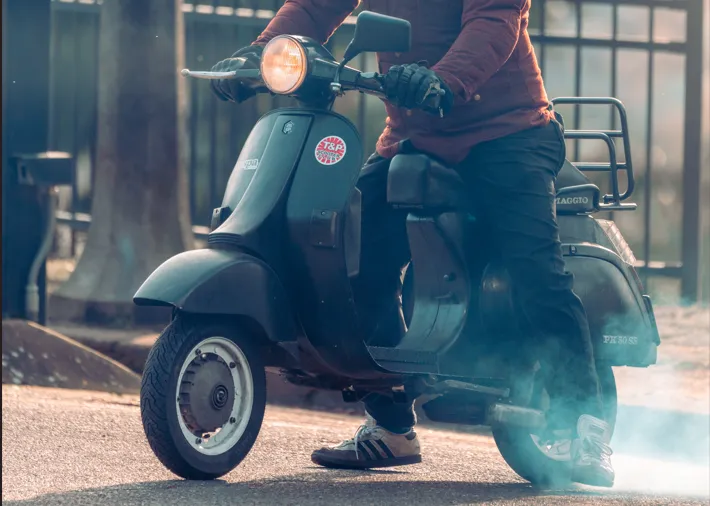
Look at that power
Imagine building a house. View components are like the pre-built sections of a house, such as the windows, doors, and cabinets, which are designed to fit into the larger structure easily. You can swap them out or change their appearance without needing to rebuild the whole house.
For example, a shopping basket in a web application is like a pre-built kitchen cabinet that you can place in any room. You can customize its size and appearance, but the functionality remains consistent, allowing you to store items. Similarly, a login panel is like a door with a lock, which can be added to any room, granting access only to those with the right key.
Using view components, developers can construct a web application like a house, with each pre-built section fitting seamlessly into the larger structure. This approach saves time, reduces complexity, and allows for more efficient maintenance and updates. The only downfall to View Components is the act of not using them.
What makes a View Component?
A view component is a class that meets one of the following criteria: its name ends with “ViewComponent,” it defines an “Invoke” or “InvokeAsync” method, it inherits from the “ViewComponent” base class, or it has the “ViewComponent” attribute applied to it. You can place View Component files anywhere in your project, but the convention is to hold them in a folder named Components located at Solution/Components.
Let’s see a View Component in action.
Here’s a quick follow along to make a View Component at its most fundamental level. It is only 6 steps and should give you an understanding of how the folder structures are arranged and how View Components are added to Views.
This View Component only displays a simple string message just so we can streamline the setup process.
1. In Visual Studio, make a new ASP.NET Core Web App (Model-View-Controller) application named ‘ViewComponentExample’ I am using NET 6.0 but other versions should be just fine.
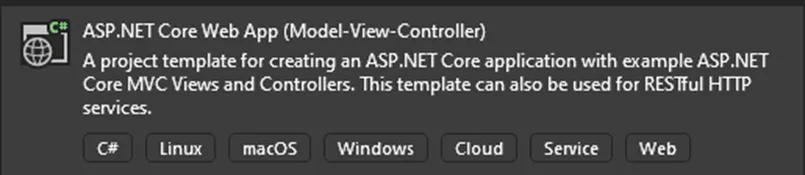
Pick this template.
2. Inside your main project folder, create a folder named Components with a new class inside of it named MyViewComponents.cs so the folder structure looks like this:
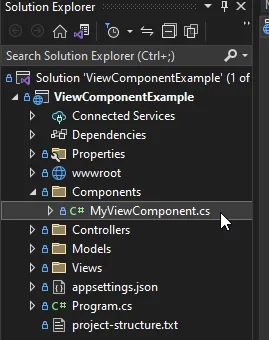
Folder Structure Pit Stop
3. Add the following code to your MyViewComponents.cs
class
using Microsoft.AspNetCore.Mvc;
using ViewComponentExample.Models;
namespace ViewComponentExample.Components
{
public class MyViewComponents : ViewComponent
{
public IViewComponentResult Invoke()
{
return View("Default");
}
}
}
4. In the Solution Explorer, create a folder in the Views\Shared folder named Components and then create a folder inside of the Components folder named MyViewComponent like this:
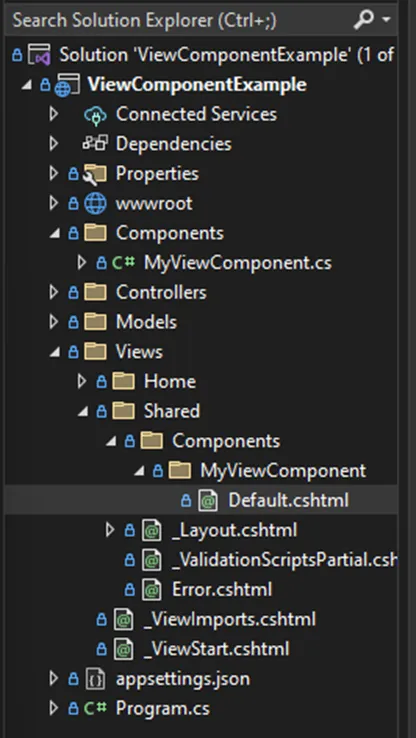
We are going to add Default.cshtml in the next step.
5. Right-click the “MyViewComponent” folder, select “Add” > “View,”, Razor View — Empty and name the new view “Default.cshtml”. Which is the naming convention for all Razor View Components. Replace all the contents with the following code:
@model string
<div>
@Model
</div>
6. Open the Views/Home/Index.cshtml file and replace all the content with the following code.
@{
ViewData["Title"] = "Home Page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
<p>Learn about <a href="https://docs.microsoft.com/aspnet/core">building Web apps with ASP.NET Core</a>.</p>
@await.InvokeAsync(nameof(ViewComponentExample.Components.MyViewComponent))
</div>
7. Run the application to see this string result:
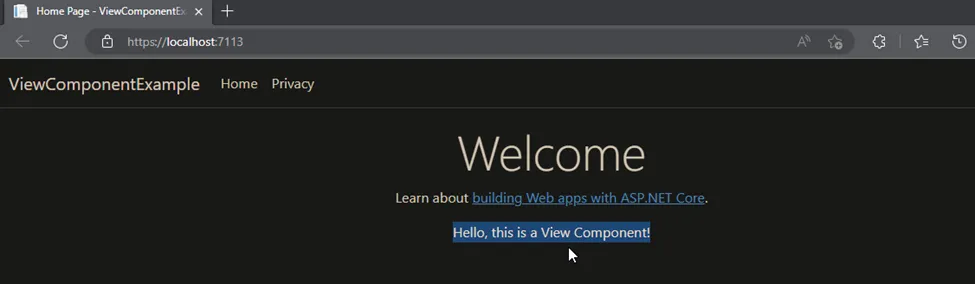
Notice that all we added to Index.cshtml was this line of code
@await.InvokeAsync(nameof(ViewComponentExample.Components.MyViewComponent))
which we can now add to any View in our application. Pretty cool right?
While inserting simple string values into a view or page is not particularly useful, view components can offer more capabilities. By returning an object that implements the IViewComponentResult interface from the Invoke or InvokeAsync method, more complex effects can be achieved.
From here, we only need to make three tiny code changes in to expand its capability.
1. Create a class in the Models folder named MyViewModel.cs with the following content:
public class MyViewModel
{
public string Message { get; set; }
public int Count { get; set; }
}
The structure of your folders and new class should look like this.
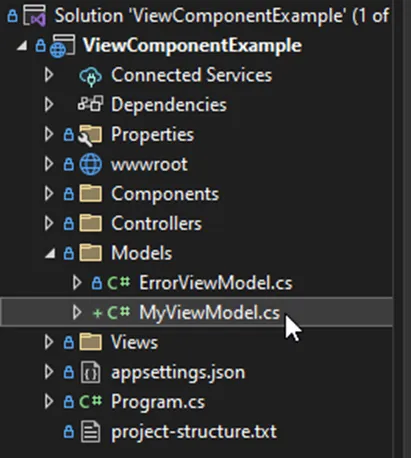
2. Expand your Components folder to open and update the code in your MyViewComponent.cs class to the following.
using Microsoft.AspNetCore.Mvc;
using ViewComponentExample.Models;
namespace ViewComponentExample.Components
{
[ViewComponent(Name = "MyViewComponent")]
public class MyViewComponent : ViewComponent
{
public IViewComponentResult Invoke()
{
var model = new MyViewModel
{
Message = "Hello, this is a View Component!",
Count = 5 // Or whatever value you need
// Initialize other properties here
};
return View("Default", model);
}
}
}
3. Finally, update the Default.cshtml view component file to these contents.
@model MyViewModel
<div>
<p>@Model.Message</p>
<p>Count: @Model.Count</p>
<!-- Display other properties here -->
</div>
Once you run the application you should get this output.
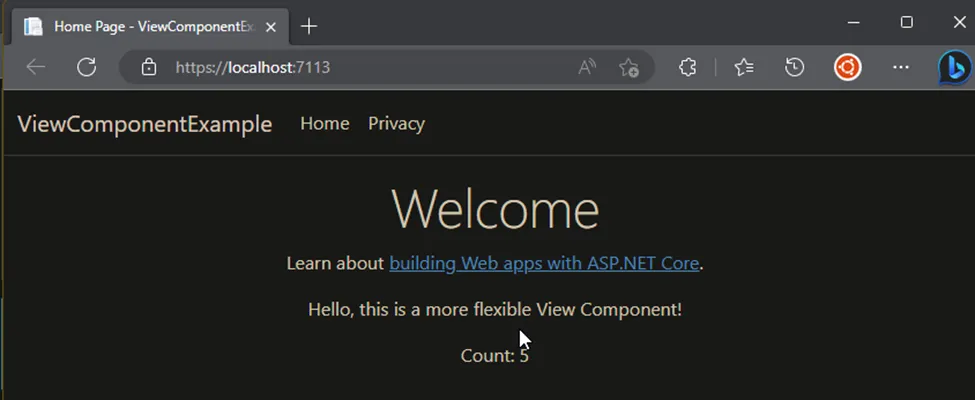
In the initial setup, the View Component was returning a simple string, which limited the complexity of data we could display in our view. Since we added the MyViewModel class, we can now pass a complex object from the View Component to the view. This is accomplished by the Invoke method of MyViewComponent.cs where an instance of MyViewModel.cs is created and returned to the view. This means our sample application can now handle more complex data structures, and benefit from compile-time type checking, which helps prevent type-related errors.
Before we wrap up this article, I want to describe three similar ways that programmers might create something similar to a View Component.
Blazor Components: Blazor is also all about components, like Angular, React, and Vue.js. But here’s the cool part — Blazor components can either run on the server with UI updates sent over SignalR, or they can run right in the client’s web browser using WebAssembly. This gives you the perks of server-side programming, like full .NET debugging and no need to make API calls for data, while still giving users a slick, interactive experience. The downside? Blazor is still pretty new and doesn’t have as much community support or as many third-party libraries as the others.
Partial Views and View Components in ASP.NET Core: These two let you make reusable pieces of UI in ASP.NET Core, but they’re used differently. Partial Views are the simple ones — they can’t do logic or fetch data. They’re just pieces of Razor views that you can slot into other views, kind of like reusable sections for things like headers, footers, or navigation menus.
View Components, on the other hand, are the heavy lifters. They can have their own actions, fetch data, perform operations, and can return a result that’s rendered in the view. This makes them great for bits of the UI that need complex logic or data access, like a shopping cart, dynamic navigation menus, or widgets that rely on data. The trade-off is that they’re a bit more complicated to set up and use than Partial Views.
So, in the end, while these different frameworks and features all have a similar concept of components, they vary a lot in what they can do, how they’re used, and how they’re implemented. Choosing between them usually comes down to what your project needs, what your team is good at, and what tech stack you’re working with in your application.