Concurrency issues are common in any multi-user environment, especially when multiple users attempt to modify the same data simultaneously. In high-traffic applications, where thousands of users or processes may be reading or updating data at the same time, ensuring data consistency becomes even more critical. Without effective concurrency control, race conditions or stale data updates can lead to significant problems, undermining the integrity of your system.
Entity Framework Core (EF Core) offers powerful tools to address these challenges, helping developers implement robust concurrency management strategies. This article will explore the importance of concurrency control, why it matters in high-traffic environments, and how to effectively implement it in EF Core to ensure both performance and scalability along with maintaining data integrity even under the heavy load.
1. Understanding concurrency in EF Core
Concurrency refers to situations where multiple processes or users attempt to update the same data in a database simultaneously. In the absence of proper concurrency control, conflicting updates can lead to data corruption or loss, where one user’s changes overwrite another’s without either realizing it.
The two common types of concurrency control are:
- Pessimistic Concurrency: Locks data until a transaction completes.
- Optimistic Concurrency: Assumes conflicts are rare and only checks for them when updating data.
In EF Core, optimistic concurrency control is the default mechanism, as it avoids unnecessary locking, improving application performance — an essential consideration for high-traffic applications.
2. Challenges of high-traffic applications
Concurrency challenges in high-traffic applications arise because multiple users frequently attempt to interact with the same records. Key issues include:
- Race Conditions: When two transactions try to modify the same data, the last update can overwrite the first one without detection.
- Lost Updates: Data can be silently overwritten if concurrency control is not properly handled.
- Increased Conflict Frequency: More users mean more chances of data conflicts, making robust conflict resolution critical.
- Scalability: Locking mechanisms (as in pessimistic concurrency) can hinder performance in high-traffic scenarios, leading to bottlenecks.
EF Core’s optimistic concurrency approach is ideal in high-traffic systems, as it ensures high performance without holding database locks for extended periods.
3. Types of concurrency conflicts
Concurrency conflicts in EF Core typically fall into two categories:
- Lost Updates: One user’s changes overwrite another’s.
- Phantom Reads: Data changes between reading and writing, leading to inconsistent results.
EF Core’s optimistic concurrency control aims to prevent lost updates by detecting conflicting changes during the SaveChanges
operation.
4. Implementing concurrency tokens in EF Core
EF Core uses concurrency tokens to detect if another user has modified a record before an update. A typical concurrency token is a field in the database that is checked before applying updates.
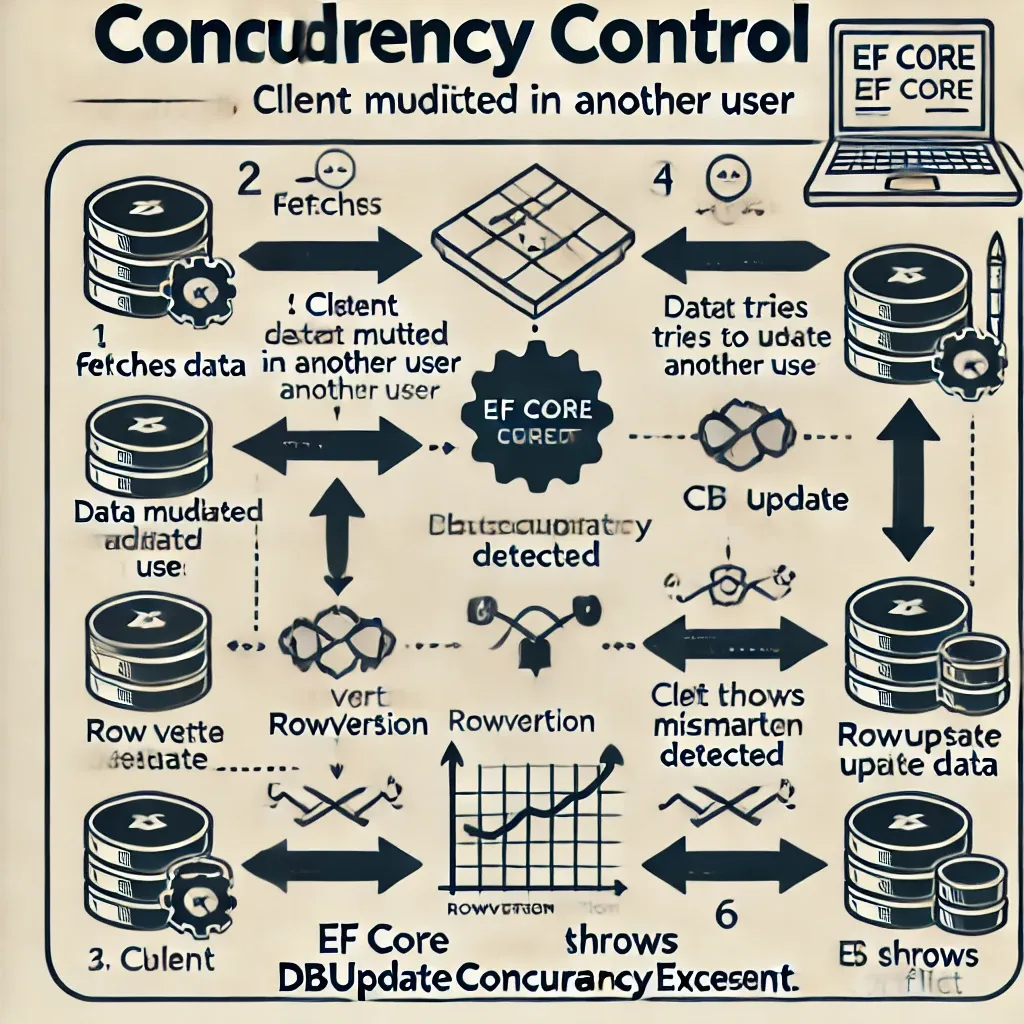
Timestamp/RowVersion example
The most common concurrency token is a RowVersion
or Timestamp
column. This column is automatically updated by the database every time a record is modified.
Step-by-step implementation
1. Add the Concurrency Token
In your model, add a byte[]
property for the RowVersion
. EF Core will automatically treat it as a concurrency token.
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
// Concurrency token
[Timestamp]
public byte[] RowVersion { get; set; }
}
2. Configure the model
By adding the [Timestamp]
attribute, EF Core marks this field as a concurrency token. Alternatively, you can configure it using the Fluent API:
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<Product>()
.Property(p => p.RowVersion)
.IsRowVersion();
}
5. Handling concurrency conflicts gracefully
Handling concurrency conflicts in a high-traffic application requires a careful balance between user experience and data consistency.
When EF Core detects that the RowVersion
in the database has changed since the data was loaded, it throws a DbUpdateConcurrencyException
. You can catch this exception and handle it appropriately, such as by informing the user of the conflict.
try
{
context.SaveChanges();
}
catch (DbUpdateConcurrencyException ex)
{
foreach (var entry in ex.Entries)
{
var clientValues = entry.Entity;
var databaseValues = entry.GetDatabaseValues();
if (databaseValues == null)
{
// Entity was deleted by another user
Console.WriteLine("The entity has been deleted.");
}
else
{
// Handle conflict
var dbEntity = databaseValues.ToObject();
Console.WriteLine($"Database version: {dbEntity}");
}
}
}
In high-traffic systems, you need to ensure that the user is informed about the conflict and possibly allowed to choose how to proceed.
In this scenario, you decide how to resolve the conflict. You could:
- Retry the update.
- Merge the user’s and the database’s changes.
- Let the user choose which version to keep.
6. Handling concurrency in different scenarios
6.1 Pessimistic concurrency control
While EF Core uses optimistic concurrency by default, you may occasionally need pessimistic concurrency, which locks the data while a transaction is in progress. Although EF Core doesn’t natively support this, you can execute raw SQL queries for operations that require explicit locks:
await context.Database.ExecuteSqlRawAsync("SELECT * FROM Products WITH (XLOCK, ROWLOCK) WHERE Id = {0}", productId);
This ensures no other transaction can modify the record until your operation completes.
6.2 Resolving conflicts in a multi-tiered architecture
In distributed systems or applications with multiple clients, resolving concurrency conflicts can be more challenging. Strategies include:
- Client-Side Merging: Present both the client’s and the server’s versions of the data to the user for manual merging.
- Retry Logic: Automatically retry the transaction if a conflict occurs.
- Conflict-Free Replicated Data Types (CRDTs): For specific use cases like collaborative editing, you may explore advanced techniques like CRDTs, which allow concurrent updates without conflicts.
7. Advanced concurrency resolution strategies for high-traffic apps
When dealing with high-traffic applications, you must adopt specific strategies to resolve concurrency conflicts efficiently without causing unnecessary delays or overwhelming the system. Let’s examine three key approaches:
7.1 Client wins strategy (Overwriting data)
In this approach, the client’s version of the data takes precedence over the database’s. This method is simple and avoids repeated conflict resolutions but risks overwriting other users’ changes.
entry.OriginalValues.SetValues(databaseValues);
context.SaveChanges();
This strategy is useful for cases where user-specific data is prioritized, but in a high-traffic system, it can lead to lost updates if not handled carefully.
7.2 Database wins strategy (Discarding client changes)
Here, the data in the database is considered the source of truth. The client’s changes are discarded, and the latest database values are reloaded.
entry.Reload();
context.SaveChanges();
This strategy is effective when preserving the most up-to-date information is essential, especially for applications dealing with sensitive or critical data.
7.3 Merge data strategy (Hybrid approach)
A hybrid approach merges the conflicting data, allowing you to selectively combine values from both the client and the database. This can be complex but is often the most user-friendly solution, especially in collaborative environments.
// Update the client’s values with only selective database values
entry.OriginalValues.SetValues(databaseValues);
entry.CurrentValues["SomeProperty"] = clientValues["SomeProperty"];
context.SaveChanges();
For high-traffic applications, it’s vital to adopt a flexible conflict resolution strategy that meets the business needs without overwhelming the system with retries or unresolved conflicts.
8. Concurrency control best practices
8.1 Use RowVersion wisely
The RowVersion
token is very effective but may not be necessary for all entities. Only apply it where multiple users are likely to modify the same record simultaneously.
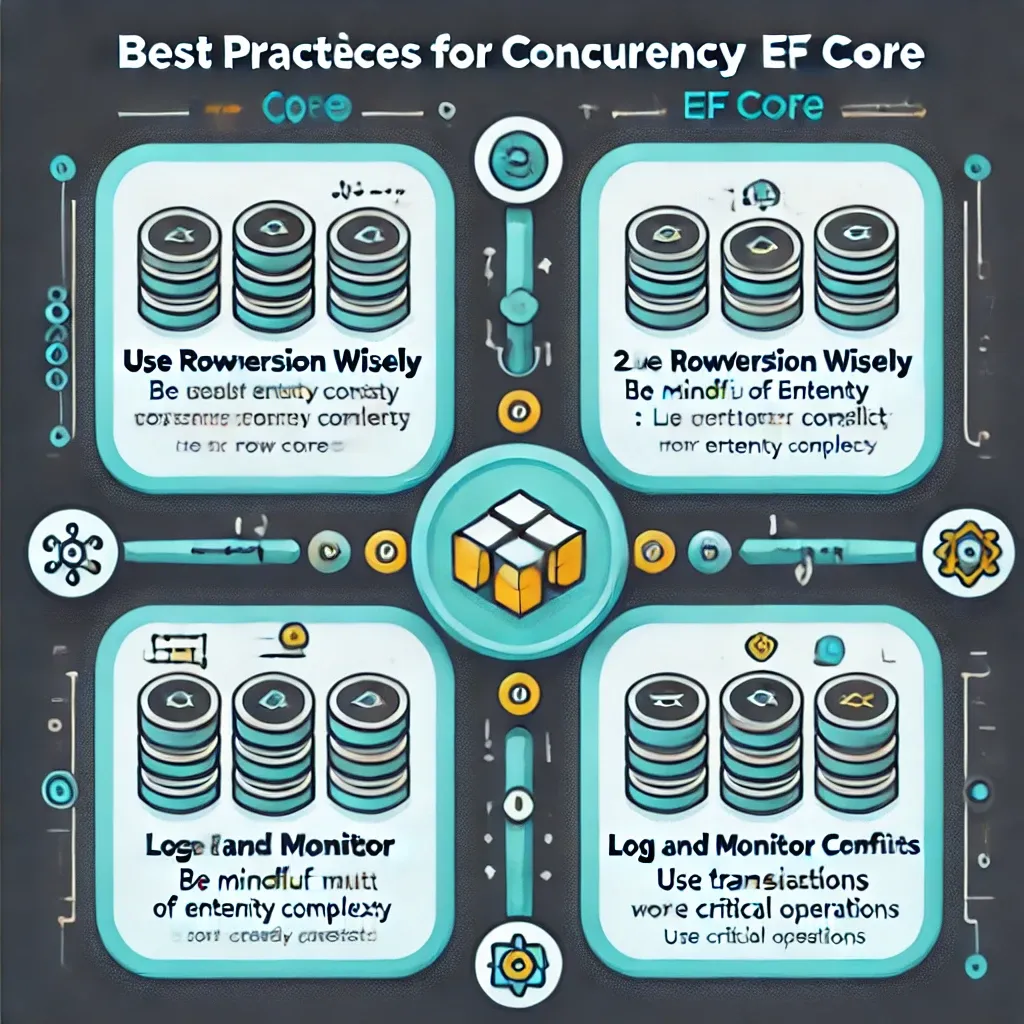
8.2 Be mindful of entity complexity
If your entity is too complex or the frequency of updates is high, you might face many conflicts. In such cases, consider splitting your entity into smaller, more manageable pieces to reduce contention.
8.3 Log and monitor conflicts
Concurrency conflicts provide valuable insight into how your application is being used. Logging these events helps identify hotspots where data contention occurs and whether more sophisticated concurrency management strategies are required.
8.4 Use transactions for critical operations
For operations where data integrity is crucial, such as financial transactions, ensure you use proper transaction handling, especially if multiple records are involved. EF Core supports transactions through the DbContext.Database.BeginTransaction
method:
using (var transaction = await context.Database.BeginTransactionAsync())
{
try
{
// Perform your operations
await context.SaveChangesAsync();
await transaction.CommitAsync();
}
catch (Exception)
{
await transaction.RollbackAsync();
}
}
Conclusion
Concurrency handling is crucial in high-traffic applications to maintain data consistency and prevent conflicts. EF Core’s optimistic concurrency control and concurrency tokens provide scalable solutions to manage these challenges efficiently. By mastering concurrency tokens, handling exceptions like DbUpdateConcurrencyException
, and using resolution strategies like "Client Wins" or "Database Wins," you can ensure data integrity while keeping your application performant under heavy load.
EF Core’s tools, such as RowVersion columns, help avoid unnecessary locking and streamline conflict resolution. By following best practices, you can ensure your application remains robust, scalable, and responsive in multi-user environments.