Master the art of caching in .NET applications to improve performance and user experience.
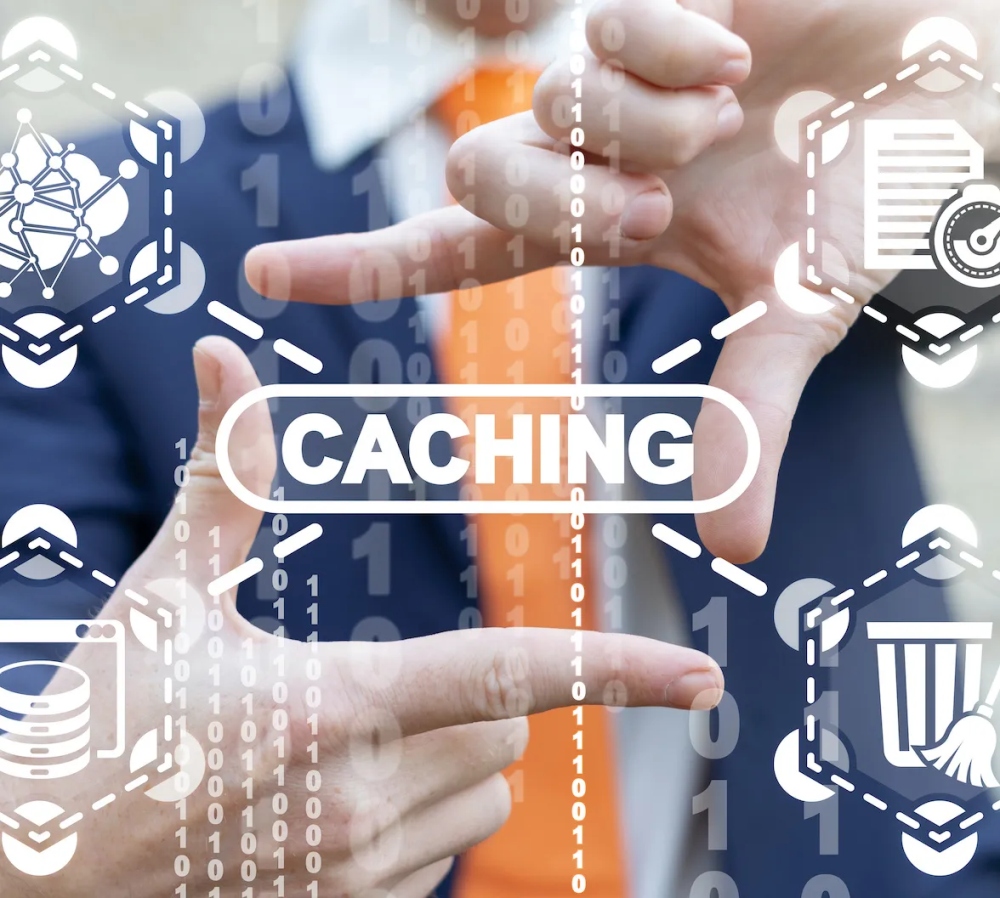
Caching is a powerful technique to improve application performance and response times. By temporarily storing the results of expensive operations or frequently accessed data, you can reduce the load on your system and provide faster response times to users. In this article, I will explore various caching strategies and techniques in .NET to help you become a caching expert and boost your application’s performance.
1. In-Memory Caching
In-memory caching stores data within the application’s memory space, providing quick access times. .NET provides the MemoryCache
class, which is an implementation of the IMemoryCache
interface, for in-memory caching.
Example: using MemoryCache
using System.Runtime.Caching;
public class DataCache
{
private readonly MemoryCache cache;
public DataCache()
{
cache = new MemoryCache("DataCache");
}
public T GetOrCreate<T>(string key, Func<T> createItem, DateTimeOffset absoluteExpiration)
{
if (!cache.Contains(key))
{
var item = createItem();
cache.Add(key, item, absoluteExpiration);
}
return (T)cache[key];
}
public void Remove(string key)
{
cache.Remove(key);
}
}
2. Distributed Caching
Distributed caching stores data across multiple nodes or services, making it suitable for scaling and sharing data across applications. Some popular distributed caching solutions for .NET include:
Example: using IDistributedCache
with Redis.
using Microsoft.Extensions.Caching.Distributed;
using Newtonsoft.Json;
public class DistributedDataCache
{
private readonly IDistributedCache cache;
public DistributedDataCache(IDistributedCache cache)
{
this.cache = cache;
}
public async Task<T> GetOrCreateAsync<T>(string key, Func<Task<T>> createItem, DistributedCacheEntryOptions options)
{
var data = await cache.GetStringAsync(key);
if (data == null)
{
var item = await createItem();
data = JsonConvert.SerializeObject(item);
await cache.SetStringAsync(key, data, options);
}
return JsonConvert.DeserializeObject<T>(data);
}
public async Task RemoveAsync(string key)
{
await cache.RemoveAsync(key);
}
}
3. Output Caching
Output caching stores the rendered output of a web request, reducing the processing time for subsequent requests. In ASP.NET Core, you can use the `ResponseCache` attribute to apply output caching to your actions. Example: Using `ResponseCache` attribute in ASP.NET Core.
using Microsoft.AspNetCore.Mvc;
public class HomeController : Controller
{
[HttpGet]
[ResponseCache(Duration = 60, Location = ResponseCacheLocation.Any, NoStore = false)]
public IActionResult Index()
{
return View();
}
}
4. Cache Invalidation
Proper cache invalidation is crucial for ensuring data consistency. Use the following strategies to manage cache invalidation:
- Cache duration: Set a suitable cache duration based on the data’s volatility.
- Cache dependencies: Set cache dependencies to invalidate the cache when a dependency changes.
- Cache eviction: Remove items from the cache when memory usage exceeds a predefined limit.
5. Cache-Aside Pattern
The cache-aside pattern is a popular caching strategy that involves loading data into the cache on demand. When data is requested, the cache is checked first. If the data is not present in the cache, it is retrieved from the primary data sttore, stored in the cache, and returned to the user.
Example: implementing the cache-aside pattern:
public async Task<Product> GetProductAsync(int id)
{
string key = $"Product_{id}";
var product = cache.Get<Product>(key);
if (product == null)
{
product = await productRepository.GetProductAsync(id);
if (product != null)
{
var options = new DistributedCacheEntryOptions
{
AbsoluteExpirationRelativeToNow = TimeSpan.FromMinutes(60)
};
await cache.SetAsync(key, product, options);
}
}
return product;
}
6. Cache Consistency
Maintaining cache consistency is essential for preventing stale or outdated data from being served. You can use the following techniques to ensure cache consistency:
- Write-through: Update the cache and primary data store simultaneously.
- Write-behind: Update the primary data store first, then update the cache asynchronously.
- Write-around: Update the primary data store, then remove the cache entry, allowing it to be fetched and cached on the next request.
7. Monitoring and Metrics
Monitor cache performance to ensure it is meeting your application’s needs. Collect metrics such as cache hit rate, cache miss rate, cache latency, and cacshe size to make informed decisions about your caching strategy.
Conclusion
Caching is an essential technique for improving .NET application performance and user experience. By understanding and implementing various caching strategies such as in-memory caching, distributed caching, output caching, cache invalidation, and the cache-aside pattern, you can optimize your application’s response times.
Additionally, maintaining cache consistency and monitoring cache performance through metrics will help you fine-tune your caching strategies and ensure that your application delivers the best possible user experience.
With these strategies and techniques in hand, you are well-equipped to wield the power of caching in .NET applications and improve performance like a true Jedi developer.