Clean Architecture in practice.
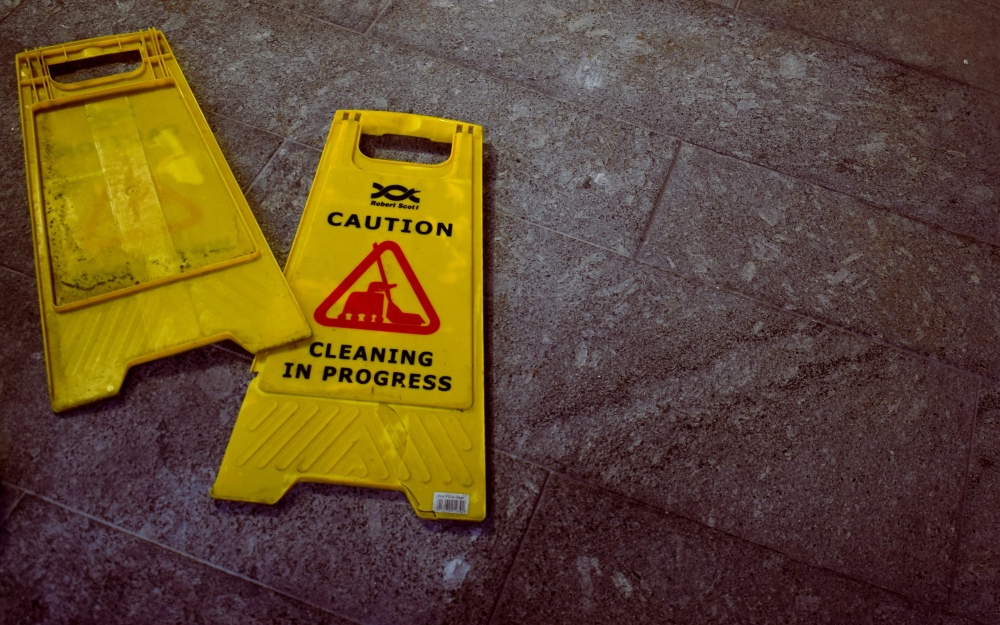
Clean Architecture is a popular approach to building software applications.
A Clean Architecture defines clear boundaries between different application layers, resulting in improved maintainability.
In addition, Clean Architecture approach aims to keep the business logic independent of any specific external frameworks or libraries, making it easier to migrate to new technologies.
In this article, we will look at how to structure a .NET project using Clean Architecture principles.
• • •
The Clean Architecture approach divides the solution into four main layers:
- Domain (core logic)
- Application (application use cases)
- Infrastructure (data access, caching…)
- Presentation (public API)
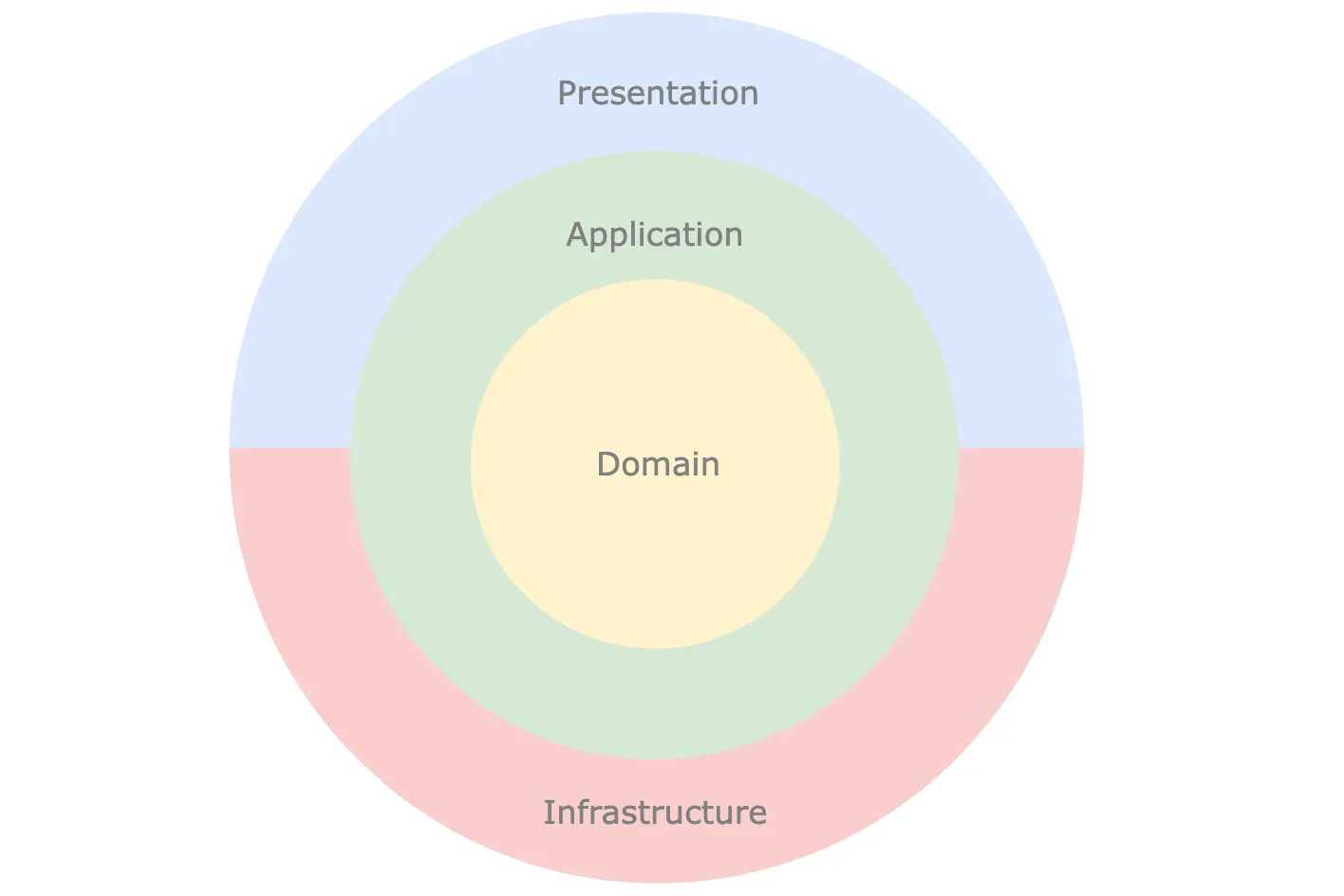
How do these layers depend on each other?
An important rule about dependencies between layers is that any inner circle must not know anything about the outer circles.
Therefore, dependencies between layers can only be directed inward.
Implementation of Clean Architecture
How to implement a clean architecture in a .NET project?
The easiest way is to create a separate project for each of the four layers shown in the diagram.
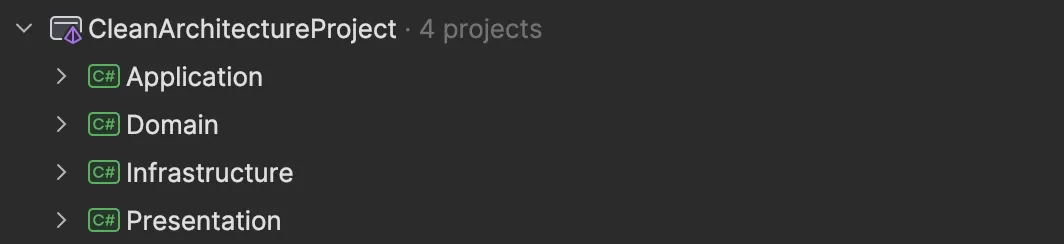
However, a little later in the article, we will adjust this approach a bit.
Now let’s talk about each layer individually.
Domain Layer
The domain layer is where the domain logic of the application is defined.
This layer contains things like entities, value objects, domain services, domain exceptions, domain events, repository interfaces, and so on.
Important thing is that the domain layer does not depend on any other layer. It depends only on .NET primitive types (int
, string
, etc).
When it comes to grouping files into folders within a domain project, there are two common ways to do this.
The first option would be to group files by their type:
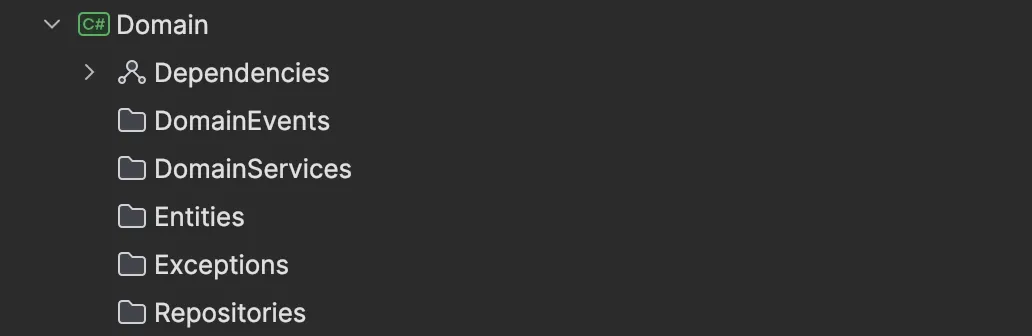
All entities are placed in the Entities
folder, all exceptions are placed in the Exception
folder, and so on.
Another approach is to use entity folders.
For example, if the application contains domain logic related to users and orders, the file structure might look like this:
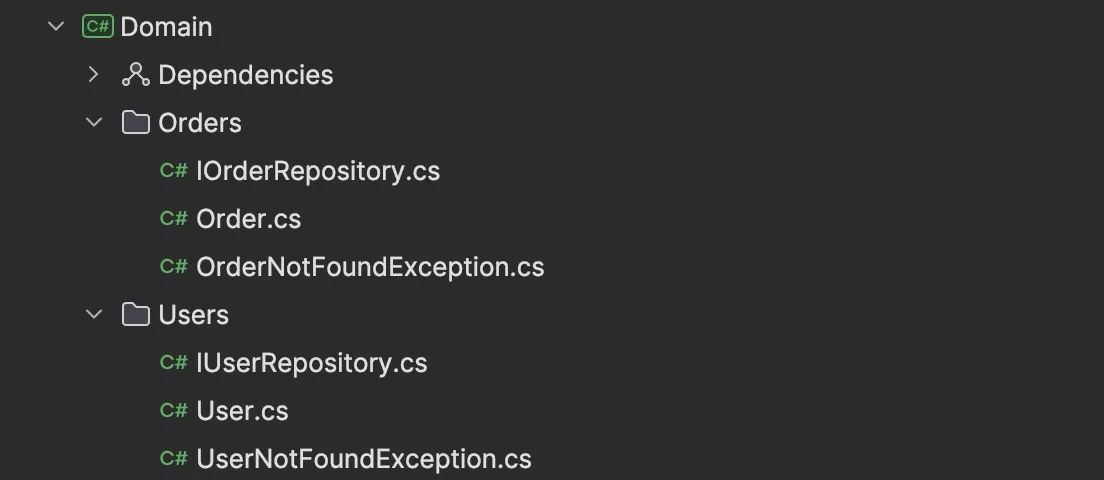
For a large domain layer, grouping by functionality by type (the first approach) may work better, because there will be no problem selecting a folder to put the file in. However, the second approach may have such difficulties, for example, when the same value object belongs to several different entities.
Application Layer
The application layer contains application’s use cases, which can be implemented as command and query handlers or simply as application service classes. In addition, the application layer is usually the place to define infrastructure abstractions (for caching, sending emails, etc).
Here’s what the folder structure might look like:
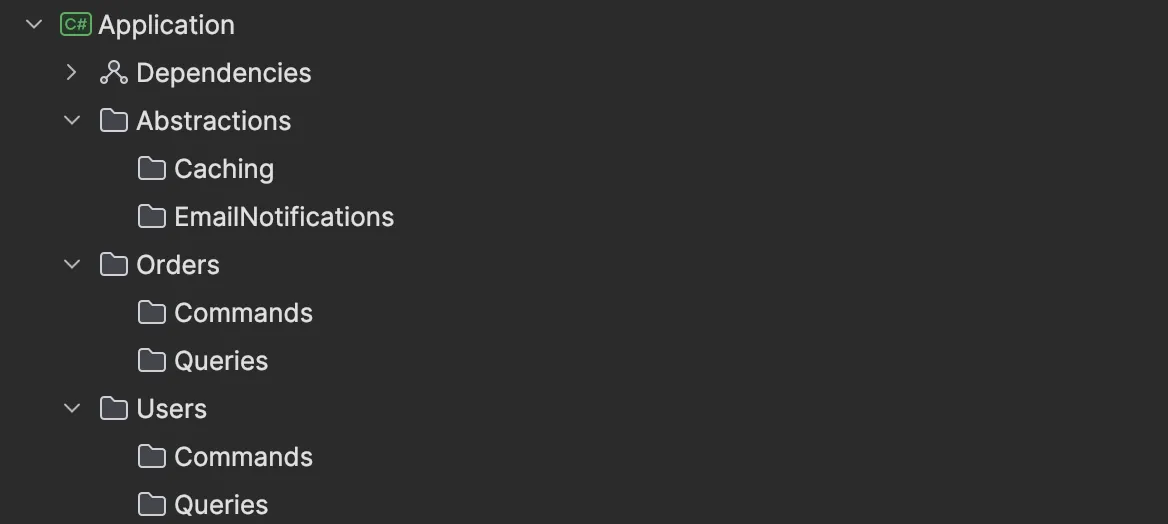
When it comes to references to other projects, the application layer only has a reference to the domain layer.
Infrastructure Layer
The infrastructure layer implements abstractions defined in application and domain layers.
The infrastructure layer can contain:
- Repositories and database migrations
- Implementation of caching services
- Implementation of identity providers
- Implementation of email providers
If the infrastructure layer is implemented as a single project, it may become too bloated over time.
To avoid this problem, we can implement the infrastructure layer as a folder with individual projects in it:
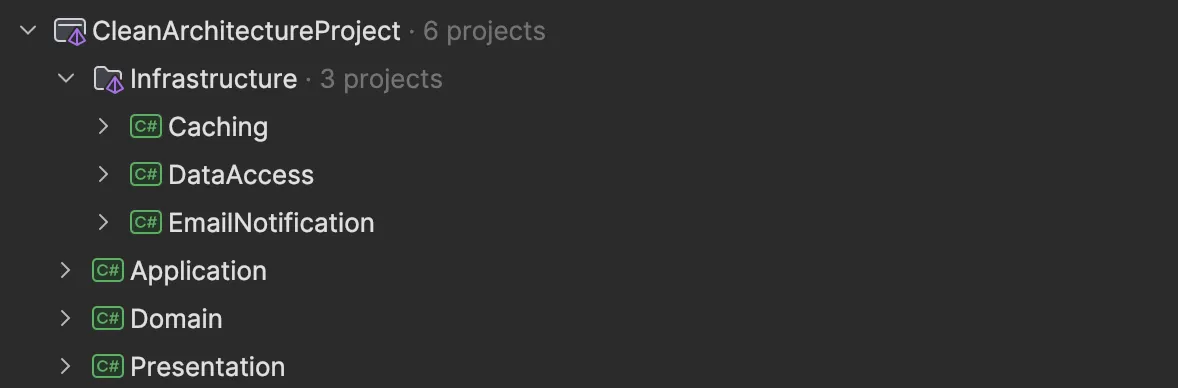
This approach provides a better separation of concerns.
The infrastructure layer must reference the domain and application projects to implement the abstractions defined in them.
Presentation Layer
The presentation layer serves as the entry point to the application.
It contains a public API (for example, RESTful endpoints) that external users or applications can interact with.
In addition to endpoints, the Presentation
layer contains other elements needed to serve incoming requests, such as middlewares.
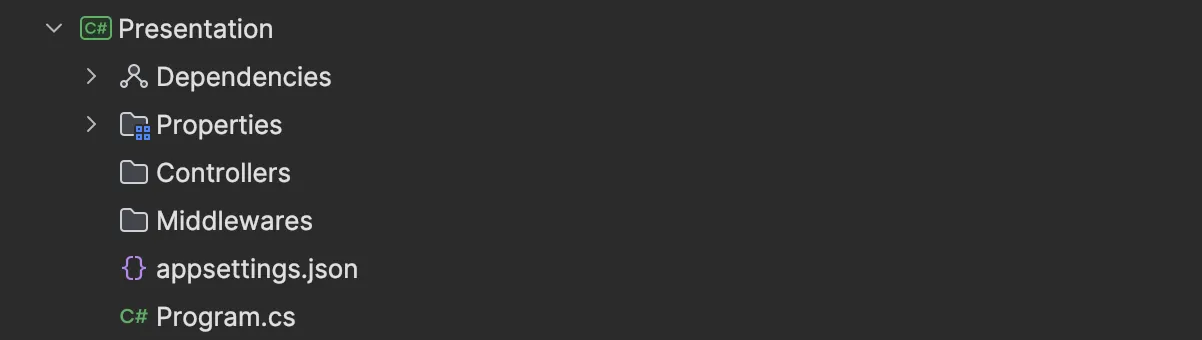
In addition, the presentation project acts as the composition root of the entire solution.
Composition root is where the dependency injection is configured.
Typically it’s done via a series of extension method calls in the Program
class:
builder.Services
.AddDomainServices()
.AddApplicationServices()
.AddDataAccessServices()
.AddEmailNotificationSErvices()
.AddCachingServices();
The presentation layer must reference the application layer in order to execute application use cases.
• • •
We looked at how to structure .NET applications using Clean Architecture principles. We saw how layers of Clean Architecture are mapped to .NET projects, how they should reference each other, and how to organize files within each project.