In today’s fast-paced world of software development, it is crucial to be familiar with design patterns that can help you create robust, efficient, and maintainable code. One of the most widely used programming frameworks for enterprise applications is the .NET framework. In this article, we will explore the most commonly used design patterns in .NET development and how they can be applied to solve common problems encountered in software development.
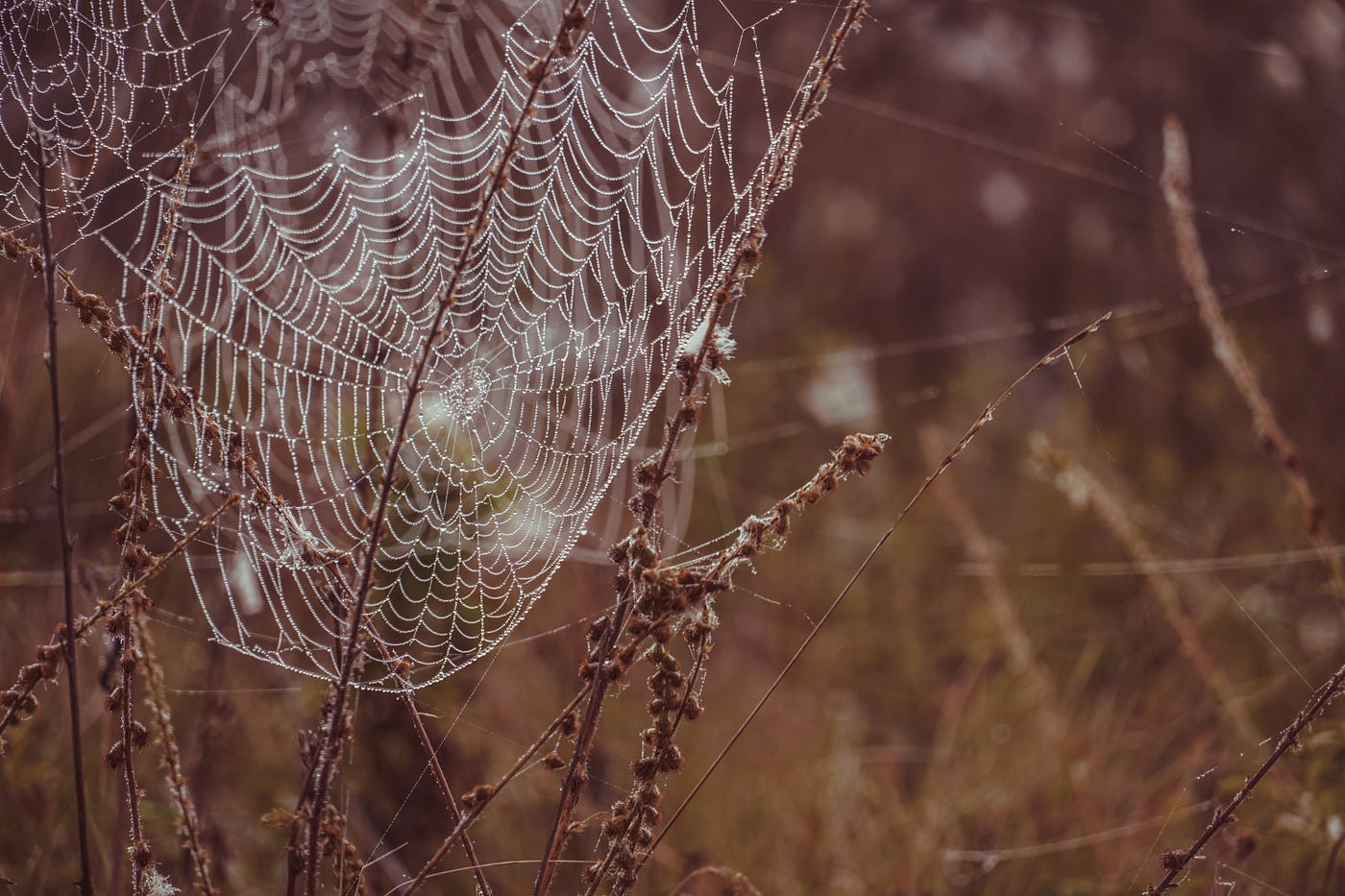
What are design patterns?
Before we dive into the most used design patterns in .NET development, let’s first understand what design patterns are. Design patterns are a set of best practices and solutions to common problems that software developers face during the development process. These patterns are usually reusable, and can be applied to different projects.
Design patterns are not a language feature or a library, but rather a way of organizing code to make it more maintainable, extensible, and reusable. They are usually categorized into three types: creational patterns, structural patterns, and behavioral patterns.
Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Structural patterns deal with object composition, and behavioral patterns deal with communication between objects.
Creational design patterns
Creational design patterns are used to create objects in a way that is suitable for a particular situation. The most commonly used creational design patterns in .NET development are:
1. Singleton pattern
The Singleton pattern is used to ensure that a class has only one instance, and it provides a global point of access to that instance. In .NET, the Singleton pattern is implemented using a private constructor and a static field that holds the single instance of the class. This pattern is useful when you want to ensure that only one instance of a class is created, and you want to provide a global point of access to that instance.
public sealed class Singleton
{
private static Singleton instance = null;
private static readonly object lockObject = new object();
private Singleton() {}
public static Singleton Instance
{
get
{
lock(lockObject)
{
if (instance == null)
{
instance = new Singleton();
}
}
return instance;
}
}
}
2. Factory pattern
The Factory pattern is used to create objects without exposing the creation logic to the client. In .NET, the Factory pattern is implemented using a class or a method that creates objects based on a set of parameters. This pattern is useful when you want to create objects based on a particular set of conditions, and you want to hide the creation logic from the client.
public interface IAnimalFactory
{
IAnimal Create();
}
public class DogFactory : IAnimalFactory
{
public IAnimal Create()
{
return new Dog();
}
}
public class CatFactory : IAnimalFactory
{
public IAnimal Create()
{
return new Cat();
}
}
public interface IAnimal
{
string Speak();
}
public class Dog : IAnimal
{
public string Speak()
{
return "Woof!";
}
}
public class Cat : IAnimal
{
public string Speak()
{
return "Meow!";
}
}
3. Builder pattern
The Builder pattern is used to separate the construction of a complex object from its representation. In .NET, the Builder pattern is implemented using a separate class or method that constructs the object step by step. This pattern is useful when you want to create complex objects that have many components, and you want to separate the construction logic from the representation.
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
public string Address { get; set; }
}
public class PersonBuilder
{
private readonly Person person;
public PersonBuilder()
{
person = new Person();
}
public PersonBuilder WithFirstName(string firstName)
{
person.FirstName = firstName;
return this;
}
public PersonBuilder WithLastName(string lastName)
{
person.LastName = lastName;
return this;
}
public PersonBuilder WithAge(int age)
{
person.Age = age;
return this;
}
public PersonBuilder WithAddress(string address)
{
person.Address = address;
return this;
}
public Person Build()
{
return person;
}
}
4. Dependency Injection pattern
The Dependency Injection pattern is a creational pattern that provides a way to create objects without having to know the details of how they are constructed. This is useful when you need to create objects that have complex dependencies or are difficult to instantiate.
public interface IDataAccessLayer
{
void SaveData(string data);
}
public class SqlDataAccessLayer : IDataAccessLayer
{
public void SaveData(string data)
{
// Implementation of saving data in SQL Server
}
}
public class BusinessLogicLayer
{
private readonly IDataAccessLayer _dataAccessLayer;
public BusinessLogicLayer(IDataAccessLayer dataAccessLayer)
{
_dataAccessLayer = dataAccessLayer;
}
public void SaveData(string data)
{
_dataAccessLayer.SaveData(data);
}
}
Structural design patterns
Structural design patterns are used to define the relationships between objects and how they can be composed to form larger structures. The most commonly used structural design patterns in .NET development are:
1. Adapter pattern
The Adapter pattern is used to convert the interface of a class into another interface that clients expect. In .NET, the Adapter pattern is implemented using a separate class that acts as a bridge between two incompatible interfaces. This pattern is useful when you want to use a class that is not compatible with the existing codebase, and you want to convert its interface to make it compatible.
public interface ITarget
{
void Request();
}
public class Adaptee
{
public void SpecificRequest()
{
Console.WriteLine("Specific request");
}
}
public class Adapter : ITarget
{
private readonly Adaptee _adaptee;
public Adapter(Adaptee adaptee)
{
_adaptee = adaptee;
}
public void Request()
{
_adaptee.SpecificRequest();
}
}
2. Decorator pattern
The Decorator pattern is used to add functionality to an object dynamically. In .NET, the Decorator pattern is implemented using a separate class that wraps the original object and provides additional functionality. This pattern is useful when you want to add functionality to an object without changing its interface.
public interface ICoffee
{
string GetDescription();
double GetCost();
}
public class SimpleCoffee : ICoffee
{
public string GetDescription()
{
return "Simple Coffee";
}
public double GetCost()
{
return 1.0;
}
}
public class CoffeeWithMilk : ICoffee
{
private readonly ICoffee _coffee;
public CoffeeWithMilk(ICoffee coffee)
{
_coffee = coffee;
}
public string GetDescription()
{
return _coffee.GetDescription() + ", with milk";
}
public double GetCost()
{
return _coffee.GetCost() + 0.5;
}
}
3. Facade pattern
The Facade pattern is used to provide a simple interface to a complex system. In .NET, the Facade pattern is implemented using a separate class that provides a simplified interface to the existing system. This pattern is useful when you want to simplify the interaction with a complex system, and you want to hide its complexity from the client.
// Complex subsystem with many parts
public class Subsystem1
{
public void Operation1()
{
Console.WriteLine("Subsystem1: Operation1");
}
public void Operation2()
{
Console.WriteLine("Subsystem1: Operation2");
}
}
public class Subsystem2
{
public void Operation3()
{
Console.WriteLine("Subsystem2: Operation3");
}
public void Operation4()
{
Console.WriteLine("Subsystem2: Operation4");
}
}
public class Subsystem3
{
public void Operation5()
{
Console.WriteLine("Subsystem3: Operation5");
}
public void Operation6()
{
Console.WriteLine("Subsystem3: Operation6");
}
}
// Facade that simplifies the interface to the subsystem
public class Facade
{
private readonly Subsystem1 _subsystem1;
private readonly Subsystem2 _subsystem2;
private readonly Subsystem3 _subsystem3;
public Facade()
{
_subsystem1 = new Subsystem1();
_subsystem2 = new Subsystem2();
_subsystem3 = new Subsystem3();
}
public void Operation()
{
_subsystem1.Operation1();
_subsystem2.Operation4();
_subsystem3.Operation6();
}
}
4. Bridge pattern
The Bridge pattern is used to decouple an abstraction from its implementation, allowing them to vary independently. It provides a way to create a family of related classes with different implementations. This pattern is particularly useful when we have multiple variations of a class that we want to use interchangeably.
public interface IImplementor
{
void OperationImpl();
}
public class ConcreteImplementorA : IImplementor
{
public void OperationImpl()
{
Console.WriteLine("Concrete Implementor A");
}
}
public class ConcreteImplementorB : IImplementor
{
public void OperationImpl()
{
Console.WriteLine("Concrete Implementor B");
}
}
public abstract class Abstraction
{
protected IImplementor _implementor;
public Abstraction(IImplementor implementor)
{
_implementor = implementor;
}
public virtual void Operation()
{
_implementor.OperationImpl();
}
}
public class RefinedAbstraction : Abstraction
{
public RefinedAbstraction(IImplementor implementor) : base(implementor)
{
}
public override void Operation()
{
_implementor.OperationImpl();
}
}
Behavioral design patterns
1. Observer pattern
The Observer Pattern is a behavioral design pattern that allows an object, called the subject, to notify a list of observers when its state changes. The observers are then automatically notified and updated. A typical example of this pattern is a news agency that notifies its subscribers when a new article is published.
public interface IObserver
{
void Update();
}
public interface ISubject
{
void Attach(IObserver observer);
void Detach(IObserver observer);
void Notify();
}
public class ConcreteSubject : ISubject
{
private List<IObserver> _observers = new List<IObserver>();
private string _state;
public string State
{
get { return _state; }
set
{
_state = value;
Notify();
}
}
public void Attach(IObserver observer)
{
_observers.Add(observer);
}
public void Detach(IObserver observer)
{
_observers.Remove(observer);
}
public void Notify()
{
foreach (IObserver observer in _observers)
{
observer.Update();
}
}
}
public class ConcreteObserver : IObserver
{
private string _name;
private string _observerState;
private ConcreteSubject _subject;
public ConcreteObserver(ConcreteSubject subject, string name)
{
_subject = subject;
_name = name;
}
public void Update()
{
_observerState = _subject.State;
Console.WriteLine($"Observer {_name}'s new state is {_observerState}");
}
}
public static void Main()
{
ConcreteSubject subject = new ConcreteSubject();
ConcreteObserver observerA = new ConcreteObserver(subject, "A");
ConcreteObserver observerB = new ConcreteObserver(subject, "B");
ConcreteObserver observerC = new ConcreteObserver(subject, "C");
subject.Attach(observerA);
subject.Attach(observerB);
subject.Attach(observerC);
subject.State = "New State";
}
2. Command pattern
The Command Pattern is a behavioral design pattern that encapsulates a request as an object, thereby allowing for the parameterization of clients with different requests, queues, and log requests, and supports undoable operations. A typical example of this pattern is a remote control for a TV, where each button press corresponds to a different command.
public interface ICommand
{
void Execute();
}
public class Receiver
{
public void Action()
{
Console.WriteLine("Receiver does some action");
}
}
public class ConcreteCommand : ICommand
{
private Receiver _receiver;
public ConcreteCommand(Receiver receiver)
{
_receiver = receiver;
}
public void Execute()
{
_receiver.Action();
}
}
public class Invoker
{
private ICommand _command;
public void SetCommand(ICommand command)
{
_command = command;
}
public void ExecuteCommand()
{
_command.Execute();
}
}
public static void Main()
{
Invoker invoker = new Invoker();
Receiver receiver = new Receiver();
ConcreteCommand command = new ConcreteCommand(receiver);
invoker.SetCommand(command);
invoker.ExecuteCommand();
}
3. Strategy pattern
The Strategy Pattern is a behavioral design pattern that allows a client to choose from a family of algorithms at runtime. This pattern is useful when there are multiple algorithms that can be used to solve a problem, and the choice of algorithm depends on the context. A typical example of this pattern is a sorting algorithm that can be chosen based on the size of the input data.
public interface IStrategy
{
void AlgorithmInterface();
}
public class ConcreteStrategyA : IStrategy
{
public void AlgorithmInterface()
{
Console.WriteLine("ConcreteStrategyA.AlgorithmInterface()");
}
}
public class ConcreteStrategyB : IStrategy
{
public void AlgorithmInterface()
{
Console.WriteLine("ConcreteStrategyB.AlgorithmInterface()");
}
}
public class Context
{
private IStrategy _strategy;
public Context(IStrategy strategy)
{
_strategy = strategy;
}
public void ContextInterface()
{
_strategy.AlgorithmInterface();
}
}
public static void Main()
{
Context context;
context = new Context(new ConcreteStrategyA());
context.ContextInterface();
context = new Context(new ConcreteStrategyB());
context.ContextInterface();
}
Conclusion
In conclusion, understanding and using design patterns in your .NET development can greatly improve the quality, scalability, and maintainability of your code. In this article, we have discussed the most commonly used design patterns in .NET development, but there are many more not covered here. By incorporating these patterns into your code, you can write high-quality software that is easy to maintain, extend, and modify over time.