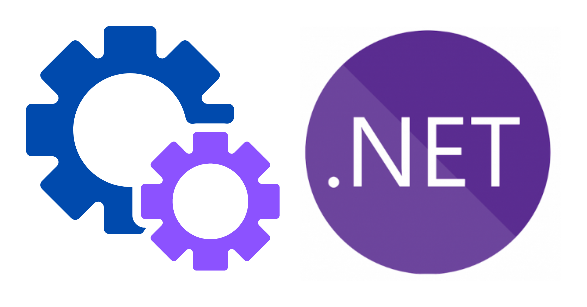
Suppose we are building a web api that contains a route to send notification messages to other systems.
For security matters, before sending any notification message, we need to provide some credentials to these systems to they accept our messages.
However, these credentials are stored in an external data source, and not in our appSettings.json. How can we load these credentials as application configuration properties?
A good approach for that is to create a custom Configuration Provider. Customs Configuration Providers from .NET. It allows us to read and load settings from external data sources.
This article presents three simple steps to read settings from a local file (notification_values.json).
1. Implement a Configuration Provider
To read settings from an external data source we need to implement a new class that inherits the ConfigurationProvider abstract class.
This abstract class is part of Microsoft.Extensions.Configuration namespace.
See the code below:
1 public class NotificationApiConfigurationProvider : Microsoft.Extensions.Configuration.ConfigurationProvider
2 {
3 public override void Load()
4 {
5 var fileContent = File.ReadAllText(@"notification_values.json");
6 var content = JsonSerializer.Deserialize<Notification>(fileContent, new JsonSerializerOptions
7 {
8 PropertyNamingPolicy = JsonNamingPolicy.CamelCase,
9 });
10
11 if(content == null) return;
12
13 Data = new Dictionary<string, string>
14 {
15 {"Notification:ApiKey", content.ApiKey},
16 {"Notification:Url", content.Url},
17 {"Notification:Method", content.Method}
18 };
19 }
20 }
The class NotificationApiConfigurationProvider inherits methods and properties from the ConfigurationProvider class. However, to read and load the configurations from a local file, we need to override the Load method from ConfigurationProvider abstract class.
From the Load method, after reading the properties from an external source, they will store in the Data property (see line 13).
Now, we need to create a source to initiate this new configuration provider.
2. Implement a Configuration Source
The IConfigurationSource interface contains the Build method. With this method, we can apply all we need to initiate our custom configuration provider.
See the code below:
public class NotificationApiConfigurationSource : IConfigurationSource
{
public IConfigurationProvider Build(IConfigurationBuilder builder)
{
return new NotificationApiConfigurationProvider();
}
}
For this sample, I created the NotificationApiConfigurationSource class that inherits the IConfigurationSource interface.
As you can see this interface has only one method, the Build method. Basically, the method implementation was to return a new instance from our custom configuration provider, that will be available when the application starts.
At this moment, we have the custom configuration provider and the configuration source ready.
The next step is about how to read the configuration in our application.
3. Load settings from Configuration Provider
The code below belongs to the Program class. Take a look at line 4:
1 using ConfigurationProvider.API.Extensions;
2
3 var builder = WebApplication.CreateBuilder(args);
4 builder.Configuration.AddNotificationConfiguration();
5
6 // Add services to the container.
7
8 builder.Services.AddControllers();
9 // Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
10 builder.Services.AddEndpointsApiExplorer();
11 builder.Services.AddSwaggerGen();
12
13 var app = builder.Build();
14
15 // Configure the HTTP request pipeline.
16 if (app.Environment.IsDevelopment())
17 {
18 app.UseSwagger();
19 app.UseSwaggerUI();
20 }
21
22 app.UseHttpsRedirection();
23
24 app.UseAuthorization();
25
26 app.MapControllers();
27
28 app.Run();
As you can see in line 4, the extension method AddNotificationConfiguration is called at this moment.
This extension method from the IConfigurationBuilder interface will create a new instance from the NotificationApiConfigurationSource class.
using ConfigurationProvider.API.CustomProvider;
namespace ConfigurationProvider.API.Extensions;
public static class ConfigurationBuilderExtensions
{
public static IConfigurationBuilder AddNotificationConfiguration(this IConfigurationBuilder builder)
{
builder.Add(new NotificationApiConfigurationSource());
return builder;
}
}
Basically, a new instance of NotificationApiConfigurationSource class is created. Then, when the application starts the custom provider will read all settings from the external data source and let them available in the application.
Now, the configuration can easily be read from any part of the Web API. For example, the code below shows how to read from a Controller class.
using ConfigurationProvider.API.Model;
using Microsoft.AspNetCore.Mvc;
namespace ConfigurationProvider.API.Controllers;
[ApiController]
[Route("[controller]")]
public class NotificationController : ControllerBase
{
private readonly IConfiguration _configuration;
public NotificationController(IConfiguration configuration)
{
_configuration = configuration;
}
[HttpGet]
public IActionResult Get()
{
var notification = new Notification
{
ApiKey = _configuration["Notification:ApiKey"],
Method = _configuration["Notification:Url"],
Url = _configuration["Notification:Method"]
};
return Ok(notification);
}
}
I hope that this can be helpful for you! Happy coding!