Table of contents
I. API design style
1. gRPC
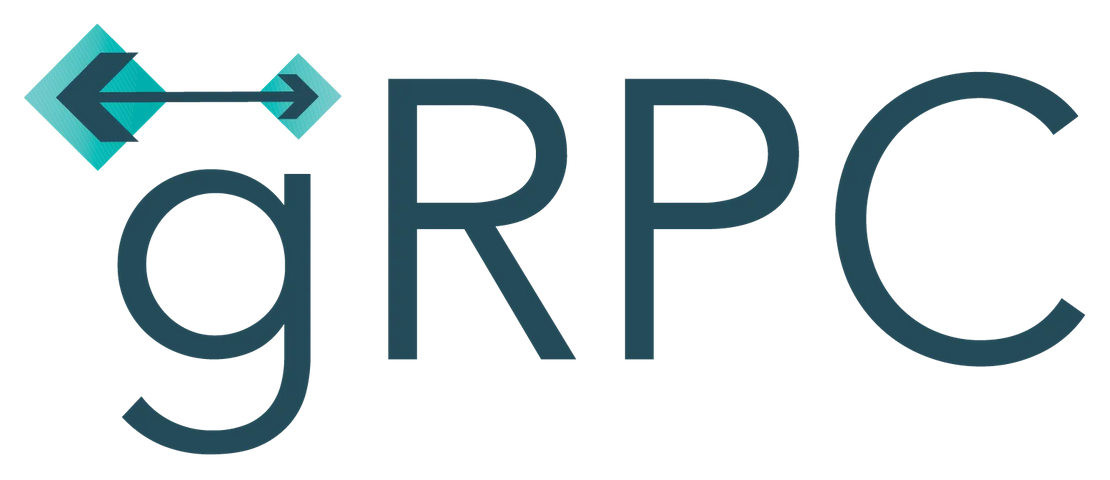
Pros:
- Efficient binary format using Protocol Buffers, leading to better performance and smaller payloads.
- Supports bi-directional streaming, allowing for real-time communication.
- Language-agnostic, with support for multiple programming languages.
- Strongly-typed schema enforces API contracts and reduces errors.
Cons:
- Less human-readable compared to JSON-based APIs.
- Limited browser support, as it uses HTTP/2 by default.
- Smaller community and less mature ecosystem compared to REST.
When to use: gRPC is well-suited for high-performance applications and microservices, where the efficient exchange of data is crucial. It’s also a great choice when you need to support real-time communication and bi-directional streaming.
2. GraphQL
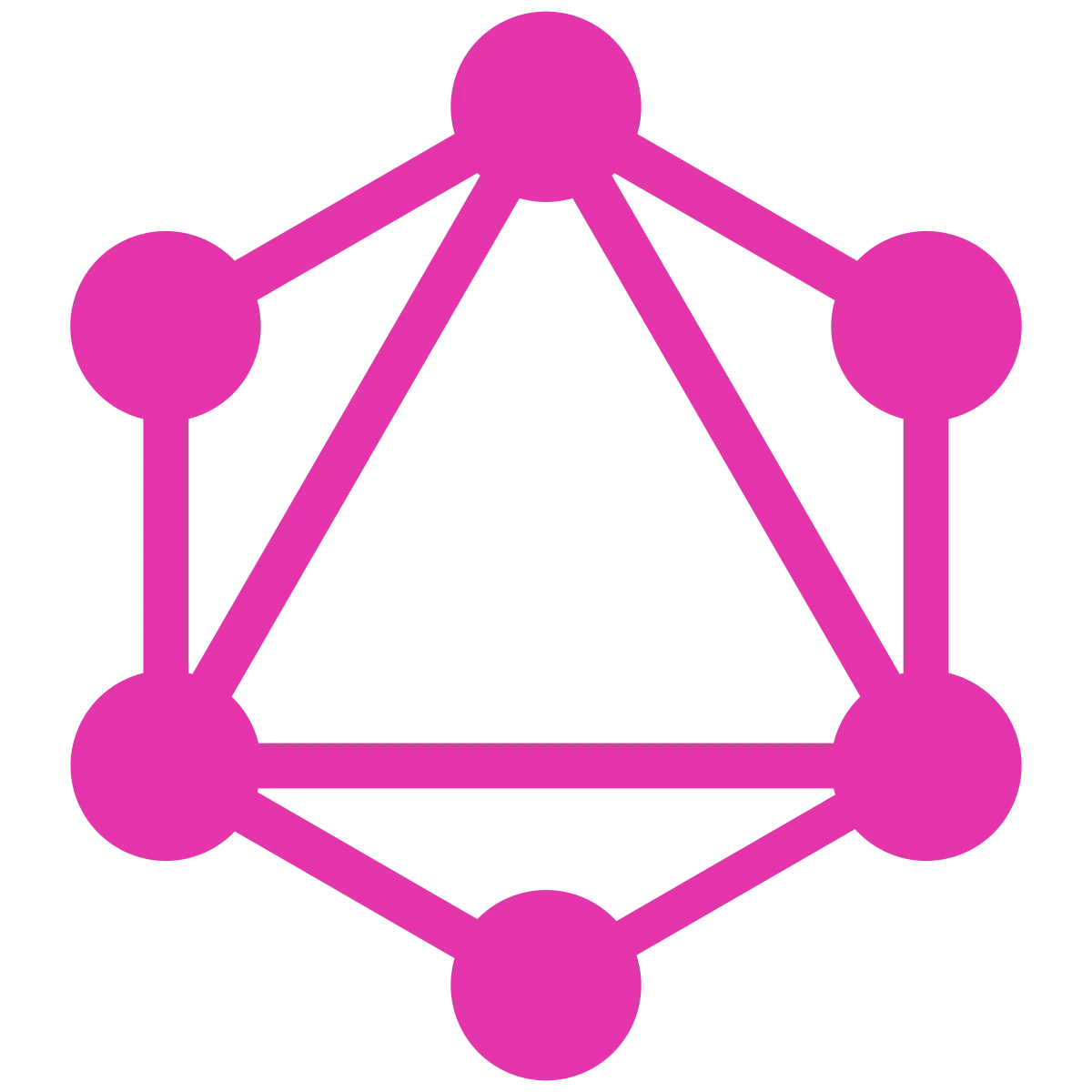
Pros:
- Flexible query language allows clients to request only the data they need.
- Consolidates multiple API requests into a single request, reducing latency and overhead.
- Supports real-time updates via subscriptions.
- Strongly-typed schema enforces API contracts and reduces errors.
Cons:
- Requires learning a new query language.
- Lacks support for some common API features like caching and real-time updates out-of-the-box.
- Smaller community and less mature ecosystem compared to REST.
When to use: GraphQL is an excellent choice for applications where clients need to fetch varying data structures and need flexibility in API response format. It’s particularly useful for mobile and front-end heavy applications that require optimized data fetching and reduced network overhead.
3. REST
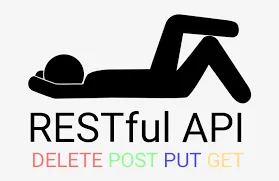
Pros:
- Simple and easy to understand, with a wide range of tools and libraries available.
- Based on standard HTTP methods, making it compatible with most clients and servers.
- Supports caching and other HTTP features out-of-the-box.
- Large community and a mature ecosystem of tools and best practices.
Cons:
- Can lead to over-fetching or under-fetching of data, resulting in increased latency and network overhead.
- Lacks support for real-time updates and subscriptions.
- No built-in support for strongly-typed schemas.
When to use: REST is a popular and widely-adopted API design style that works well for most applications. It’s a good choice for projects with simple data requirements and a need for compatibility with a wide range of clients and servers.
In summary, the choice of API design style depends on the specific requirements of your project. gRPC is a great choice for high-performance applications and microservices, GraphQL offers flexibility and optimized data fetching for client-heavy applications, and REST is a widely-adopted and compatible choice for most projects.
II. Database
1. Microsoft SQL Server
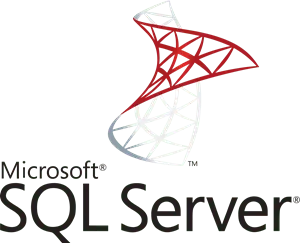
Pros:
- Robust and feature-rich, offering advanced tools for performance tuning, security, and scalability.
- Deep integration with .NET and the Microsoft ecosystem.
- Supports SQL, making it easier for developers familiar with relational databases.
- Offers comprehensive documentation and a large community.
Cons:
- Can be expensive, particularly for enterprise editions.
- Limited to the Windows platform (although a Linux version is available).
When to use: SQL Server is an excellent choice for organizations invested in the Microsoft ecosystem or for those who need a robust, feature-rich, and scalable relational database system.
2. PostgreSQL
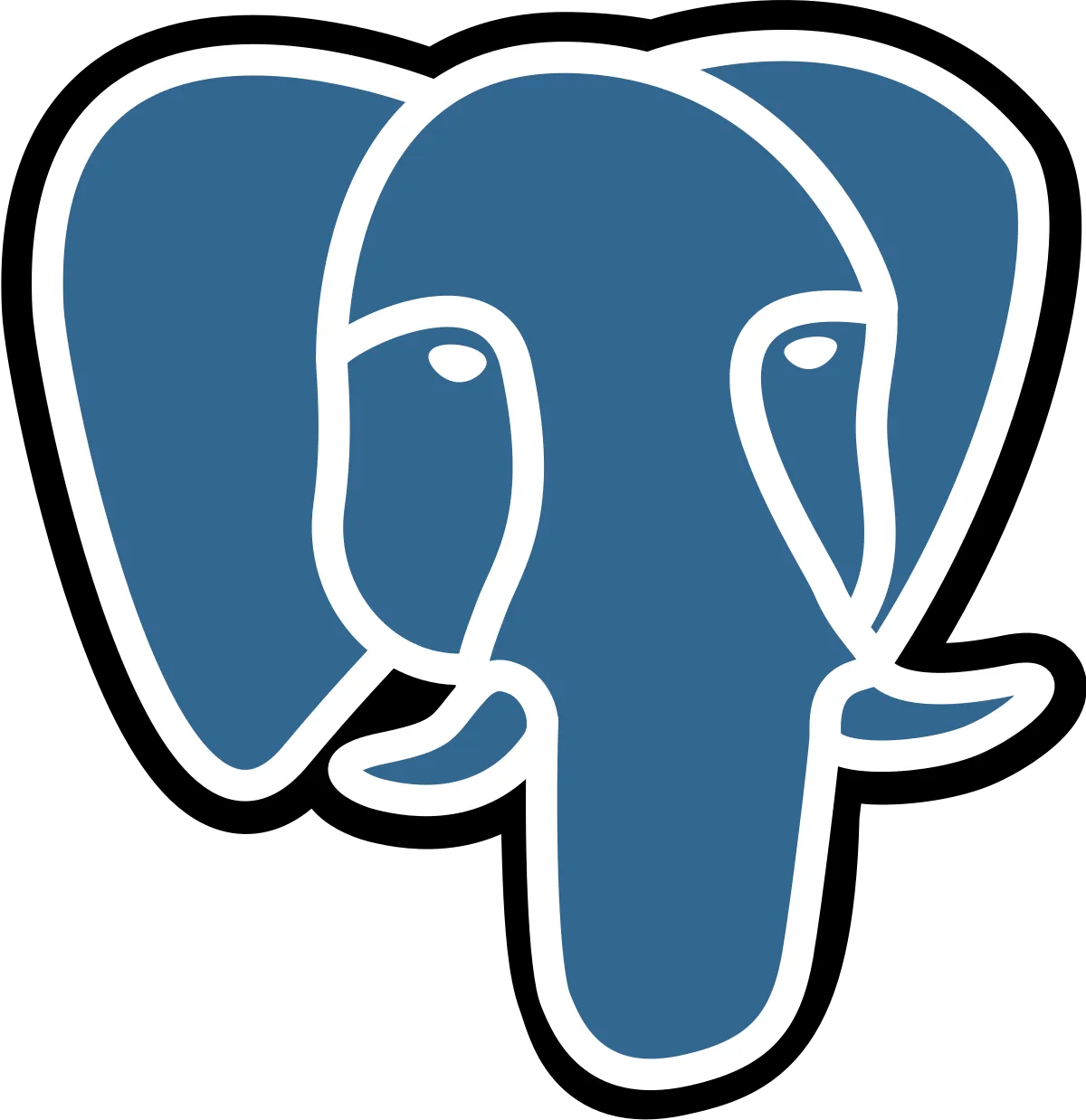
Pros:
- Open-source, with a strong community and a rich ecosystem of tools and extensions.
- Highly extensible, allowing developers to define custom data types, operators, and functions.
- Supports advanced features like full-text search, spatial data types, and JSON support.
- Cross-platform, supporting multiple operating systems.
Cons:
- May have a steeper learning curve compared to other relational databases.
- Lower performance in certain workloads compared to commercial databases like SQL Server.
When to use: PostgreSQL is a good choice for projects that require an open-source, extensible, and cross-platform relational database system with advanced features.
3. MySQL
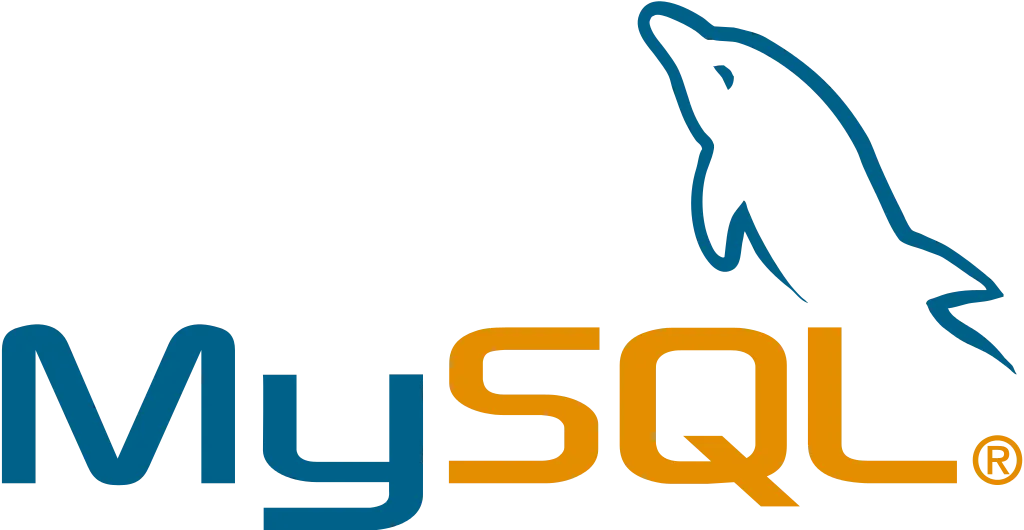
Pros:
- Open-source and widely-used, with a large community and extensive documentation.
- Easy to set up and use, making it suitable for beginners.
- Supports SQL, making it familiar for developers experienced with relational databases.
- Cross-platform, supporting multiple operating systems.
Cons:
- Lacks some advanced features found in other relational databases, like PostgreSQL.
- Owned by Oracle, which may raise concerns about its long-term open-source commitment.
When to use: MySQL is a popular choice for web applications and small to medium-sized projects that require a reliable, easy-to-use, and widely-supported relational database system.
4. MongoDB
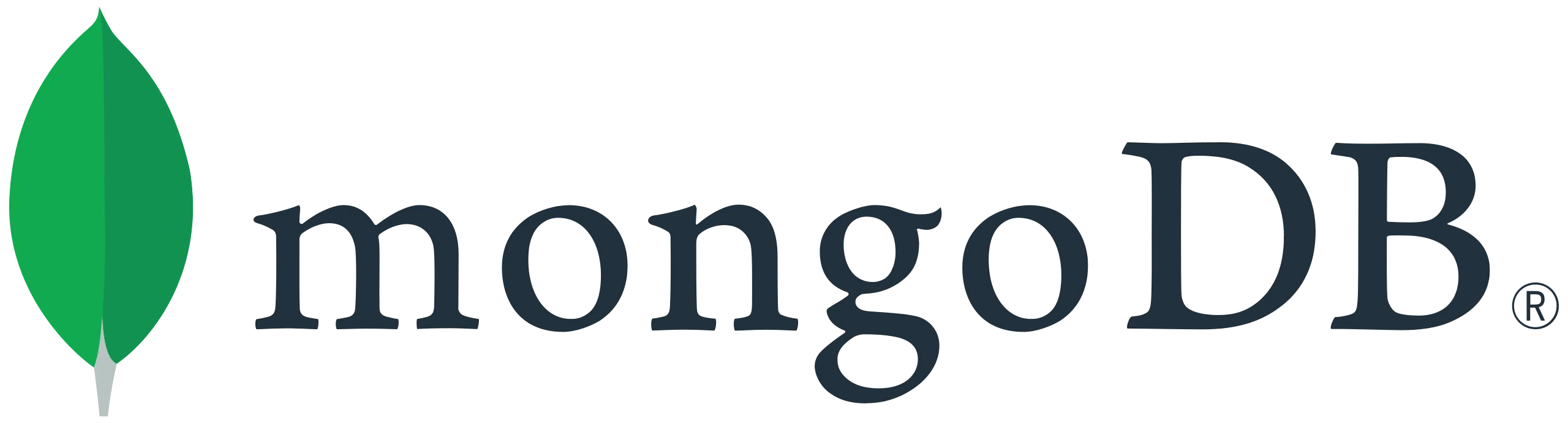
Pros:
- Schema-less, offering flexibility in data modeling.
- Supports horizontal scaling through sharding.
- JSON-based document storage, making it easier to work with for developers familiar with JavaScript.
- Large community and extensive documentation.
Cons:
- Lacks support for transactions and joins, which are common in relational databases.
- Inconsistent performance under certain workloads.
When to use: MongoDB is a good choice for projects that require a flexible and scalable NoSQL database, particularly for applications with rapidly evolving data structures or heavy read/write workloads.
5. Couchbase
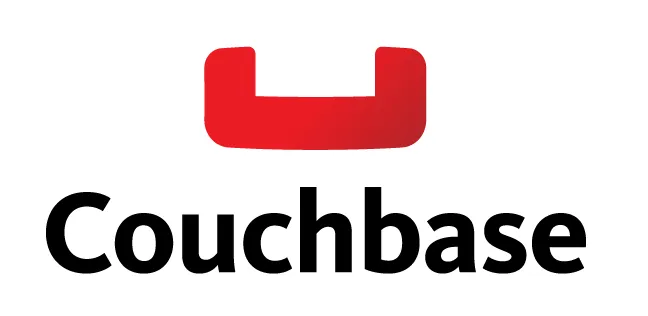
Pros:
- Offers high performance and low-latency data access.
- Supports horizontal scaling through clustering and automatic data sharding.
- Built-in caching layer, reducing the need for separate caching mechanisms.
- Strong support for mobile and edge computing with Couchbase Lite and Sync Gateway.
Cons:
- Commercial editions can be expensive.
- Smaller community compared to more popular NoSQL databases like MongoDB.
When to use: Couchbase is suitable for projects that require high performance, low latency, and strong support for mobile and edge computing, particularly in real-time or distributed applications.
6. Cassandra
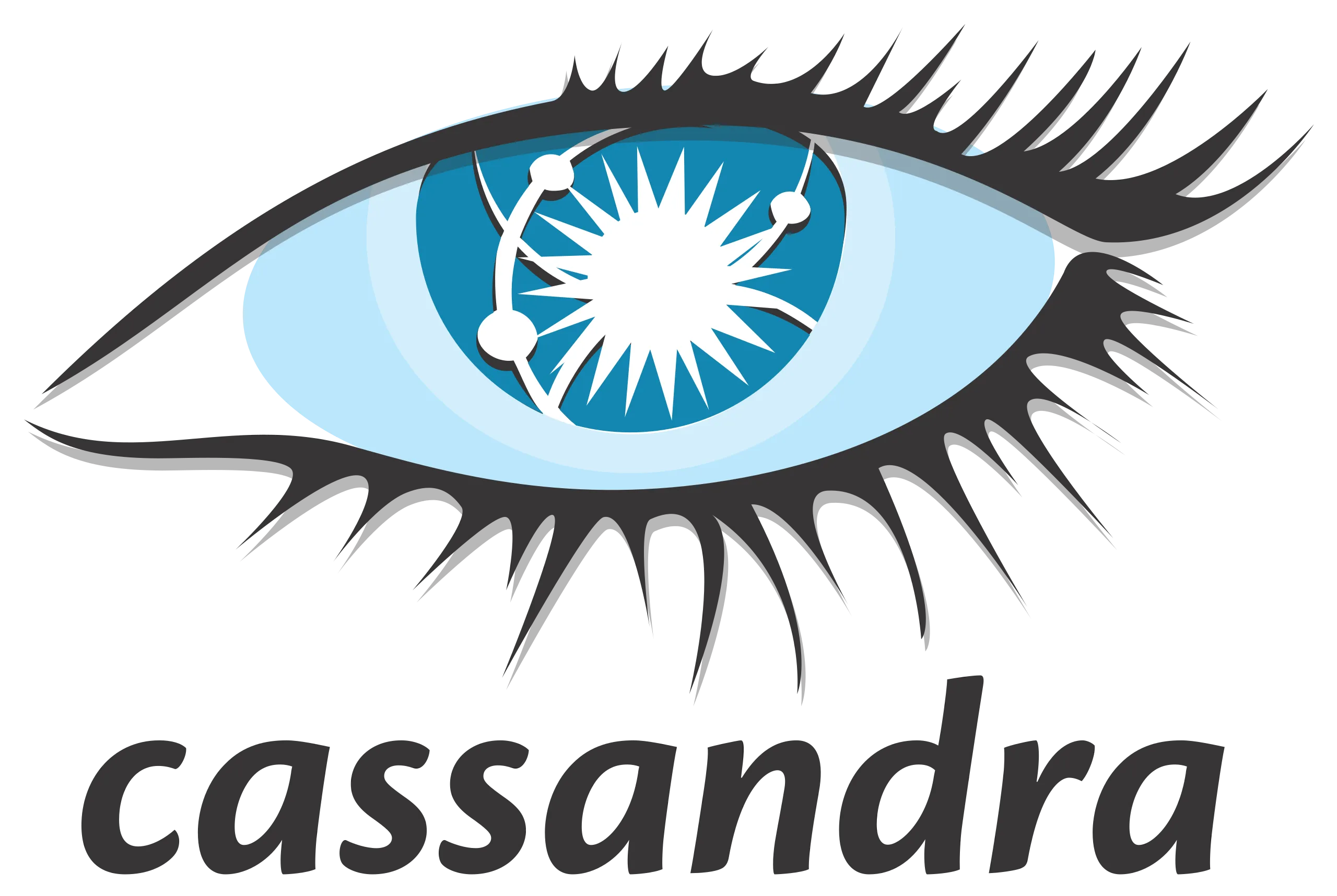
Pros:
- Designed for high availability and fault tolerance, with no single point of failure.
- Linear scalability, making it suitable for large-scale, distributed applications.
- Supports tunable consistency, allowing developers to choose the desired level of data consistency.
- Wide-column data model enables efficient querying and storage of large amounts of data.
Cons:
- Steeper learning curve compared to other databases.
- Lacks support for complex transactions and joins, which are common in relational databases.
III. Caching mechanisms
1. Redis
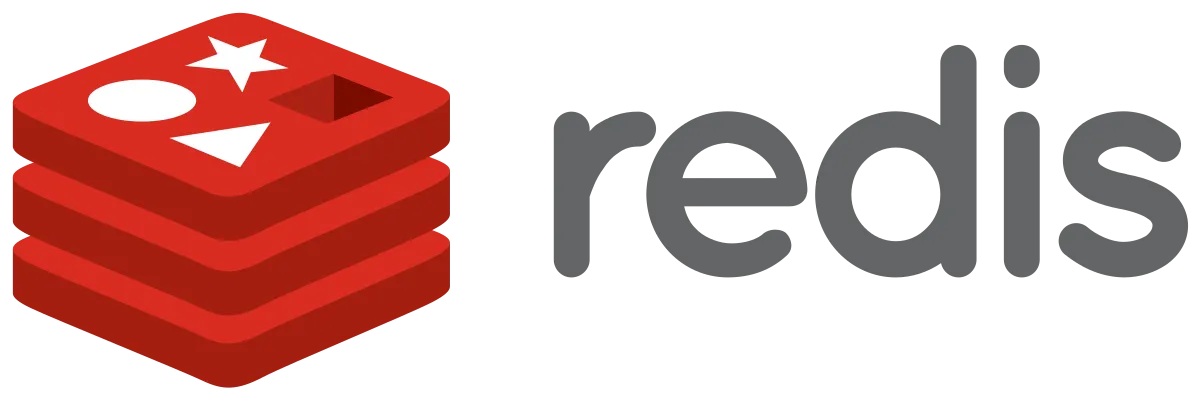
Pros:
- High performance, offering in-memory data storage for low-latency access.
- Supports a variety of data structures, such as strings, hashes, lists, sets, and sorted sets.
- Offers built-in support for data replication, persistence, and clustering.
- Large community and extensive documentation.
Cons:
- Limited security features out-of-the-box, requiring additional configuration and setup.
- May consume more memory compared to other caching mechanisms due to its in-memory storage.
When to use: Redis is a great choice for projects that require high-performance, low-latency caching, and support for various data structures. It’s particularly useful for applications with heavy read/write workloads, such as real-time analytics or gaming.
2. Memcached
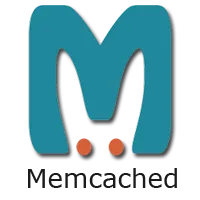
Pros:
- Simple and easy to use, with a focus on key-value storage.
- Low memory overhead and efficient memory usage.
- Offers support for multiple programming languages.
- Widely used and well-documented.
Cons:
- Lacks support for advanced data structures and features, such as data persistence and replication.
- No built-in support for clustering or horizontal scaling.
When to use: Memcached is suitable for projects that require a simple, lightweight, and easy-to-use caching mechanism. It’s a good choice for web applications with high read and low write workloads, such as content-heavy websites.
3. NCache
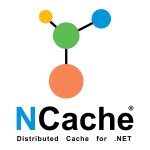
Pros:
- Designed specifically for .NET applications, with deep integration and support for .NET features.
- Offers advanced features like data replication, data partitioning, and distributed caching.
- Provides built-in support for ASP.NET session state and output caching.
- Commercial editions offer additional features and professional support.
Cons:
- Limited to .NET applications, unlike Redis and Memcached, which support multiple languages.
- Commercial editions can be expensive compared to open-source alternatives.
When to use: NCache is an excellent choice for .NET applications that require advanced caching features, such as data replication, data partitioning, and distributed caching. It’s particularly useful for enterprise-level applications with high scalability and performance requirements.
4. Microsoft.Extensions.Caching.Memory (MemoryCache)
Pros:
- Built-in .NET caching mechanism, requiring no additional dependencies.
- Easy to use and configure, with native support for .NET types and objects.
- Provides a simple in-memory cache store for fast data access.
- Supports cache expiration policies and dependencies.
Cons:
- Lacks advanced features like data replication and distributed caching.
- Limited to the scope of a single application instance, making it unsuitable for distributed applications.
When to use: MemoryCache is a good choice for small to medium-sized .NET applications that require a simple and easy-to-use caching mechanism. It’s suitable for applications that don’t require advanced features like data replication or distributed caching.
In summary, the choice of caching mechanism depends on the specific requirements of your project. Redis offers high performance and support for various data structures, Memcached provides simplicity and ease of use, NCache is a powerful option for .NET applications with advanced caching needs, and MemoryCache is a built-in solution for simple caching scenarios in .NET applications.
IV. Logging and monitoring
1. ELK Stack (Elasticsearch, Logstash, and Kibana)
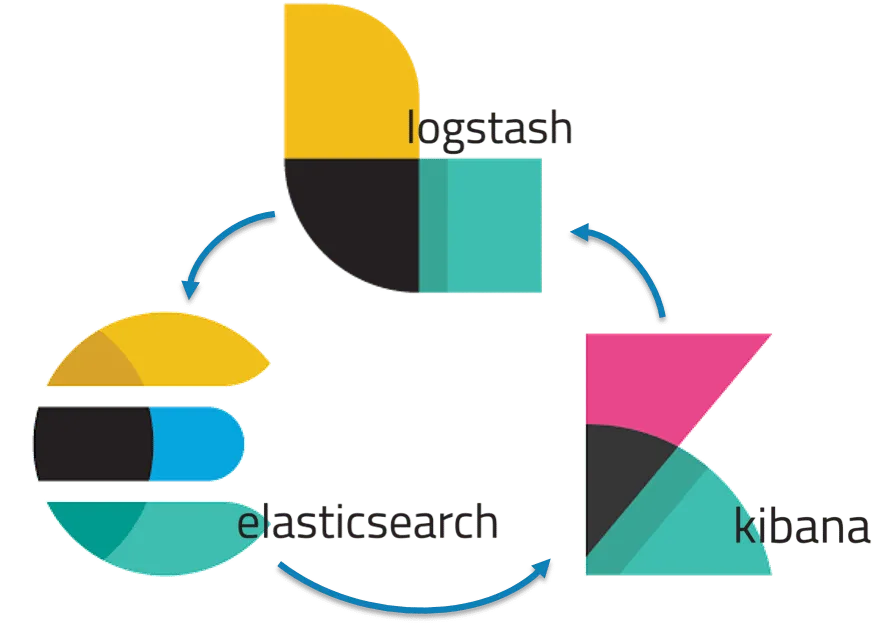
Pros:
- Offers a comprehensive solution for log aggregation, storage, and analysis.
- Elasticsearch provides a powerful search engine for fast and efficient log querying.
- Logstash enables log data ingestion from multiple sources and formats.
- Kibana offers a web-based interface for log visualization and analysis.
- Highly scalable and capable of handling large volumes of log data.
Cons:
- Can be complex to set up and configure, particularly for large-scale deployments.
- May require significant resources for optimal performance.
When to use: The ELK Stack is an excellent choice for projects that require a comprehensive and scalable logging and monitoring solution. It’s particularly useful for organizations with large volumes of log data or those that need advanced log analysis and visualization capabilities.
2. Serilog
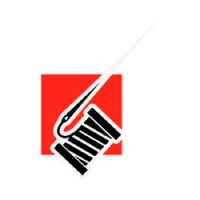
Pros:
- Designed specifically for .NET applications, with native support for structured logging.
- Offers a wide range of sinks for log output, including file, console, and popular log aggregation services.
- Easy to set up and configure, with a flexible and extensible API.
- Supports log filtering and enrichment for better log context and analysis.
Cons:
- Limited to .NET applications, unlike some other logging libraries that support multiple languages.
- Lacks built-in log aggregation and visualization features.
When to use: Serilog is a great choice for .NET applications that require structured logging and support for various log output sinks. It’s suitable for projects that need a flexible and extensible logging library with native .NET integration.
3. NLog
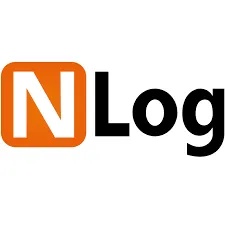
Pros:
- Offers a high-performance and flexible logging solution for .NET applications.
- Supports a wide range of log output targets, including files, databases, email, and log aggregation services.
- Provides advanced features like log routing, filtering, and buffering.
- Easy to set up and configure with a robust configuration system.
Cons:
- Limited to .NET applications, unlike some other logging libraries that support multiple languages.
- Lacks built-in log aggregation and visualization features.
When to use: NLog is a good choice for .NET applications that require a high-performance and flexible logging solution. It’s suitable for projects that need advanced logging features like log routing, filtering, and buffering, as well as support for various log output targets.
4. Application Insights (part of Azure Monitor)
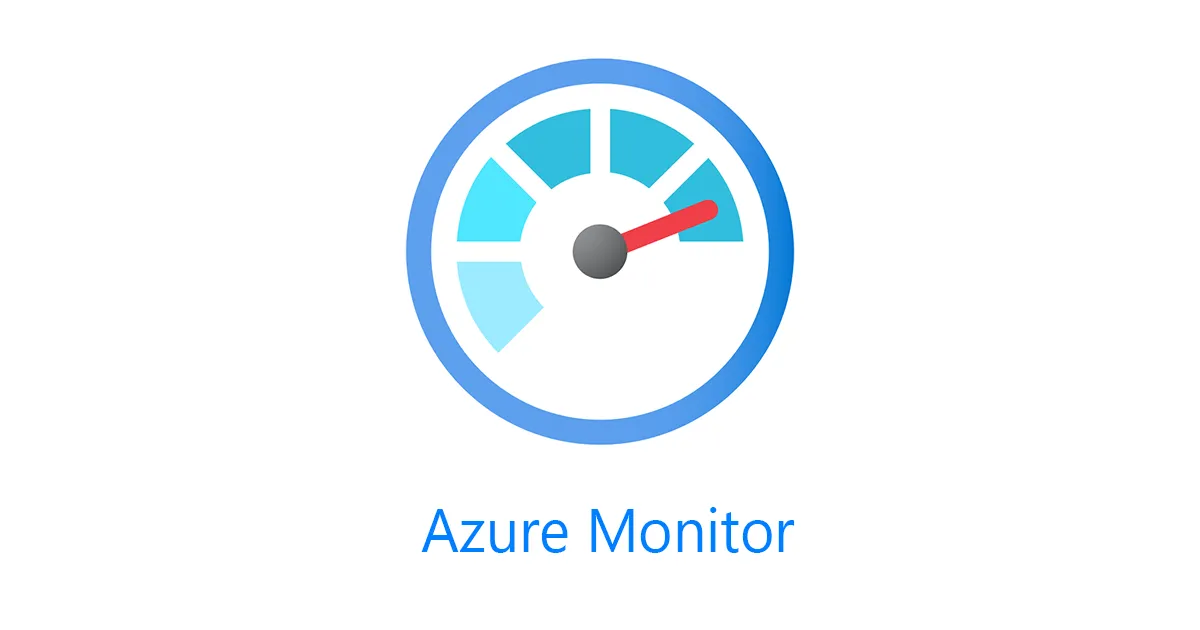
Pros:
- Offers a comprehensive application performance management (APM) solution for .NET applications.
- Deep integration with Azure and the Microsoft ecosystem.
- Provides built-in support for log aggregation, visualization, and analysis.
- Includes features for application monitoring, diagnostics, and alerting.
Cons:
- Tightly coupled with Azure, which may not be ideal for organizations using other cloud providers or on-premise deployments.
- Can be more expensive compared to open-source alternatives.
When to use: Application Insights is an excellent choice for organizations invested in the Azure ecosystem or those who need a comprehensive APM solution for their .NET applications. It’s particularly useful for projects that require built-in support for log aggregation, visualization, and analysis, as well as application monitoring and diagnostics.
In summary, the choice of logging and monitoring tools depends on the specific requirements of your project. The ELK Stack offers a comprehensive and scalable solution for log management, Serilog and NLog provide flexible and extensible logging libraries for .NET applications, and Application Insights delivers a powerful APM solution with deep Azure integration.
V. Authentication & Authorization
In this section, we will cover four popular tools and libraries for authentication and authorization, including JWT and Azure AD, along with their pros and cons, and when to use each one effectively.
1. JWT (JSON Web Tokens)
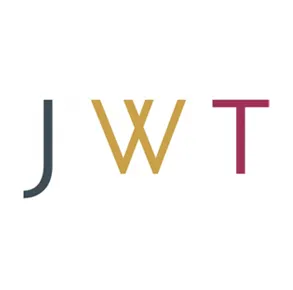
Pros:
- Lightweight and stateless, making it suitable for scalable and distributed applications.
- Offers a standardized method for securely representing and exchanging claims between parties.
- Can be used with various authentication and authorization mechanisms.
- Supported by a wide range of programming languages and libraries.
Cons:
- Requires careful handling and storage, as compromised tokens can lead to security breaches.
- Lacks built-in support for token revocation, which may require additional mechanisms to handle.
When to use: JWT is a great choice for projects that require a stateless and scalable method for handling authentication and authorization. It’s particularly useful for API-driven applications, single-page applications (SPAs), and microservices.
2. Azure AD (Azure Active Directory)
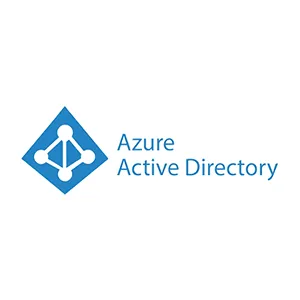
Pros:
- Offers a comprehensive identity and access management (IAM) solution for the Microsoft ecosystem.
- Provides built-in support for single sign-on (SSO), multi-factor authentication (MFA), and conditional access.
- Integrates seamlessly with other Azure services and Microsoft applications.
- Supports various identity protocols like OAuth 2.0, OpenID Connect, and SAML.
Cons:
- Tightly coupled with Azure, which may not be ideal for organizations using other cloud providers or on-premise deployments.
- Can be more expensive compared to open-source alternatives.
When to use: Azure AD is an excellent choice for organizations invested in the Microsoft ecosystem or those who need a comprehensive IAM solution. It’s particularly useful for projects that require seamless integration with other Azure services and Microsoft applications, as well as built-in support for SSO, MFA, and conditional access.
3. IdentityServer4
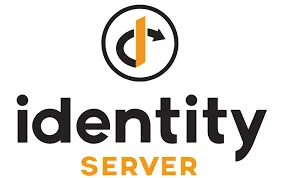
Pros:
- Open-source and widely-used identity and access management solution for .NET applications.
- Supports various identity protocols like OAuth 2.0, OpenID Connect, and SAML.
- Provides extensibility and customization options for different authentication and authorization scenarios.
- Offers a high level of control over the identity and access management process.
Cons:
- Can be complex to set up and configure, particularly for large-scale deployments.
- Lacks built-in support for some advanced features like MFA and conditional access, which may require additional implementation.
When to use: IdentityServer4 is a good choice for .NET applications that require a flexible and extensible identity and access management solution. It’s suitable for projects that need support for various identity protocols and a high level of control over the authentication and authorization process.
4. Okta
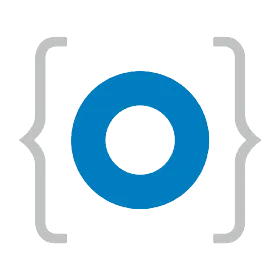
Pros:
- Offers a comprehensive and cloud-based identity and access management solution.
- Supports various identity protocols like OAuth 2.0, OpenID Connect, and SAML.
- Provides built-in support for single sign-on (SSO), multi-factor authentication (MFA), and conditional access.
- Integrates with a wide range of applications and services.
Cons:
- Can be more expensive compared to open-source alternatives.
- May require additional configuration and customization for specific use cases.
When to use: Okta is an excellent choice for organizations that need a comprehensive, cloud-based IAM solution. It’s particularly useful for projects that require seamless integration with various applications and services, as well as built-in support for SSO, MFA, and conditional access.
In summary, the choice of authentication and authorization tools depends on the specific requirements of your project. JWT offers a lightweight and stateless solution for handling claims, Azure AD provides a comprehensive IAM solution for the Microsoft ecosystem, IdentityServer4 delivers a flexible and extensible option for .NET applications, and Okta offers a powerful, cloud-based IAM solution that integrates with various applications and services.
VI. Task scheduling
1. Quartz.NET
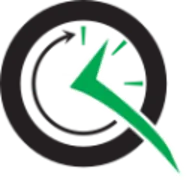
Pros:
- Offers a powerful and flexible task scheduling solution for .NET applications.
- Supports a wide range of scheduling options, including cron expressions, intervals, and calendars.
- Provides built-in support for job persistence, clustering, and failover.
- Extensible and customizable, with support for custom job factories and plugins.
Cons:
- Can be complex to set up and configure, particularly for large-scale deployments.
- May require additional resources and infrastructure for clustering and job persistence.
When to use: Quartz.NET is an excellent choice for .NET applications that require a powerful and flexible task scheduling solution. It’s particularly useful for projects that need advanced scheduling options, as well as built-in support for job persistence, clustering, and failover.
2. Hangfire
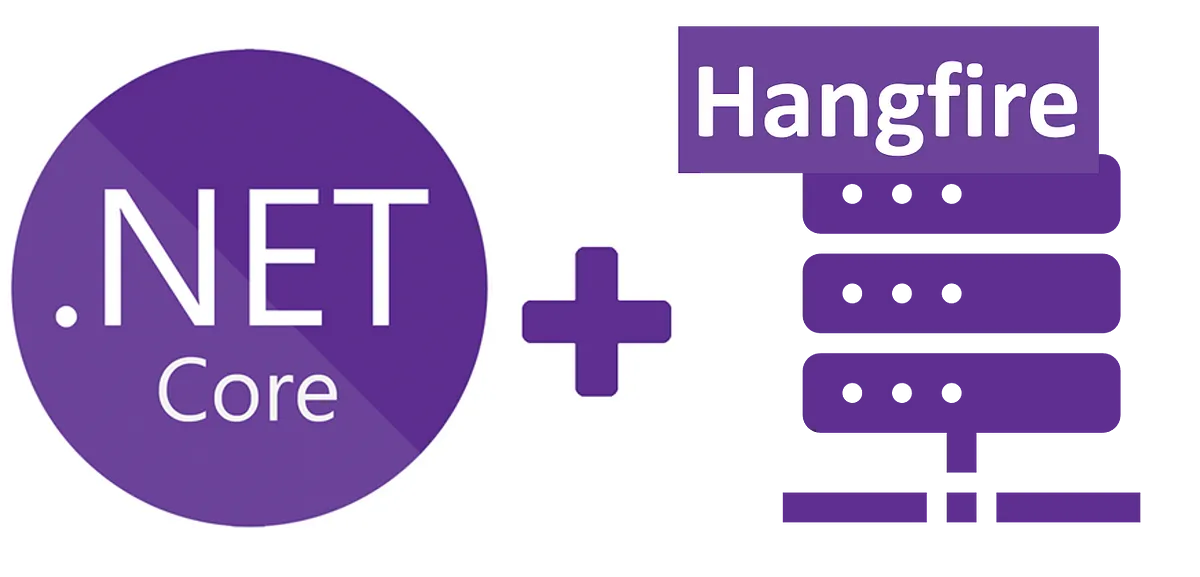
Pros:
- Offers a simple and easy-to-use task scheduling solution for .NET applications.
- Supports various job types, including fire-and-forget, delayed, recurring, and continuations.
- Provides built-in support for job persistence using various storage providers (e.g., SQL Server, Redis, PostgreSQL).
- Includes a web-based dashboard for monitoring and managing scheduled tasks.
Cons:
- Lacks some advanced scheduling features available in Quartz.NET, such as calendar-based scheduling.
- May not be suitable for projects that require high-precision scheduling or real-time processing.
When to use: Hangfire is a great choice for .NET applications that require a simple and easy-to-use task scheduling solution. It’s suitable for projects that need support for various job types and built-in job persistence, as well as a web-based dashboard for monitoring and managing tasks.
3. FluentScheduler
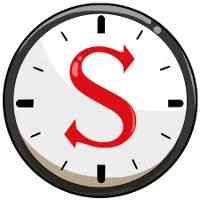
Pros:
- Offers a lightweight and easy-to-use task scheduling solution for .NET applications.
- Provides a fluent API for creating and managing scheduled tasks.
- Supports various scheduling options, including intervals and cron expressions.
- Does not require additional resources or infrastructure for job persistence or clustering.
Cons:
- Lacks built-in support for job persistence, clustering, and failover.
- May not be suitable for projects that require advanced scheduling features or large-scale deployments.
When to use: FluentScheduler is a good choice for small to medium-sized .NET applications that require a lightweight and easy-to-use task scheduling solution. It’s suitable for projects that don’t require advanced features like job persistence, clustering, or failover.
4. .NET Core Worker Service
Pros:
- Built-in .NET Core feature for creating long-running, background services.
- Offers a simple and native way to implement scheduled tasks in .NET Core applications.
- Easy to set up and integrate with other .NET Core features and libraries.
- Can be hosted as a Windows Service or a Linux daemon.
Cons:
- Limited to .NET Core applications.
- Lacks built-in support for job persistence, clustering, and failover.
- May require additional libraries or custom implementations for advanced scheduling features.
When to use: .NET Core Worker Service is a good choice for .NET Core applications that require a simple and native way to implement scheduled tasks. It’s suitable for projects that don’t require advanced features like job persistence, clustering, or failover and can benefit from tight integration with other .NET Core features and libraries.
In summary, the choice of task scheduling tools depends on the specific requirements of your project. Quartz.NET offers a powerful and flexible solution with advanced scheduling features, Hangfire provides a simple and easy-to-use option with built-in job persistence and a web-based dashboard, FluentScheduler delivers a lightweight and easy-to-use solution, and .NET Core Worker Service presents a native option for implementing scheduled tasks in .NET Core.
Other
AutoMapper
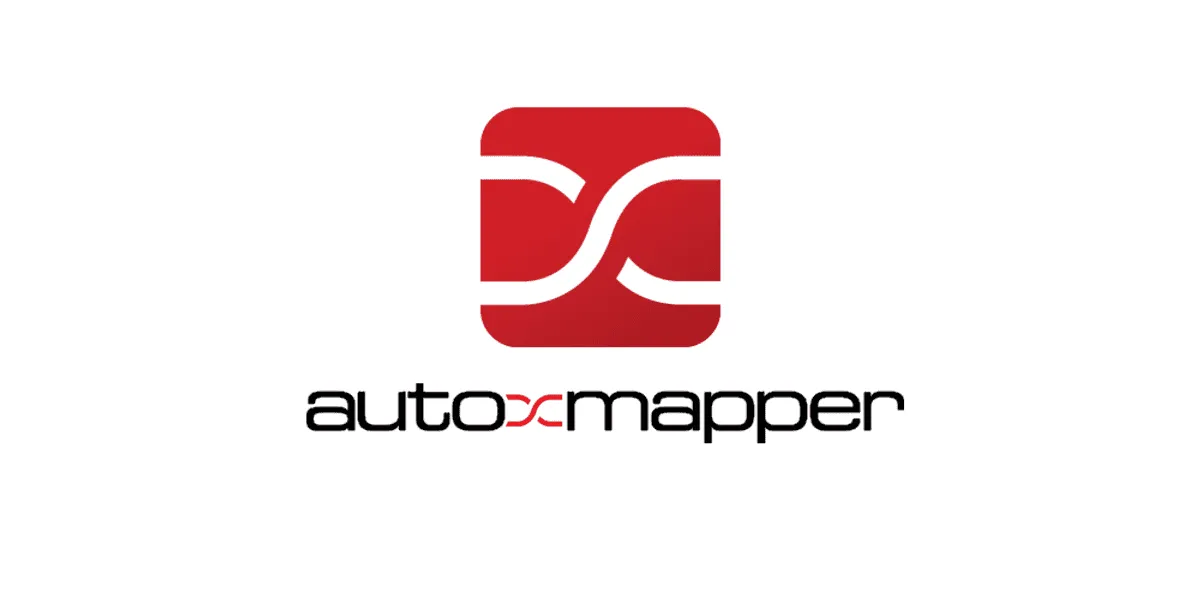
Pros:
- Offers a powerful and flexible object mapping solution for .NET applications.
- Reduces the amount of repetitive and error-prone code needed for object-to-object mapping.
- Provides a simple, convention-based configuration for common mapping scenarios.
- Supports advanced mapping scenarios, including custom value resolvers and type converters.
Cons:
- Can introduce performance overhead in some scenarios, particularly when mapping large collections or complex object graphs.
- May require additional configuration and customization for non-conventional mapping scenarios.
When to use: AutoMapper is an excellent choice for .NET applications that require a powerful and flexible object mapping solution. It’s particularly useful for projects that involve complex object-to-object mapping scenarios or that need to reduce repetitive mapping code.
MediatR
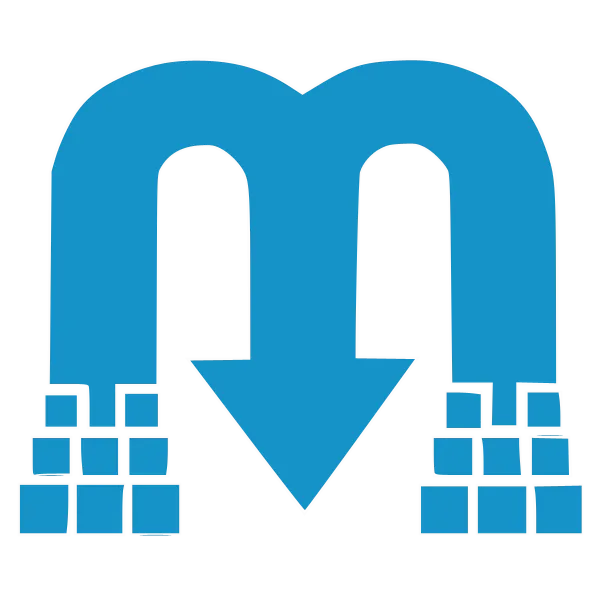
Pros:
- Offers a simple and easy-to-use implementation of the Mediator pattern for .NET applications.
- Helps to decouple components and promote a clean architecture by encapsulating requests and responses.
- Supports various types of message handlers, including request handlers, notification handlers, and pipeline behaviors.
- Can be easily integrated with popular dependency injection containers.
Cons:
- Introduces additional complexity and abstraction, which may not be suitable for small or simple projects.
- May require additional configuration and customization for advanced scenarios.
When to use: MediatR is a great choice for .NET applications that require a simple and easy-to-use implementation of the Mediator pattern. It’s suitable for projects that aim to promote a clean architecture and decouple components by encapsulating requests and responses.
Polly
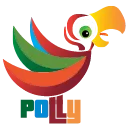
Pros:
- Offers a powerful and flexible resilience and transient-fault-handling library for .NET applications.
- Provides a wide range of policies, including retry, circuit breaker, fallback, and bulkhead isolation.
- Supports policy composition and customization for advanced resilience scenarios.
- Can be easily integrated with HttpClient and other popular .NET libraries.
Cons:
- Adds additional complexity and abstraction, which may not be suitable for small or simple projects.
- May require additional configuration and customization for advanced resilience scenarios.
When to use: Polly is an excellent choice for .NET applications that require a powerful and flexible resilience and transient-fault-handling library. It’s particularly useful for projects that involve communication with external services, databases, or APIs, where handling transient faults and implementing robust resilience strategies are crucial.
Dapper
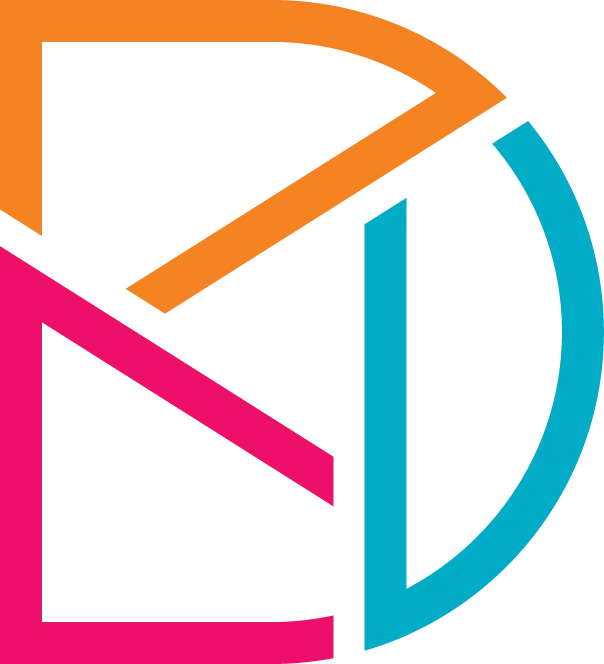
Pros:
- Offers a high-performance and easy-to-use micro-ORM for .NET applications.
- Provides a simple and straightforward API for executing SQL queries and mapping results to objects.
- Supports various database providers and connection types, including SQL Server, MySQL, and PostgreSQL.
- Can be easily integrated with popular .NET data access libraries, such as ADO.NET and Entity Framework.
Cons:
- Lacks some advanced ORM features, such as change tracking, lazy loading, and complex query generation.
- Requires developers to write raw SQL queries, which may not be suitable for some projects or teams.
When to use: Dapper is a good choice for .NET applications that require a high-performance and easy-to-use micro-ORM. It’s suitable for projects that prioritize performance over advanced ORM features and prefer writing raw SQL queries over using a full-fledged ORM like Entity Framework.
Conclusion
In summary, the choice of other tools and libraries depends on the specific requirements of your project. AutoMapper offers a powerful object mapping solution, MediatR provides a simple implementation of the Mediator pattern, Polly delivers a powerful resilience and transient-fault-handling library, and Dapper presents a high-performance micro-ORM for .NET applications.