Exploring Key Features, Use Cases, and Performance of Two Popular Real-time Communication Protocols
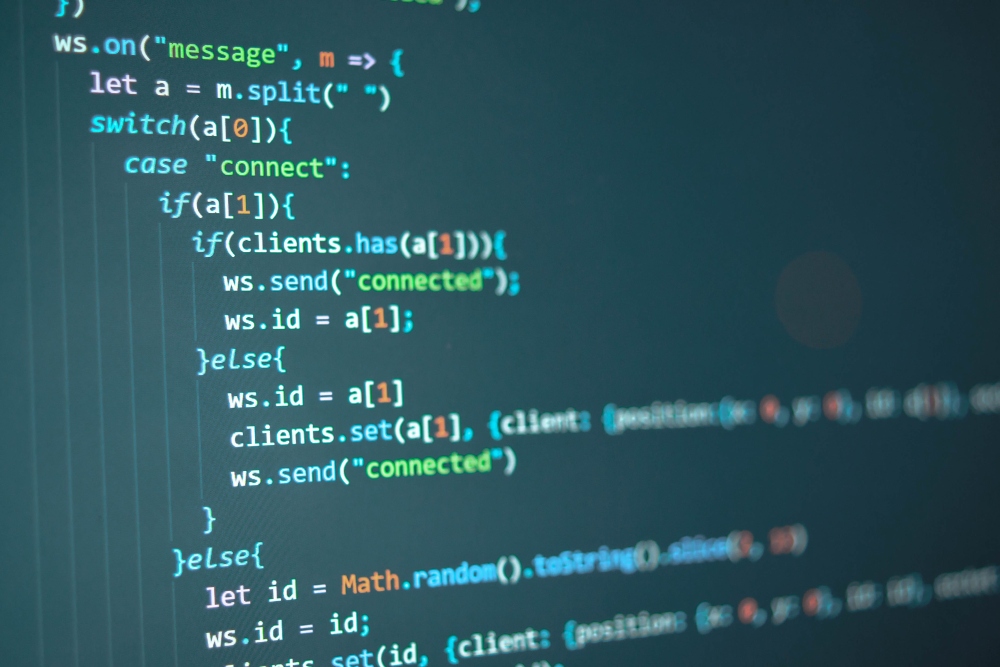
Introduction
Real-time communication plays a crucial role in today’s connected world, powering applications such as IoT devices, web-based chat, gaming, and industrial automation. Choosing the right protocol to facilitate this communication is essential to ensure the smooth functioning and optimal performance of an application. MQTT and SignalR are two popular messaging protocols that serve different purposes and excel in different scenarios.
Understanding MQTT
MQTT Protocol Features
MQTT, short for Message Queuing Telemetry Transport, is a lightweight, publish-subscribe messaging protocol designed for low-bandwidth, high-latency networks. It works over TCP/IP and supports various Quality of Service (QoS) levels, ranging from at-most-once to exactly-once message delivery. Some of the key features of the MQTT protocol include:
- Low overhead: MQTT uses a compact binary format for messages, keeping the protocol overhead minimal and reducing bandwidth consumption.
- Last Will and Testament (LWT): MQTT enables clients to specify a message to be sent by the broker if the client unexpectedly disconnects, ensuring other clients are informed of the disconnection.
- Retained messages: The broker can store the last message published on a topic, allowing new subscribers to receive the most recent message upon subscription.
- Clean and persistent sessions: MQTT supports both transient and durable connections, providing flexibility for various application scenarios.
MQTT Use Cases
Due to its lightweight nature and support for low-bandwidth networks, MQTT is commonly used in the following scenarios:
- Internet of Things (IoT) applications: MQTT is ideal for connecting various IoT devices that send and receive small amounts of data infrequently and over long periods.
- Industrial automation: In environments where low-bandwidth and high-latency networks are common, MQTT excels at providing efficient communication between machines and control systems.
- Remote monitoring and control: MQTT can be employed in applications that require monitoring and control of devices over large geographical distances, such as energy management systems, smart grids, and agriculture.
MQTT Example
To demonstrate MQTT in action, here’s an example using C# to send and receive messages:
Sending a message with MQTT:
using MQTTnet;
using MQTTnet.Client;
using System.Text;
var factory = new MqttFactory();
var client = factory.CreateMqttClient();
var options = new MqttClientOptionsBuilder()
.WithClientId("MyClientId")
.WithTcpServer("test.mosquitto.org")
.Build();
await client.ConnectAsync(options);
var message = new MqttApplicationMessageBuilder()
.WithTopic("mytopic")
.WithPayload(Encoding.UTF8.GetBytes("Hello World"))
.WithAtLeastOnceQoS()
.Build();
await client.PublishAsync(message);
Receiving a message with MQTT:
using MQTTnet;
using MQTTnet.Client;
using MQTTnet.Client.Options;
using System.Text;
var factory = new MqttFactory();
var client = factory.CreateMqttClient();
var options = new MqttClientOptionsBuilder()
.WithClientId("MyClientId")
.WithTcpServer("test.mosquitto.org")
.Build();
await client.ConnectAsync(options);
client.UseApplicationMessageReceivedHandler(e =>
{
var topic = e.ApplicationMessage.Topic;
var payload = Encoding.UTF8.GetString(e.ApplicationMessage.Payload);
Console.WriteLine($"Received: {payload} on topic: {topic}");
});
await client.SubscribeAsync("mytopic");
Understanding SignalR
SignalR Protocol Features
SignalR is a real-time messaging framework for web applications, enabling bi-directional, real-time communication between server and client. It simplifies the process of implementing real-time functionality in .NET applications and provides an abstraction over different transport protocols. Some key features of SignalR include:
- Automatic transport selection: SignalR automatically chooses the best transport protocol (WebSockets, Server-Sent Events, or long polling) based on the capabilities of the client and server, ensuring optimal performance.
- Connection management: SignalR handles connection management, including connection lifetime events and automatic reconnection, simplifying the development process.
- Group communication: SignalR allows for creating groups of connections, enabling efficient broadcasting of messages to a subset of connected clients.
- High-level abstraction: SignalR offers a high-level API that abstracts away low-level details of real-time communication, making it easier for developers to build real-time applications.
SignalR Use Cases
SignalR is best suited for web-based real-time applications, where low latency and high responsiveness are crucial. Common use cases include:
- Web-based chat applications: SignalR can be employed to create chat systems that provide real-time communication between users.
- Gaming: Multiplayer online games benefit from SignalR’s real-time capabilities, allowing seamless communication between players and game servers.
- Notifications and updates: SignalR is ideal for applications that require instant updates or notifications, such as stock market trackers, news websites, or social media platforms.
SignalR Example
To demonstrate SignalR in action, here’s an example using C# to send and receive messages in a .NET Core application:
Creating a SignalR Hub:
using Microsoft.AspNetCore.SignalR;
public class MyHub : Hub
{
public async Task SendMessage(string message)
{
await Clients.All.SendAsync("ReceiveMessage", message);
}
}
Configuring SignalR in Startup.cs:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.Extensions.DependencyInjection;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSignalR();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHub<MyHub>("/myhub");
});
}
}
Sending a message from a client:
using Microsoft.AspNetCore.SignalR.Client;
var connection = new HubConnectionBuilder()
.WithUrl("https://localhost:5001/myhub")
.Build();
await connection.StartAsync();
await connection.SendAsync("SendMessage", "Hello World");
Receiving a message on a client:
using Microsoft.AspNetCore.SignalR.Client;
var connection = new HubConnectionBuilder()
.WithUrl("https://localhost:5001/myhub")
.Build();
connection.On<string>("ReceiveMessage", message =>
{
Console.WriteLine($"Received: {message}");
});
await connection.StartAsync();
Key Differences Between MQTT and SignalR
Network Environment
One of the most prominent differences between MQTT and SignalR is the type of network environment they are designed for. MQTT is best suited for low-bandwidth, high-latency networks, often found in IoT and industrial automation scenarios. SignalR, on the other hand, is designed for web-based real-time applications where low latency and high responsiveness are critical.
Messaging Model
MQTT uses a publish-subscribe model, where clients send and receive messages through topics managed by a broker. This allows for decoupling between publishers and subscribers, as they do not need to know each other’s identities. SignalR follows a more direct client-server communication model. Clients and the server can directly call methods on each other, providing a more tightly coupled communication pattern.
Scalability and Performance
MQTT is designed to be lightweight and efficient, making it an ideal choice for applications that demand low power consumption and minimal bandwidth usage. SignalR, while offering more features and a higher level of abstraction, can be more resource-intensive compared to MQTT. However, SignalR’s automatic transport selection ensures the best possible performance based on the client’s and server’s capabilities.
Supported Transport Protocols
MQTT operates over TCP/IP, ensuring reliable communication between clients and the broker. This is particularly important for IoT and industrial automation applications, where reliable message delivery is crucial. SignalR supports multiple transport protocols, including WebSockets, Server-Sent Events, and long polling. This flexibility allows SignalR to adapt to different network environments and cater to a broader range of applications.
Choosing the Right Protocol: MQTT or SignalR
Evaluating Your Application’s Requirements
To choose the right protocol between MQTT and SignalR, it is essential to carefully evaluate your application’s requirements. Consider factors such as network environment, data size and frequency, latency and responsiveness, and the desired communication pattern.
Ask yourself the following questions:
- Is your application primarily focused on IoT devices or industrial automation?
- Do you need to support low-bandwidth, high-latency networks?
- Is a publish-subscribe model more suitable for your application?
If the answer to these questions is ‘yes,’ MQTT may be the better choice. On the other hand, consider SignalR if:
- Your application is a web-based real-time system.
- You require low-latency, highly responsive communication.
- A client-server communication model is more suitable for your needs.
Decision Factors to Consider
To help you make an informed decision, take the following factors into account:
- Network environment: MQTT is designed for low-bandwidth, high-latency networks, while SignalR excels in web-based real-time applications.
- Messaging model: MQTT uses a publish-subscribe model that decouples publishers and subscribers, whereas SignalR offers a more tightly coupled client-server communication model.
- Scalability and performance: MQTT is lightweight and efficient, ideal for low power consumption and minimal bandwidth usage. SignalR provides more features and a higher level of abstraction, which can be more resource-intensive.
- Supported transport protocols: MQTT operates over TCP/IP for reliable communication, while SignalR supports multiple transport protocols (WebSockets, Server-Sent Events, and long polling) for greater flexibility.