Во время разработки иногда нужно знать состояние объекта и какие данные в нём находятся. Debug не всегда подходит, иногда удобнее смотреть состояние объекта на веб-странице или может возникнуть необходимость сохранить информацию, например в файл.
Есть множество решений как реализовать эту задачу, и одно из простых и эффективных это написать Extension
:
public static class Extensions
{
public static string ObjectProperties(this object obj)
{
var properties = obj.GetType().GetProperties();
var dump = new StringBuilder();
foreach (var prop in properties)
{
dump.AppendLine($"{prop.Name}: {prop.GetValue(obj, null)}");
}
return dump.ToString();
}
}
Вариант получения списка свойств:
public static class Extensions
{
public static IList<string> ObjectPropertiesList(this object obj)
{
var properties = obj.GetType().GetProperties();
return properties.Select(prop => $"{prop.Name}: {prop.GetValue(obj, null)}").ToList();
}
}
Это рабочий вариант, но не показывает свойства вложенных объектов. В этой задаче нет необходимости показывать данные на множестве уровней вложенности, достаточно узнать про первый уровень.
public static IEnumerable<string> ObjectPropertiesFull(this object obj)
{
var dump = new List<string>();
var properties = obj.GetType().GetProperties();
foreach (var prop in properties)
{
if (prop.GetValue(obj, null) is ICollection collectionItems)
{
dump.Add(prop.Name);
foreach (var item in collectionItems)
{
dump.Add($" - {item}");
var objectType = item.GetType();
foreach (var nestedObjectProp in objectType.GetProperties())
{
dump.Add($" --- {nestedObjectProp.Name}: {nestedObjectProp.GetValue(item)}");
}
}
}
else if (prop.GetValue(obj, null) is Object nestedObject)
{
dump.Add($"{prop.Name}: {prop.GetValue(obj, null)}");
var nestedType = nestedObject.GetType();
foreach (var nestedTypeProp in nestedType.GetProperties())
{
dump.Add($" --- {nestedTypeProp.Name}: {nestedTypeProp.GetValue(nestedObject)}");
}
}
}
return dump;
}
Этого в большинстве случаев достаточно. Если возникнет необходимость узнать больше информации об объекте - можно использовать рекурсию и более глубокий анализ свойств.
Codebase: https://codebase.blackball.lv/project/code-buns/source?file=Extensions/ObjectExtensions.cs#code-buns
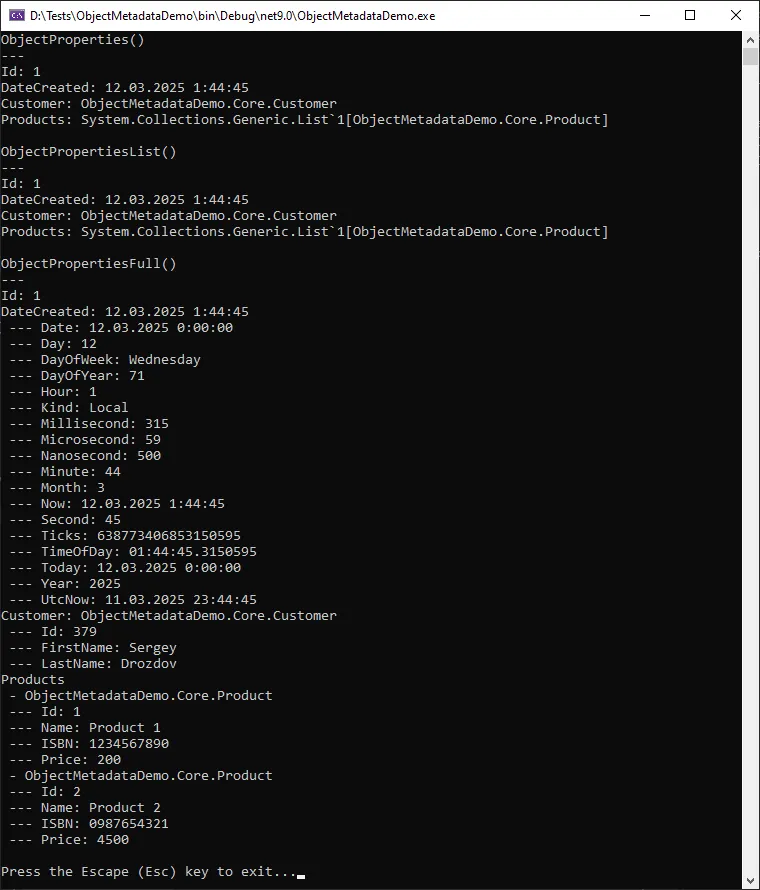