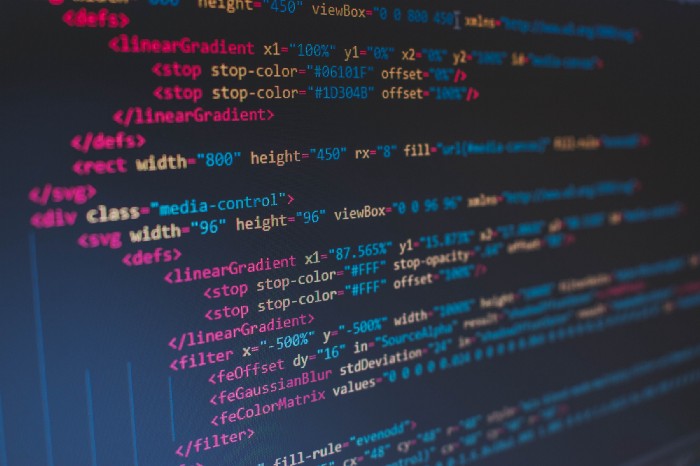
Table Of Content
- Introduction
- Top 6 Tips to optimize the performance of your .Net application
- 1. Avoid throwing exceptions
- 2. Minify your files
- 3. Avoid blocking calls
- 4. Cache your pages
- 5. Optimize custom code
- 6. Minimize large object allocation
- Bonus: Performance Optimization Tips
- Client-side improvement
- Wrapping Up
Introduction
You can find a lot of evidence that shows that due to slow loading times and clunky interaction, customers drive elsewhere and leave your site. Even if we talk about internal applications where users have no other option but to use the app, their satisfaction is highly coupled to speed.
Performance is the key aspect of the success of any application and ASP.NET is one of the best platforms for developing high-performance apps that ensure your product is working up to mark. There are various ways to approach the .NET performance tuning of a web application and perform timely checks to make sure that your app is running well. Using the best .Net development tools such as Visual Studio, NCrunch, Postman, NDepend, etc. can also help developers to develop a high-performing and cutting-edge application.
One of the main reasons behind the popularity of ASP.NET is its performance. And it provides developers with a stable foundation for application development. Therefore, we’re here to share with you the top 6 tips that will help you to optimize the performance of your .Net application. So without any further ado, let’s get started!
Top 6 Tips to Optimize the Performance of your .Net Application
1. Avoid throwing exceptions
Exceptions are one of the major factors to handle errors that might occur in your .Net Core applications. However, it comes with a performance hit so proper management is required else it might harm the app. They are likely one of the most serious resource hogs you will ever see in web applications. For instance, catching a particular exception is better than catching the most generic one, because hiding a problem is never a solution and it never works in the long term.
2. Minify your files
Using a compression tool can help you to decrease the amount of data sent using a wire. But make sure that all the compression-based algorithms that are used to send HTML, CSS, and JavaScript are lossless compression algorithms. So, it results in compress(x) => decompress(x) which is always equal to x.
function doSomething()
{
var size_of_something_to_do = 55;
for (var counter_of_stuff = 0; counter_of_stuff < size_of_something_to_do; counter_of_stuff++)
{
size_of_something_to_do — ;
}
}
is similar to:
function doSomething() {var a = 55; for (var b = 0; b < a; b++) { a — ;}}
Code
This is because the variable scope is limited and the whitespace is not required. This process is called minification which can be applied to CSS and HTML.
3. Avoid Blocking Calls
Design your .Net application in such a way that it executes multiple processes simultaneously using an Asynchronous API. It handles thousands of concurrent requests without waiting on blocking calls. So it is not required to wait for the task to be complete, rather it can start working on another thread. The problem with .NET web apps is blocking asynchronous execution by calling Task. Wait. Also, avoid calling Task. Run because .Net already has some threads that are in the running form leads to additional and unnecessary Thread Pool Scheduling.
Using the hot code paths asynchronous and long-running patterns APIs in your .Net Core application can also help you to enhance your performance and run the app smoothly without any errors. APIs asynchronously. By doing this, your operations can be performed without impacting other processes. Use a Profiler to find the threads that are added to the Thread Pool to make sure that the application is performing well and facilitating tedious tasks.
4. Cache your pages
Caching your pages and invalidating them under specific circumstances helps you to improve the performance of your .Net Core application. The cache is available till the specified time and duration at which you have input and preserve the server from unwanted requests so that overlapping does not occur. Therefore, to increase the performance, make sure you’re not assigning a short expiration to cached items as it expires more frequently and works for the garbage collector. If the page has both dynamic and static sections, partial caching should be used by breaking up your page and specifying caching having fewer static controls.
The data which is included on your pages might change slowly just like the hot questions page. But these changes are not powerful enough to trouble re-querying the database. Instead of rendering your pages, we can shove the page into a cache and serve subsequent requests using that data.
If you’re using ASP.NET MVC caching, then you must be familiar with the action response which is so simple, similar to adding an attribute.
[HandleError]
public class HomeController : Controller
{
[OutputCache(Duration=10, VaryByParam=”none”)]
public ActionResult Index()
{
return View();
}
}
Code
5. Optimize custom code
Code optimization and improving business logic can affect an application’s performance in a positive way. But how? Here’s what you can do:
- Make sure to optimize custom handler code, logging, and authentication that executes and manages every request.
- Avoid performing long-running custom executions as it blocks the request that is going to the server and increases data fetching time for the application. Instead of this, you can optimize your application code on the server or client-side.
- Work asynchronously so that it doesn’t affect other tasks.
- Taking real-time client-server communication, you can easily asynchronously work.
6. Minimize large object allocation
As we all know, the .Net Core garbage collector focuses on managing all the allocations and releases that are not required for the development process and releases unnecessary memory automatically. So we’re not required to worry about the unwanted data and code present as the .Net core takes care of it and cleans unreferenced objects that take CPU time. Garbage collection is costly and large heap generations can optimize the app’s performance. But how?
- Consider caching big objects as it prevents costly allocations.
- Start using ArrayPool<T> which allows you to store large arrays.
- Remove the unwanted large objects on hot code paths which is not required.
Bonus: Performance Optimization Tips
- Perform code review to maintain code control over code.
- Load CSS first and JavaScript last.
- Reduce HTTP request.
- Avoid client-side redirects.
- Use caching to improve performance.
- Use APM tools to identify poorly performing applications.
- Use Prefix to evaluate the distribution of work.
- Use asynchronous database operations.
- Enable compression.
- Use Retrace to detect production issues in a staging environment.
Client-side Improvement:
- Start using ReadFormAsync over Request.Form.
- Avoid storing IHttpContextAccessor.HttpContext in a field.
- Avoid accessing HttpContext from numerous threads as it is not safe and results in undefined behavior.
- Avoid modifying the status code after the response body has started.
- Don’t capture services injected into the controllers.
As we all know, every new version that is introduced comes with a new set of features, functionalities, and better performance. For instance, .NET Core 2.1 came up with a JIT compiler that gets support from compiled regular expressions and Span <T> tags. If we talk about .Net Core 2.2, it has support for HTTP/2 whereas 3.0 delivered fast memory writing and reading. The latest .Net version 5 is planning to introduce some new features with optimized performance capabilities, and a simplified process. So that it becomes easier for developers to develop a high-performing app in the newer version compared to the previous one.
Wrapping Up
In closing, we can say that the quality of the large-scale application is limited which governs their development. If you’re not ready with the proper pre-production processes, we will inevitably introduce poor-performing code to our users. But using the Prefix pre-production tool and Retrace, we can gain insights into the performance impact of changes before bringing down critical systems.
All these 6 tips will surely help you to enhance the performance of your .Net Core application. However, you might find it hard to check everything when you have a large-scale application. So a better and more effective way is to use an automatic tool that will help you to detect errors before it’s too late and reduce the harmful effects that might be dangerous.
We hope you find this post and the tips that we discussed to help you to improve the performance of your .Net Core application. Try to implement more of it to get the best and highly optimized performance that every user is expecting. If you face any challenges while implementing any tips, you can get in touch with the best software development company that offers the best .Net development services to overcome all your project challenges.
It would be great for us if you share this article on Facebook or Twitter to reach more audiences. Feel free to approach us in the comment section given below and ask your queries.
Thank you!