvider();
var myHttpClient = serviceProvider.GetRequiredService<MyHttpClient>();
myHttpClient.MakeRequest();
}
}
In this example, we register an instance of HttpClient as a service using the AddHttpClient
method. We also define a class called MyHttpClient
, which we will use to demonstrate how to inject HttpClient into a service.
Injecting HttpClient into a service
Now that we have registered HttpClient as a service, let’s inject it into a custom service class.
using System;
using System.Net.Http;
using System.Threading.Tasks;
public class MyHttpClient
{
private readonly HttpClient _httpClient;
public MyHttpClient(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task MakeRequest()
{
HttpResponseMessage response = await _httpClient.GetAsync("https://api.example.com/data");
if (response.IsSuccessStatusCode)
{
string content = await response.Content.ReadAsStringAsync();
Console.WriteLine("Response Content: " + content);
}
else
{
Console.WriteLine("Error: " + response.StatusCode);
}
}
}
In this code, we inject an instance of HttpClient into the MyHttpClient
class through its constructor. This allows us to use HttpClient within the class to make HTTP requests.
Benefits of Dependency Injection
Using dependency injection with HttpClient offers several benefits:
- Testability: It becomes easy to write unit tests for your classes because you can provide mock HttpClient instances during testing.
- Centralized Configuration: You can configure HttpClient settings, such as base addresses and default headers, in one place and reuse the configured instance across your application.
- Decoupling: By injecting HttpClient as a dependency, you achieve a more decoupled design, making your codebase easier to maintain and extend.
- Resource Management: The dependency injection container handles the lifecycle of HttpClient instances, ensuring efficient resource management and reuse.
Conclusion
In this article, we’ve explored the concept of dependency injection and demonstrated how to integrate HttpClient with dependency injection in C#. By using the built-in .NET Core Dependency Injection container, we registered HttpClient as a service and injected it into a custom class.
Using dependency injection with HttpClient not only simplifies testing but also promotes maintainable and decoupled code. It’s a valuable technique for building robust and scalable applications that interact with web resources.
As you continue to work with HttpClient and dependency injection, consider applying best practices such as proper exception handling, managing configuration, and handling transient errors for a more resilient application. Happy coding!